How to Use Laravel Queue with Amazon SQS
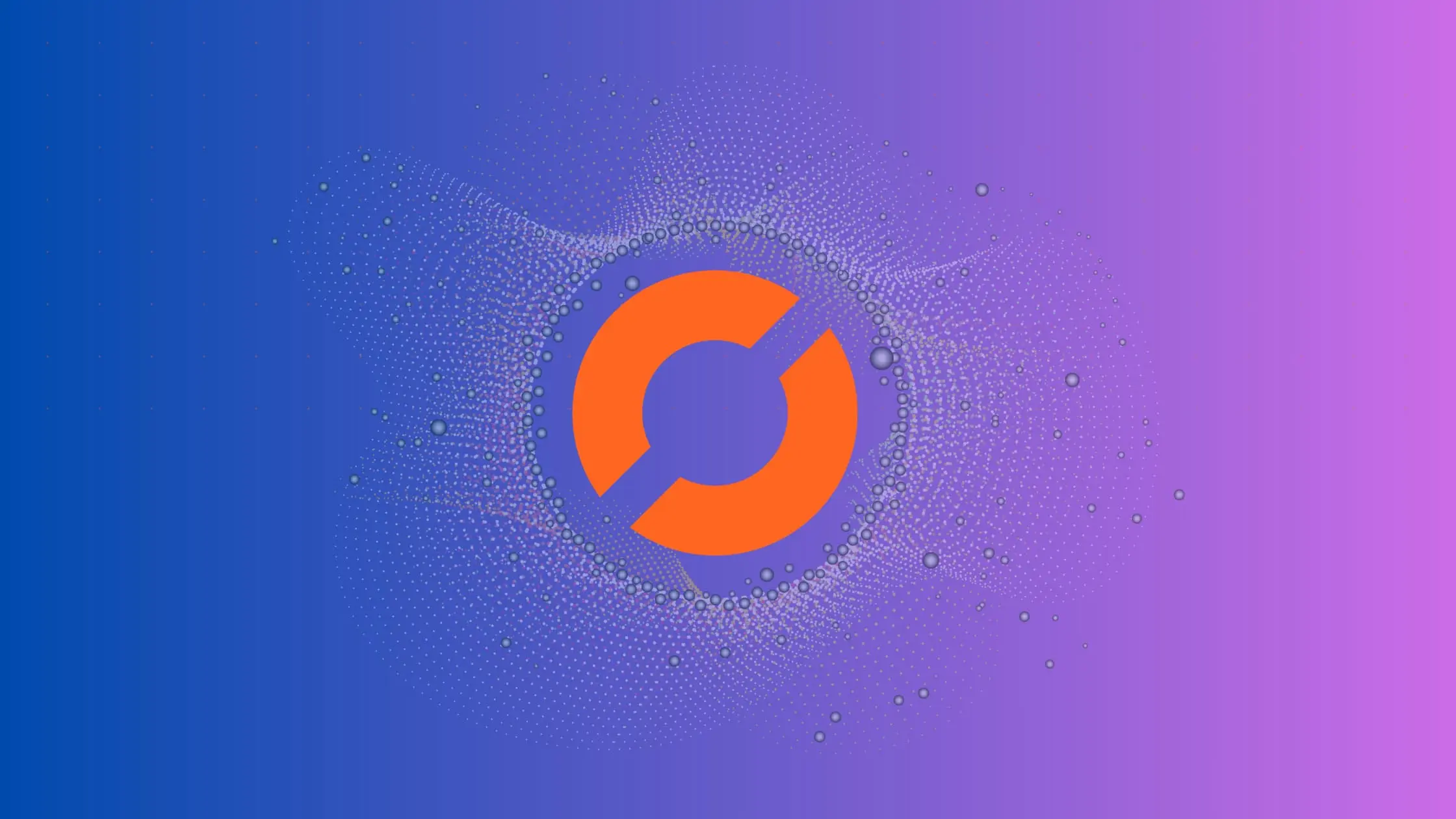
As a data scientist or software engineer, you might have come across the need to process tasks asynchronously in your Laravel application. The Laravel queue system provides an easy way to run tasks in the background and make your application more responsive. However, if you have a high volume of tasks to process, you might need a queue service that can scale horizontally. Amazon Simple Queue Service (SQS) is a fully managed message queuing service that can help you achieve this goal.
In this article, we will show you how to use Laravel Queue with Amazon SQS. We will cover the following topics:
- Setting up Laravel Queue with Amazon SQS
- Creating and dispatching jobs
- Consuming jobs with Amazon SQS
Table of Contents
- Setting up Laravel Queue with Amazon SQS
- Creating and Dispatching Jobs
- Consuming Jobs with Amazon SQS
- Conclusion
Setting up Laravel Queue with Amazon SQS
Before we can start using Amazon SQS as our queue service, we need to configure our Laravel application to use it. Here are the steps to follow:
- Create an AWS account and sign in to the AWS Management Console.
- Go to the IAM dashboard and create a new IAM user with programmatic access.
- Attach the AmazonSQSFullAccess policy to the IAM user.
- Take note of the Access key ID and Secret access key for the IAM user.
- Install the AWS SDK for PHP using Composer:
composer require aws/aws-sdk-php
- Open the
.env
file in your Laravel application and add the following lines:
QUEUE_CONNECTION=sqs
AWS_ACCESS_KEY_ID=your-access-key-id
AWS_SECRET_ACCESS_KEY=your-secret-access-key
AWS_DEFAULT_REGION=your-region
AWS_SQS_QUEUE_URL=your-queue-url
Replace your-access-key-id
, your-secret-access-key
, your-region
, and your-queue-url
with your AWS IAM user credentials and SQS queue URL.
That’s it! Now your Laravel application is configured to use Amazon SQS as the queue service.
Creating and Dispatching Jobs
In Laravel, a job is a unit of work that can be executed asynchronously by the queue worker. Let’s create a simple job that sends an email using the Laravel built-in Mail
facade. Here’s what the job class looks like:
<?php
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use Illuminate\Support\Facades\Mail;
class SendEmail implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
public $email;
public function __construct($email)
{
$this->email = $email;
}
public function handle()
{
Mail::to($this->email)->send(new \App\Mail\Welcome());
}
}
This job class uses the ShouldQueue
interface, which tells Laravel to run the job asynchronously. The handle
method contains the logic of sending an email using the Mail
facade.
To dispatch this job, we can use the dispatch
method of the Bus
facade:
use App\Jobs\SendEmail;
SendEmail::dispatch('john@example.com');
This will add a new job to the Amazon SQS queue.
Consuming Jobs with Amazon SQS
Now that we have dispatched a job, we need to consume it using the queue worker. Laravel provides a built-in queue worker that can be started using the queue:work
Artisan command:
php artisan queue:work --queue=your-queue-name --tries=3
Replace your-queue-name
with the name of your SQS queue.
The --tries
option specifies the number of times the job should be attempted before it is marked as failed. If the job fails, it will be retried according to the retry_after
option in the job class.
That’s it! Now you can process tasks asynchronously in your Laravel application using Amazon SQS as the queue service.
Conclusion
In this article, we have shown you how to use Laravel Queue with Amazon SQS. We have covered the steps to configure Laravel to use Amazon SQS as the queue service, how to create and dispatch jobs, and how to consume jobs using the queue worker.
Using Amazon SQS as the queue service can help you scale your Laravel application horizontally and process tasks asynchronously with ease.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.