How to Use a Dictionary to Replace Column Values on Given Index Numbers on a Pandas Dataframe
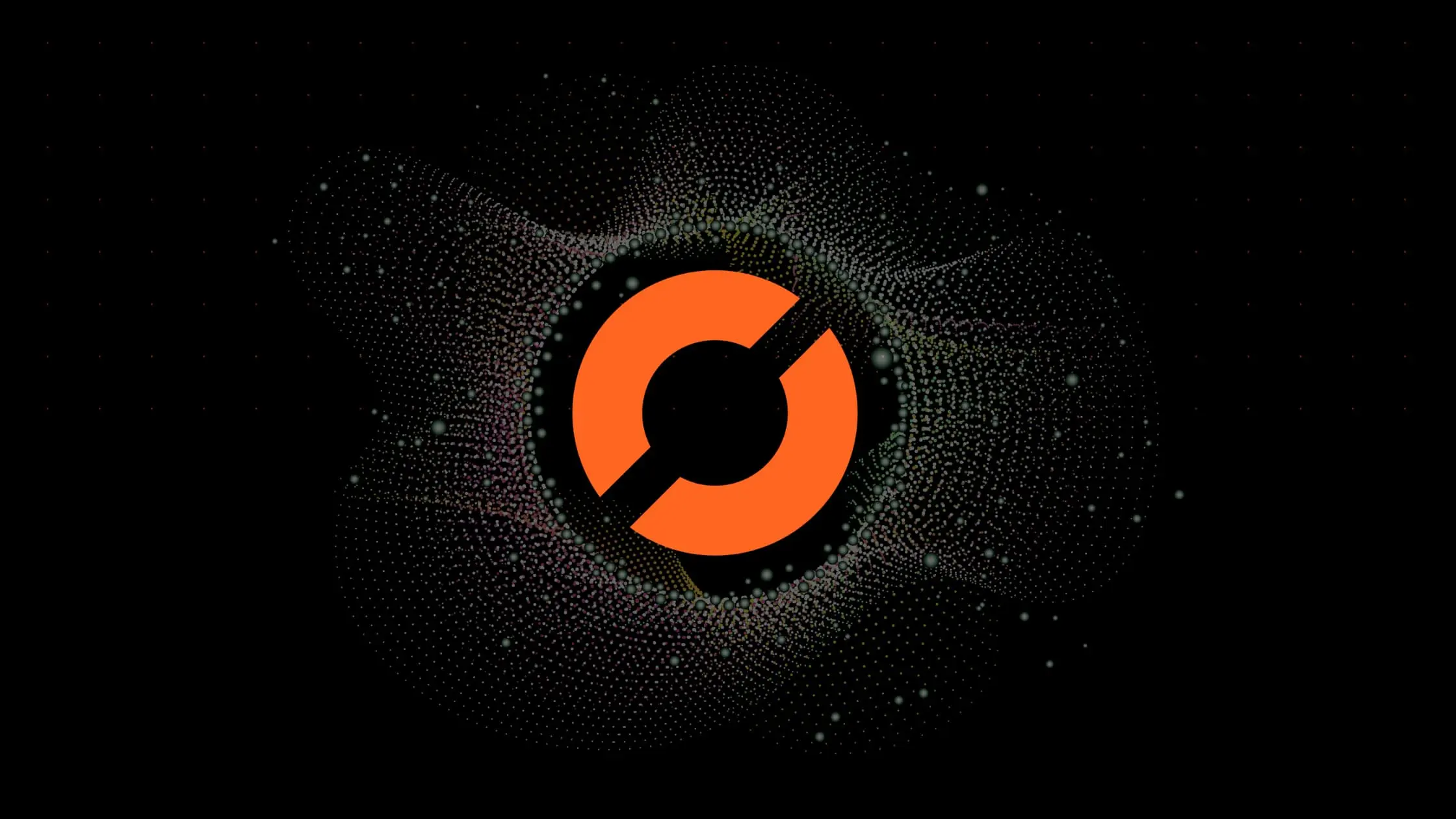
As a data scientist or software engineer, it is often necessary to manipulate data within a pandas dataframe. One common task is to replace specific values in a column with new values based on their index numbers. This can be accomplished easily using a dictionary in Python.
