How to Use Pandas to Check Multiple Columns for a Condition
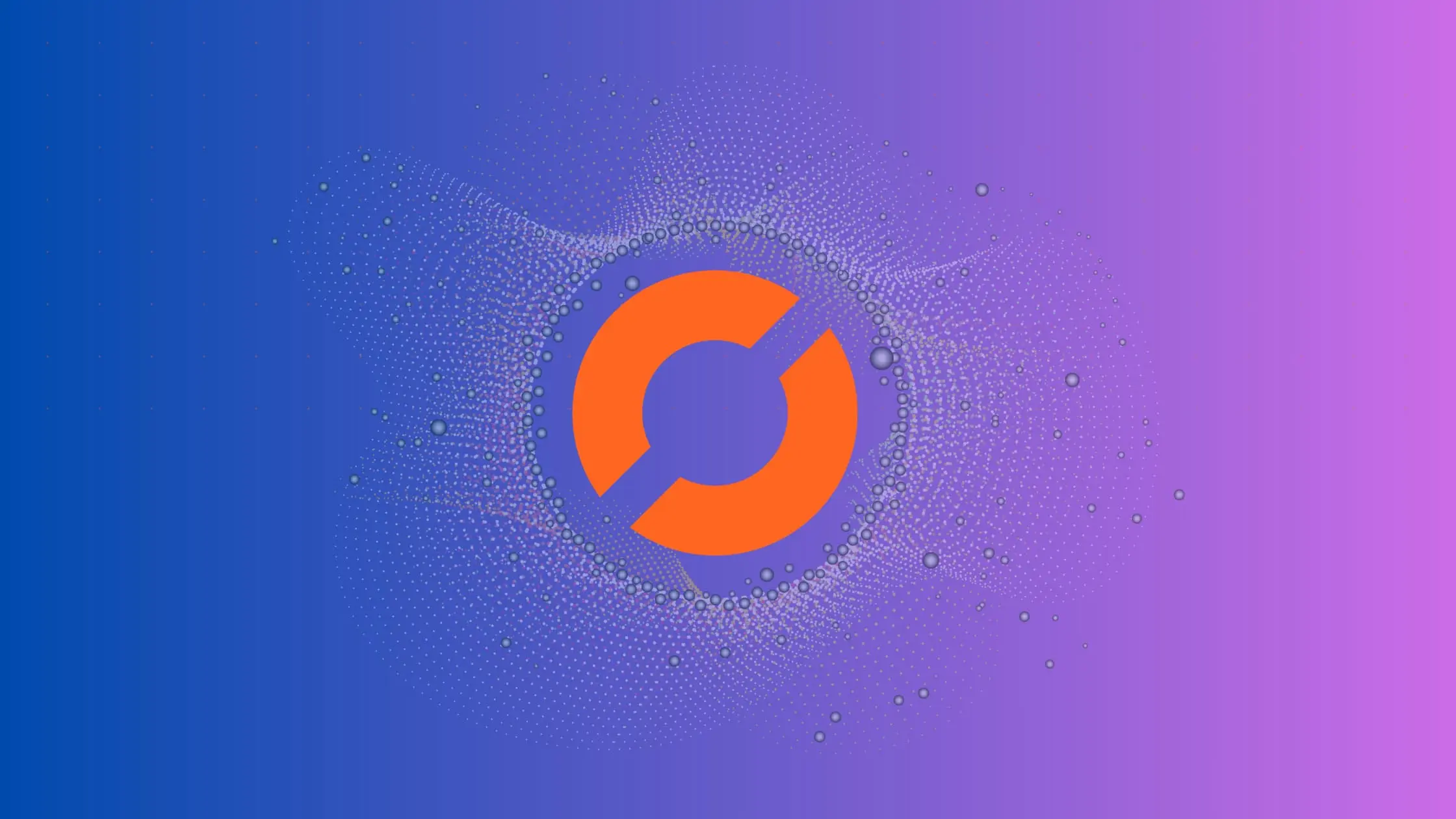
If you are a data scientist or software engineer who works with data on a regular basis, you have likely encountered situations where you need to check multiple columns in a dataframe for a specific condition. Pandas, a popular Python library for data manipulation and analysis, provides several ways to accomplish this task efficiently and effectively.
In this article, we will explore some of the most common techniques for checking multiple columns for a condition using Pandas. We will cover the following topics:
- Using the
loc
method to filter rows based on multiple conditions - Using the
query
method to filter rows based on multiple conditions - Using boolean indexing to filter rows based on multiple conditions
- Using the
apply
method to apply a function to multiple columns
Using the loc Method to Filter Rows Based on Multiple Conditions
The loc
method is a powerful tool for selecting rows and columns from a Pandas dataframe based on specific conditions. To filter rows based on multiple conditions, we can use the &
(and) and |
(or) operators to combine multiple conditions.
For example, let’s say we have a dataframe df
with columns A
, B
, and C
. We want to select all rows where A
is greater than 5 and B
is less than 10. We can accomplish this using the following code:
import pandas as pd
data = {'A' : [6, 3, 7, 2, 8],
'B' : [9, 4, 5, 14, 6]
df = pd.DataFrame(data)
result = df.loc[(df['A'] > 5) & (df['B'] < 10)]
print(result)
Output :
A B
0 6 9
2 7 5
4 8 6
This code creates a boolean mask with two conditions: df['A'] > 5
and df['B'] < 10
. The &
operator combines these conditions into a single boolean mask that selects all rows where both conditions are true.
Using the query Method to Filter Rows Based on Multiple Conditions
The query
method is a convenient way to filter rows from a dataframe based on a string containing one or more conditions. To filter rows based on multiple conditions, we can use the and
and or
keywords to combine multiple conditions.
Using the same example as before, we can accomplish the same result using the following code:
result = df.query('A > 5 and B < 10')
print(result)
This code creates a string with two conditions separated by the and
keyword. The query
method evaluates this string as a boolean mask and selects all rows where both conditions are true.
Using Boolean Indexing to Filter Rows Based on Multiple Conditions
Boolean indexing is a simple and efficient way to filter rows from a dataframe based on a boolean mask. To filter rows based on multiple conditions, we can create a boolean mask with the &
and |
operators, and use it to select the desired rows.
Using the same example as before, we can accomplish the same result using the following code:
mask = (df['A'] > 5) & (df['B'] < 10)
result = df[mask]
print(result)
This code creates a boolean mask with two conditions, and uses it to select all rows where both conditions are true.
Using the apply Method to Apply a Function to Multiple Columns
The apply
method is a versatile tool for applying a function to one or more columns in a dataframe. To apply a function to multiple columns, we can use the apply
method with the axis
parameter set to 1
to apply the function row-wise.
For example, let’s say we have a dataframe df
with columns A
, B
, and C
. We want to create a new column D
that contains the sum of A
and B
for each row. We can accomplish this using the following code:
df['D'] = df.apply(lambda row: row['A'] + row['B'], axis=1)
print(df)
Output :
A B D
0 6 9 15
1 3 4 7
2 7 5 12
3 2 14 16
4 8 6 14
This code applies a lambda function to each row of the dataframe, which calculates the sum of A
and B
and assigns it to a new column D
.
Conclusion
In this article, we have explored several techniques for checking multiple columns for a condition using Pandas. We have shown how to use the loc
and query
methods to filter rows based on multiple conditions, how to use boolean indexing to select rows based on a boolean mask, and how to use the apply
method to apply a function to multiple columns.
By mastering these techniques, you can become more efficient and effective at working with data in Pandas, and become a more valuable data scientist or software engineer.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.