How to Update Column Values in Pandas Based on Criteria From Another Column
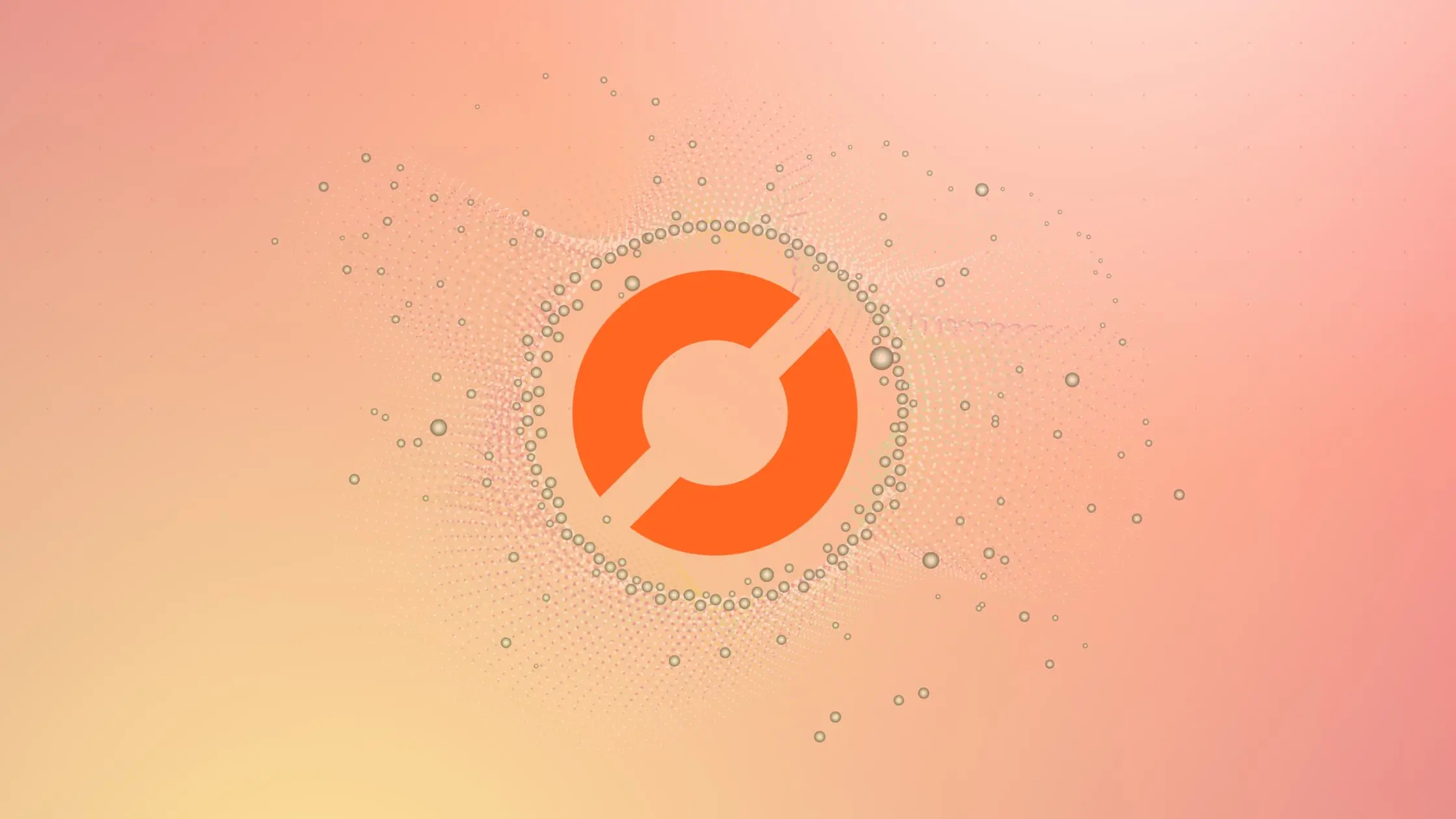
As a data scientist or software engineer, you may often come across a situation where you need to update certain column values in a Pandas DataFrame based on certain criteria from another column. This can be done using conditional statements and the apply
function in Pandas. In this article, we will discuss how to update column values in Pandas based on criteria from another column, with step-by-step instructions and code examples.