In this tutorial, we will explain how to use a dictionary to replace column values on given index numbers on a pandas dataframe. We will first provide some background on dictionaries and pandas dataframes, and then provide a step-by-step guide on how to implement this technique.
Table of Contents
Background
Dictionaries
A dictionary is an unordered collection of key-value pairs. Each key-value pair is separated by a colon, and the pairs are separated by commas. Dictionaries are used to store data in a way that is easy to access and modify.
To create a dictionary in Python, we use curly braces {}. For example, the following code creates a dictionary with three key-value pairs:
my_dict = {'apple': 1, 'banana': 2, 'orange': 3}
Pandas Dataframes
A pandas dataframe is a two-dimensional table-like data structure that is used to store and manipulate data. It is similar to a spreadsheet in Excel, with rows and columns.
To create a pandas dataframe in Python, we use the pandas.DataFrame()
function. For example, the following code creates a dataframe with three columns and three rows:
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35], 'gender': ['F', 'M', 'M']}
df = pd.DataFrame(data)
Step-by-Step Guide
Now that we have covered the background on dictionaries and pandas dataframes, we will provide a step-by-step guide on how to use a dictionary to replace column values on given index numbers on a pandas dataframe.
Step 1: Create a Dictionary of Replacement Values
The first step is to create a dictionary of replacement values. The keys in the dictionary should be the index numbers of the values you want to replace, and the values should be the new values you want to replace them with.
For example, suppose we have the following dataframe:
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35], 'gender': ['F', 'M', 'M']}
df = pd.DataFrame(data)
print(df)
Output:
name age gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 M
We want to replace the age of Bob (index 1) with 40. To do this, we create a dictionary with the key-value pair {1: 40}
:
replacement_dict = {1: 40}
Step 2: Use the Dictionary to Replace Values in the Dataframe
The second step is to use the dictionary to replace the values in the dataframe. We can do this using the update()
method in pandas.
The update()
method takes a Series version of the dictionary of replacement values as input as shown below:
df['age'].update(pd.Series(replacement_dict))
After running this code, the age of Bob in the dataframe will be updated to 40.
Step 3: Verify the Results
The final step is to verify that the replacement was successful. We can do this by printing the dataframe and checking that the value has been updated.
print(df)
The output should be:
name age gender
0 Alice 25 F
1 Bob 40 M
2 Charlie 35 M
Common Errors and How to Handle Them
Error 1: KeyError - Index Not Found in DataFrame: If an index specified in the dictionary is not present in the DataFrame, a KeyError will occur. To handle this, verify the existence of each index in the dictionary before replacement.
for index in replacement_dict.keys():
if index not in df.index:
print(f"Index {index} not found in DataFrame.")
# Handle or remove the index from the dictionary
Error 2: ValueError - Incompatible Data Types: Mismatched data types between the DataFrame and dictionary values can result in a ValueError. Ensure data type consistency for seamless replacement.
for index, value in replacement_dict.items():
if not pd.api.types.is_numeric_dtype(df['Column1'].dtype):
print(f"Data type mismatch at index {index}.")
# Convert the value to the appropriate data type
Error 3: TypeError - Mismatched Data Structures: If the structure of the DataFrame and dictionary don’t align, a TypeError may occur. Ensure that the column names match and that the dictionary is appropriately structured.
if set(replacement_dict.keys()) != set(df.index):
print("Indices in the dictionary do not match DataFrame indices.")
# Adjust the dictionary to match DataFrame structure
Error 4: IndexError - Invalid Index Number: If an invalid index number is used, an IndexError will be raised. Validate the index numbers before replacement.
for index in replacement_dict.keys():
if not 0 <= index < len(df):
print(f"Invalid index {index}.")
# Adjust the index or remove it from the dictionary
Error 5: Missing Values in the Dictionary: If a specified index in the DataFrame lacks a corresponding value in the dictionary, a ValueError may occur. Ensure that all replacement indices have corresponding values.
for index in df.index:
if index not in replacement_dict:
print(f"Missing value for index {index}.")
# Provide a default value or handle the missing value
Conclusion
In this tutorial, we have explained how to use a dictionary to replace column values on given index numbers on a pandas dataframe. This technique is useful for manipulating data within a dataframe, and can be used in a variety of contexts.
By following the step-by-step guide provided in this tutorial, you should now be able to use a dictionary to replace column values on given index numbers on a pandas dataframe in your own work.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.