How to Start Index at 1 for Pandas DataFrame
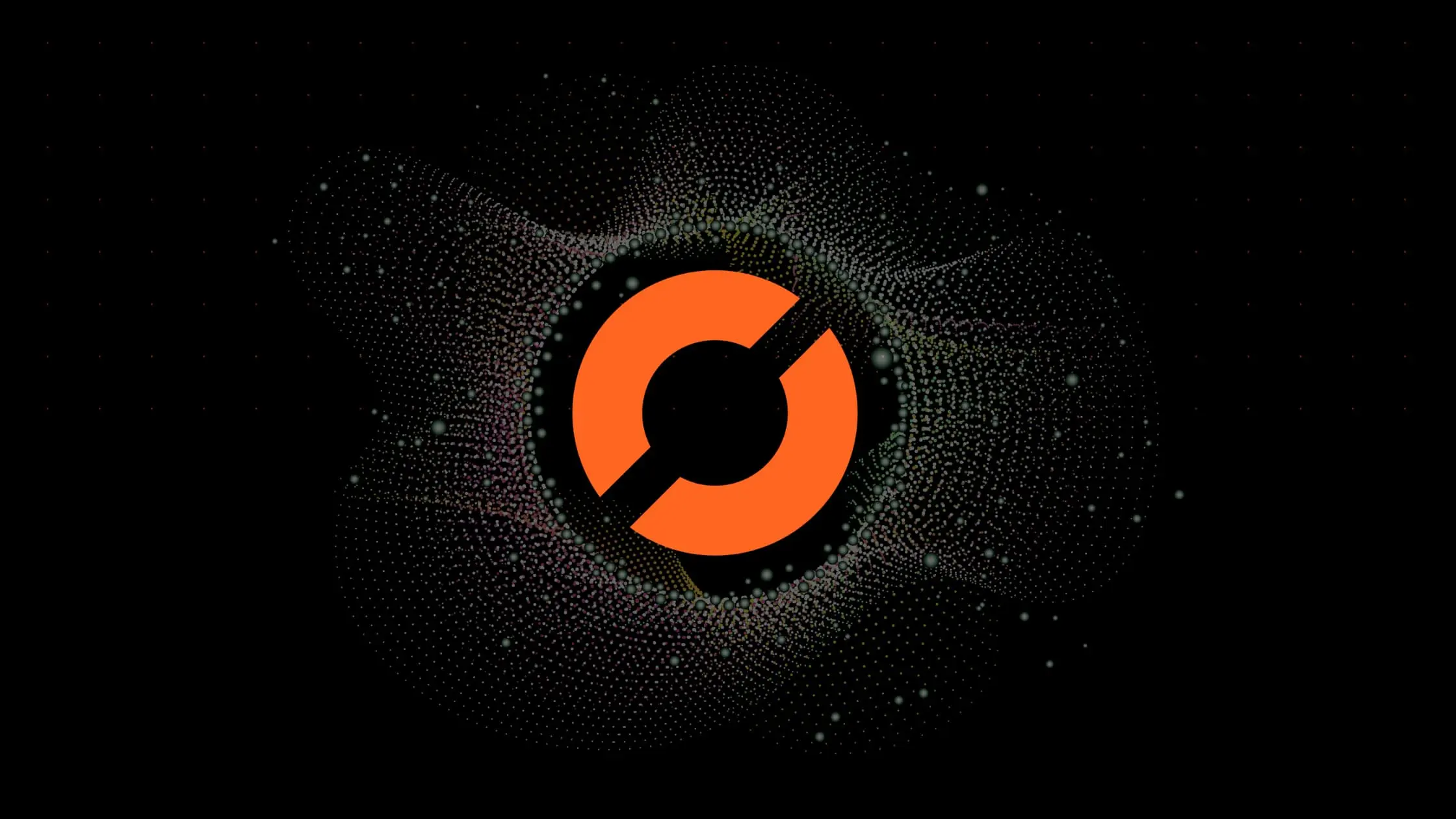
As a data scientist or software engineer, you may have encountered a situation where you need to start the index of a Pandas DataFrame at 1 instead of 0. This can be useful for various reasons, such as aligning data with external sources or improving readability for non-technical users. In this article, we will discuss the different ways to accomplish this task.
Table of Contents
- Introduction
- Method 1: Reset Index
- Method 2: Set Index Directly
- Method 3: Create a Custom Index
- Conclusion
Method 1: Reset Index
The simplest method to start the index at 1 is to reset the index of the DataFrame and add 1 to each value. Here’s an example:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# reset the index and add 1 to each value
df.index = df.index + 1
print(df)
Output:
A B
1 1 4
2 2 5
3 3 6
In this example, we first create a DataFrame with two columns and three rows. We then reset the index using the reset_index()
method, which moves the existing index to a new column called “index”. Finally, we add 1 to each value in the “index” column using the +
operator.
Method 2: Set Index Directly
Another way to start the index at 1 is to set the index directly using the index
attribute of the DataFrame:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# set the index directly to a range starting from 1
df.index = range(1, len(df)+1)
print(df)
Output:
A B
1 1 4
2 2 5
3 3 6
In this example, we use the range()
function to create a range of values starting from 1 and ending at the length of the DataFrame plus 1. We then assign this range to the index
attribute of the DataFrame.
This method has the advantage of not creating a new column for the original index, but it requires more code than the previous method.
Method 3: Create a Custom Index
If you want more control over the index values, you can create a custom index using the Index
class:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# create a custom index starting from 1
index = pd.Index(range(1, len(df)+1))
# assign the custom index to the DataFrame
df.index = index
print(df)
Output:
A B
1 1 4
2 2 5
3 3 6
In this example, we first create a range of values starting from 1 and ending at the length of the DataFrame plus 1 using the range()
function. We then create a custom index using the Index
class and assign it to a variable called index
. Finally, we assign the custom index to the DataFrame using the index
attribute.
This method is useful if you need to create a non-sequential index or if you want to use a different data type for the index values.
Conclusion
Starting the index at 1 for a Pandas DataFrame can be useful for various reasons, and there are several ways to accomplish this task. In this article, we discussed three methods: resetting the index and adding 1 to each value, setting the index directly to a range starting from 1, and creating a custom index using the Index
class. Depending on your specific use case, you may prefer one method over the others.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.