How to Suppress Scientific Notation in Pandas
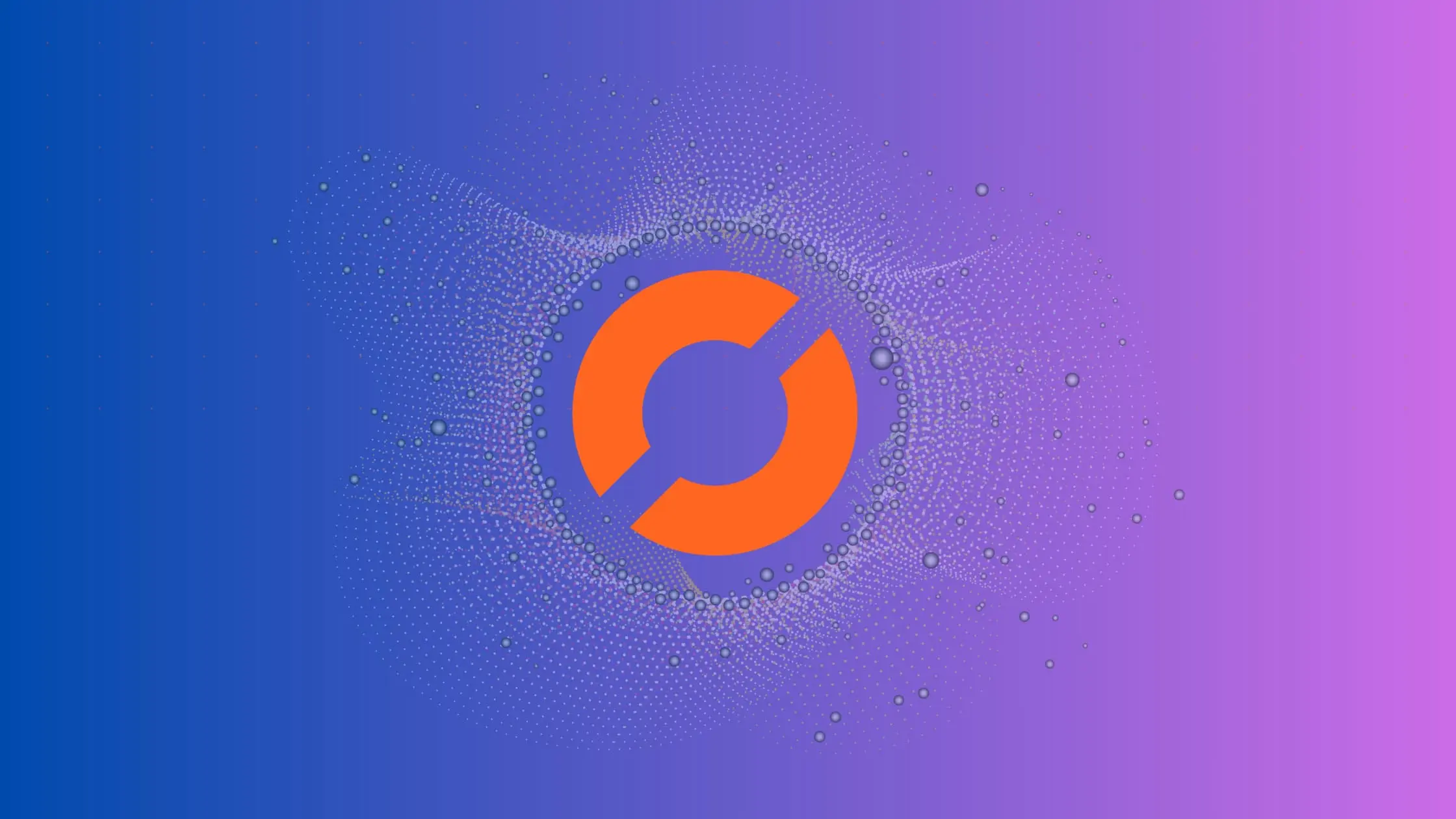
How to Suppress Scientific Notation in Pandas
If you’ve ever worked with large datasets in Pandas, you may have noticed that the numbers in your dataframes are sometimes displayed in scientific notation. While this is a useful feature for displaying very large or very small numbers, it can be frustrating when you want to work with the data in its original form. Fortunately, there’s a simple way to suppress scientific notation in Pandas.
What is Scientific Notation?
Scientific notation is a way of representing numbers that are either very large or very small. It uses a base number (usually 10) multiplied by a power of 10 to represent the original number. For example, the number 10,000,000 can be represented in scientific notation as 1 x 10^7. Similarly, the number 0.00000001 can be represented as 1 x 10^-8.
Why does Pandas use Scientific Notation?
Pandas uses scientific notation to display large or small numbers in a more compact and readable format. When working with datasets that contain a wide range of values, scientific notation can make it easier to compare and analyze the data. However, if you want to work with the data in its original form, you may want to suppress scientific notation.
How to Suppress Scientific Notation in Pandas
To suppress scientific notation in Pandas, you can use the float_format
method. This method allows you to specify a formatting string that will be used to display floating point numbers in your dataframe. By setting the precision and width of the formatting string, you can control how the numbers are displayed.
Here’s an example of how to use the float_format
method to suppress scientific notation:
import pandas as pd
# create a dataframe with a column of large numbers
df = pd.DataFrame({'large_numbers': [10000000000.0, 20000000000, 30000000000]})
# display the dataframe with scientific notation
print(df)
# suppress scientific notation by setting float_format
pd.options.display.float_format = '{:.0f}'.format
# display the dataframe without scientific notation
print(df)
In this example, we first create a dataframe with a column of large numbers. When we display the dataframe without using float_format
, we can see that the numbers are displayed in scientific notation. However, when we set pd.options.display.float_format = '{:.0f}'.format
, we suppress the scientific notation and display the numbers in their original form.
You can adjust the formatting string to control the precision and width of the displayed numbers. For example, if you want to display floating point numbers with two decimal places, you can set pd.options.display.float_format = '{:.2f}'.format
.
Conclusion
Scientific notation is a useful feature for displaying large or small numbers in a compact and readable format. However, if you want to work with the data in its original form, you may want to suppress scientific notation. Pandas provides a simple way to do this using the float_format
method. By setting a formatting string that controls the precision and width of the displayed numbers, you can customize the way your data is displayed in Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.