Table of Contents
- What is Pandas?
- Updating Column Values Based on Criteria
- Updating Multiple Columns Based on Criteria
- Common Errors and Solutions
- Conclusion
What is Pandas?
Pandas is an open-source data analysis and manipulation library for Python. It provides data structures for efficiently storing and manipulating large datasets, as well as tools for data cleaning, transformation, and analysis. Pandas is widely used in data science and machine learning for tasks such as data preprocessing, exploratory data analysis, and feature engineering.
Updating Column Values Based on Criteria
Suppose you have a Pandas DataFrame with two columns, column1
and column2
. You want to update the values in column2
based on certain criteria from column1
. For example, you may want to update the values in column2
to be the same as the values in column1
if the values in column1
meet a certain condition.
To do this, you can use the apply
function in Pandas along with a lambda function that checks the condition and returns the updated value. Here’s an example code snippet that updates the values in column2
to be the same as the values in column1
if the values in column1
are greater than 10:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'column1': [5, 10, 15, 20], 'column2': [0, 0, 0, 0]})
# update column2 based on criteria from column1
df['column2'] = df.apply(lambda row: row['column1'] if row['column1'] > 10 else row['column2'], axis=1)
print(df)
Output:
column1 column2
0 5 0
1 10 0
2 15 15
3 20 20
In this example, we first create a sample DataFrame with two columns, column1
and column2
, and initialize the values in column2
to 0. We then update the values in column2
based on the condition that the values in column1
are greater than 10. We do this by applying a lambda function to each row of the DataFrame using the apply
function. The lambda function checks if the value in column1
is greater than 10, and if so, returns the value in column1
. Otherwise, it returns the value in column2
. We specify axis=1
to apply the function to each row of the DataFrame.
Updating Multiple Columns Based on Criteria
In some cases, you may need to update multiple columns in a Pandas DataFrame based on criteria from another column. You can do this by modifying the lambda function to return a tuple of updated values, and then assigning the tuple to the corresponding columns.
Here’s an example code snippet that updates the values in column2
and column3
to be the same as the values in column1
if the values in column1
are greater than 10:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'column1': [5, 10, 15, 20], 'column2': [0, 0, 0, 0], 'column3': [0, 0, 0, 0]})
# update column2 and column3 based on criteria from column1
df[['column2', 'column3']] = df.apply(lambda row: (row['column1'], row['column1']) if row['column1'] > 10 else (row['column2'], row['column3']), axis=1).tolist()
print(df)
Output:
column1 column2 column3
0 5 0 0
1 10 0 0
2 15 15 15
3 20 20 20
In this example, we first create a sample DataFrame with three columns, column1
, column2
, and column3
, and initialize the values in column2
and column3
to 0. We then update the values in column2
and column3
based on the condition that the values in column1
are greater than 10. We do this by applying a lambda function to each row of the DataFrame using the apply
function. The lambda function checks if the value in column1
is greater than 10, and if so, returns a list of the value in column1
for both column2
and column3
. Otherwise, it returns a list of the values in column2
and column3
. We assign the resulting list to the corresponding columns using the double bracket notation ([['column2', 'column3']]
).
Common Errors and Solutions
Error 1: Incorrect Axis Specification
When using the apply
function, specifying the axis incorrectly can lead to unexpected results or errors. Ensure that axis=1
is used when applying the function row-wise.
# Incorrect usage
df['column2'] = df.apply(lambda row: row['column1'] if row['column1'] > 10 else row['column2'], axis=0).tolist()
# Correct usage
df['column2'] = df.apply(lambda row: row['column1'] if row['column1'] > 10 else row['column2'], axis=1).tolist()
Error 2: Mismatched DataFrame Shapes
Error: Assigning a single value to multiple columns without matching the DataFrame shapes.
Solution: Make sure the shapes match when updating multiple columns; use tuples or lists accordingly.
Conclusion
In this article, we discussed how to update column values in Pandas based on criteria from another column. We showed how to use the apply
function along with a lambda function to update a single column, and how to modify the lambda function to update multiple columns. By following these steps, you can easily update column values in Pandas based on any criteria from another column.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.