How to Strip White Space from Pandas DataFrames
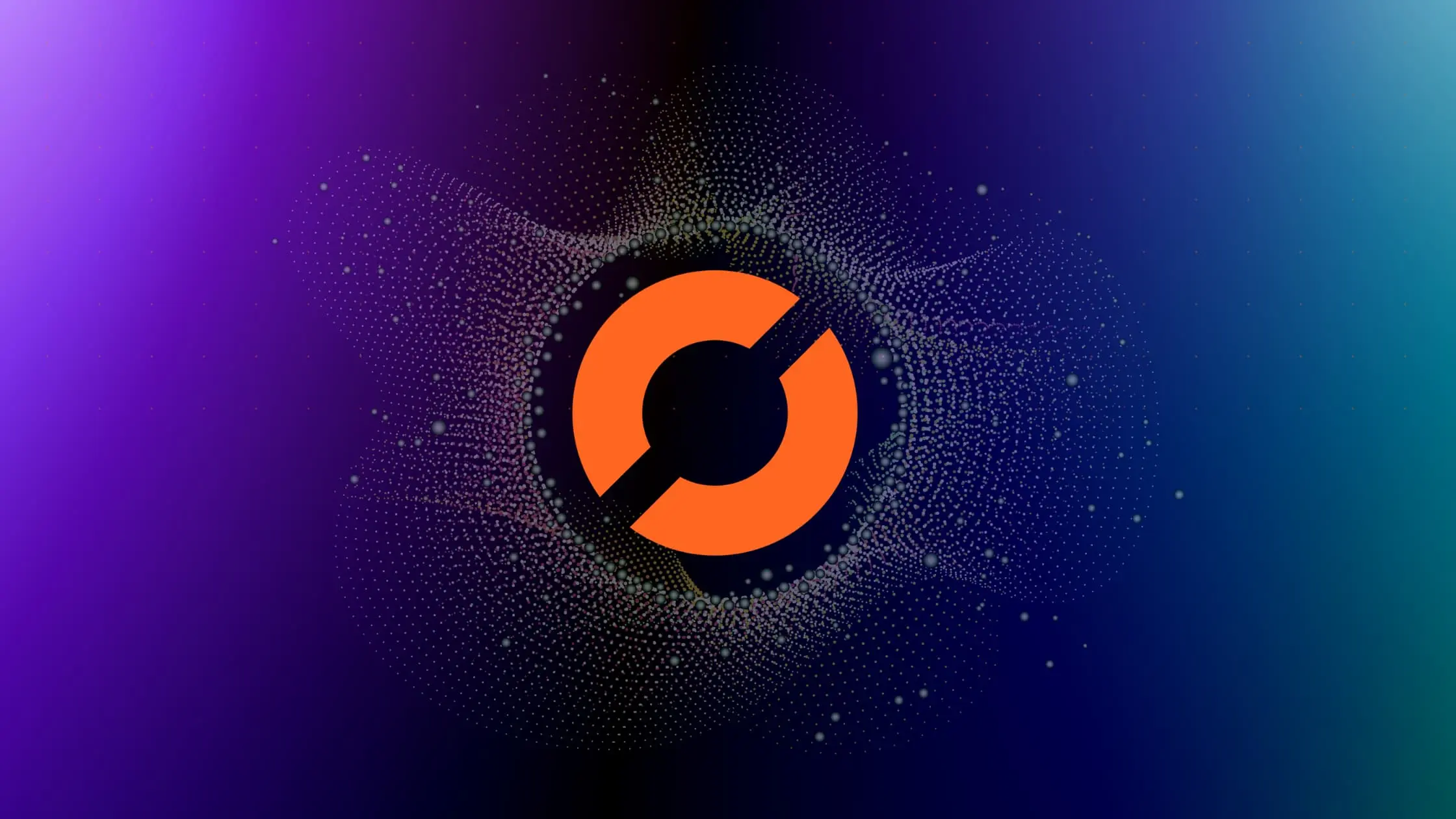
As a data scientist or software engineer, you are likely familiar with Pandas, a powerful Python library used for data manipulation and analysis. One common data cleaning task is to remove leading and trailing white space from strings in a DataFrame. In this article, we will explore several methods for stripping white space from Pandas DataFrames.
