How to Update a Cell Value in Pandas DataFrame
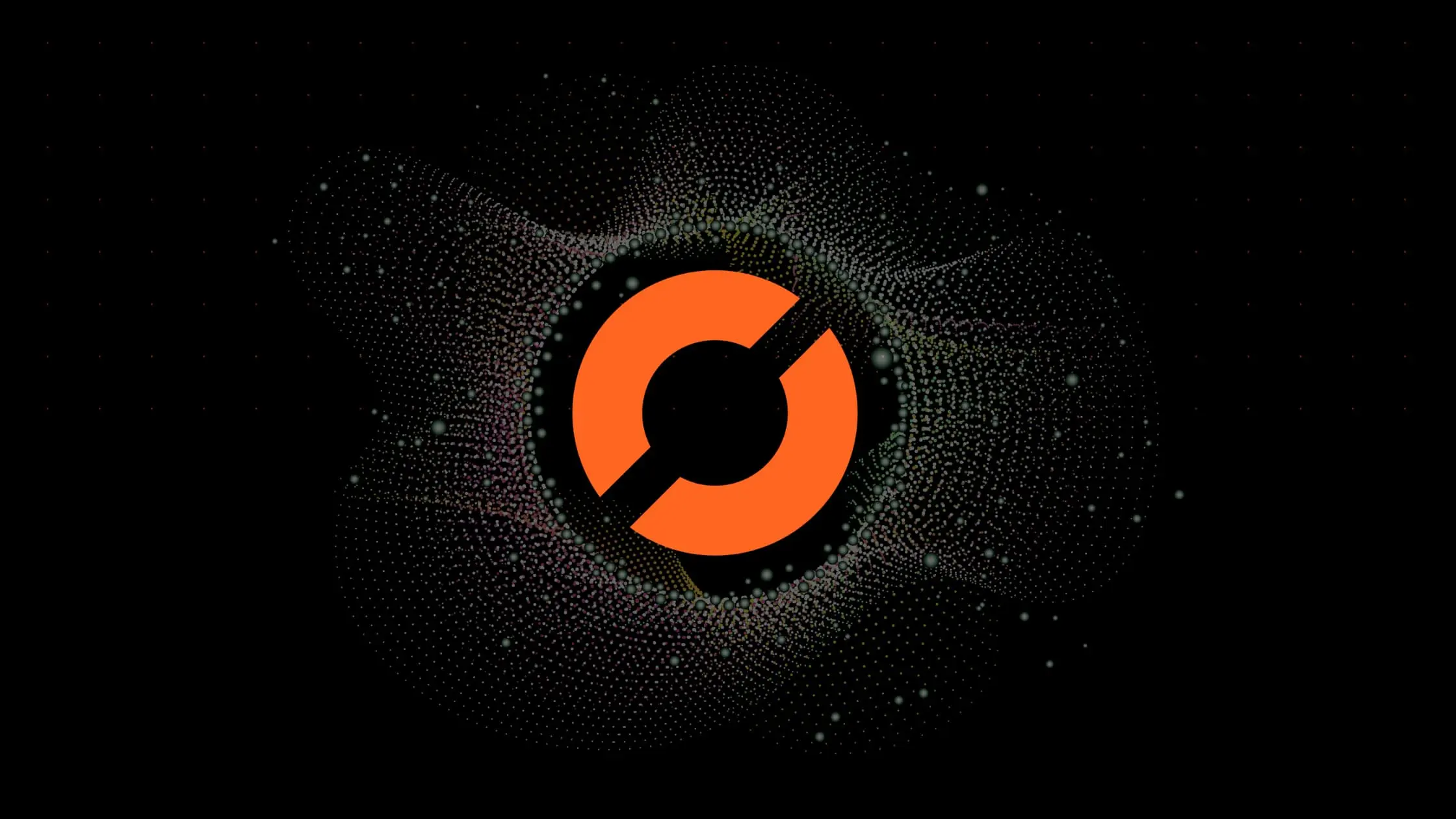
As a data scientist or software engineer, you’ll likely spend a lot of time working with data in Python using the Pandas library. One essential task you’ll need to perform is updating the values in a Pandas DataFrame. In this article, we’ll explore how to update a cell value in a Pandas DataFrame, including the different ways to update a single cell and multiple cells at once.
What Is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table, but with more powerful features. Pandas makes it easy to manipulate and analyze data in Python, from cleaning and preprocessing to analysis and visualization.
How to Update a Single Cell Value
To update a single cell value in a Pandas DataFrame, you can use the .at
or .iat
accessor methods. The .at
method uses the label of the row and column to update the cell value, while the .iat
method uses the integer position.
Let’s assume we have the following DataFrame:
import pandas as pd
# Create a dataframe
data = {'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'gender': ['F', 'M', 'M']}
df = pd.DataFrame(data)
print(df)
Output:
name age gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 M
To update the cell value of the first row and age column to 26, we can use the .at
method as follows:
df.at[0, 'age'] = 26
print(df)
Output:
name age gender
0 Alice 26 F
1 Bob 30 M
2 Charlie 35 M
Alternatively, we can use the .iat
method to update the same cell value:
df.iat[0, 1] = 26
print(df)
Output:
name age gender
0 Alice 26 F
1 Bob 30 M
2 Charlie 35 M
Both methods achieve the same result, but the .at
method is more flexible since it allows us to use labels instead of integer positions.
How to Update Multiple Cell Values
To update multiple cell values in a Pandas DataFrame, we can use boolean indexing or the .loc
accessor method. Boolean indexing allows us to select a subset of the DataFrame based on a condition and update the selected cells. The .loc
method, on the other hand, allows us to select a subset of the DataFrame using labels or boolean masks and update the selected cells.
Let’s assume we have the following DataFrame:
# Create a dataframe
data = {'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'gender': ['F', 'M', 'M']}
df = pd.DataFrame(data)
print(df)
Output:
name age gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 M
To update the age of all males to 40, we can use boolean indexing as follows:
df.loc[df['gender'] == 'M', 'age'] = 40
print(df)
Output:
name age gender
0 Alice 25 F
1 Bob 40 M
2 Charlie 40 M
Alternatively, we can use the .loc
method to achieve the same result:
df.loc[df['gender'] == 'M', ['age']] = 40
print(df)
Output:
name age gender
0 Alice 25 F
1 Bob 40 M
2 Charlie 40 M
Both methods achieve the same result, but the .loc
method is more flexible since it allows us to select multiple columns at once.
Conclusion
In this article, we explored how to update a cell value in a Pandas DataFrame. We learned that we can update a single cell using either the .at
or .iat
accessor methods, and update multiple cells using boolean indexing or the .loc
accessor method. By mastering these techniques, you’ll be able to manipulate and analyze data in Pandas with ease.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.