Why Strip White Space?
Before we dive into the techniques for stripping white space, let’s first discuss why it’s important. White space refers to any blank space in a string, including spaces, tabs, and line breaks. In some cases, white space is intentionally included in data, such as when formatting text. However, in other cases, white space can be accidentally introduced when data is entered or imported, leading to inconsistencies and errors in analysis. By removing white space, we can ensure that our data is clean and consistent, making it easier to analyze and draw insights from.
Method 1: Using the strip() Method
The most straightforward way to remove white space from strings in a Pandas DataFrame is to use the strip()
method. This method removes leading and trailing white space from a string, but leaves any white space within the string intact. To apply this method to a DataFrame, we can use the .applymap()
method to apply the strip()
method element-wise to all strings in the DataFrame.
import pandas as pd
# create example DataFrame
df = pd.DataFrame({'A': [' apple', 'banana ', ' orange '], 'B': [' cat ', ' dog', 'bird ']})
print(df)
Output:
A B
0 apple cat
1 banana dog
2 orange bird
# apply strip() method to all strings in DataFrame
df = df.applymap(lambda x: x.strip() if isinstance(x, str) else x)
print(df)
Output:
A B
0 apple cat
1 banana dog
2 orange bird
In this example, we create a DataFrame with two columns, 'A'
and 'B'
, each containing strings with leading and trailing white space. We then apply the strip()
method to all strings in the DataFrame using a lambda function and the .applymap()
method. The resulting DataFrame has all white space removed from the strings.
Method 2: Using the str.strip() Method
Another way to remove white space from strings in a Pandas DataFrame is to use the str.strip()
method. This method is similar to the strip()
method, but is applied directly to a string using the str
accessor. To apply this method to all strings in a DataFrame, we can use the .apply()
method to apply the str.strip()
method to each element in a column.
import pandas as pd
# create example DataFrame
df = pd.DataFrame({'A': [' apple', 'banana ', ' orange '], 'B': [' cat ', ' dog', 'bird ']})
# apply str.strip() method to all strings in column 'A'
df['A'] = df['A'].apply(lambda x: x.strip() if isinstance(x, str) else x)
print(df)
Output:
A B
0 apple cat
1 banana dog
2 orange bird
In this example, we create a DataFrame with two columns, 'A'
and 'B'
, each containing strings with leading and trailing white space. We then apply the str.strip()
method to all strings in column 'A'
using the .apply()
method and a lambda function. The resulting DataFrame has all white space removed from the strings in column 'A'
.
Method 3: Using Regular Expressions
A more powerful way to remove white space from strings in a Pandas DataFrame is to use regular expressions. Regular expressions are a sequence of characters that define a search pattern. They can be used to match and manipulate text in a variety of ways, including removing white space. To use regular expressions to remove white space from a DataFrame, we can use the replace()
method with a regular expression pattern that matches white space.
import pandas as pd
# create example DataFrame
df = pd.DataFrame({'A': [' apple', 'banana ', ' orange '], 'B': [' cat ', ' dog', 'bird ']})
# apply regular expression to remove white space from all strings in DataFrame
df = df.replace(r'\s+', '', regex=True)
print(df)
Output:
A B
0 apple cat
1 banana dog
2 orange bird
In this example, we create a DataFrame with two columns, 'A'
and 'B'
, each containing strings with leading and trailing white space. We then use the replace()
method with a regular expression pattern that matches one or more white space characters (\s+
) and replaces them with a single space character. The resulting DataFrame has all white space removed from the strings.
Conclusion
In this article, we explored several methods for stripping white space from Pandas DataFrames. By removing white space, we can ensure that our data is clean and consistent, making it easier to analyze and draw insights from. Whether you prefer the simplicity of the strip()
method, the flexibility of the str.strip()
method, or the power of regular expressions, Pandas provides several ways to remove white space from your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.