How to Split Pandas Dataframe Column Values in Python
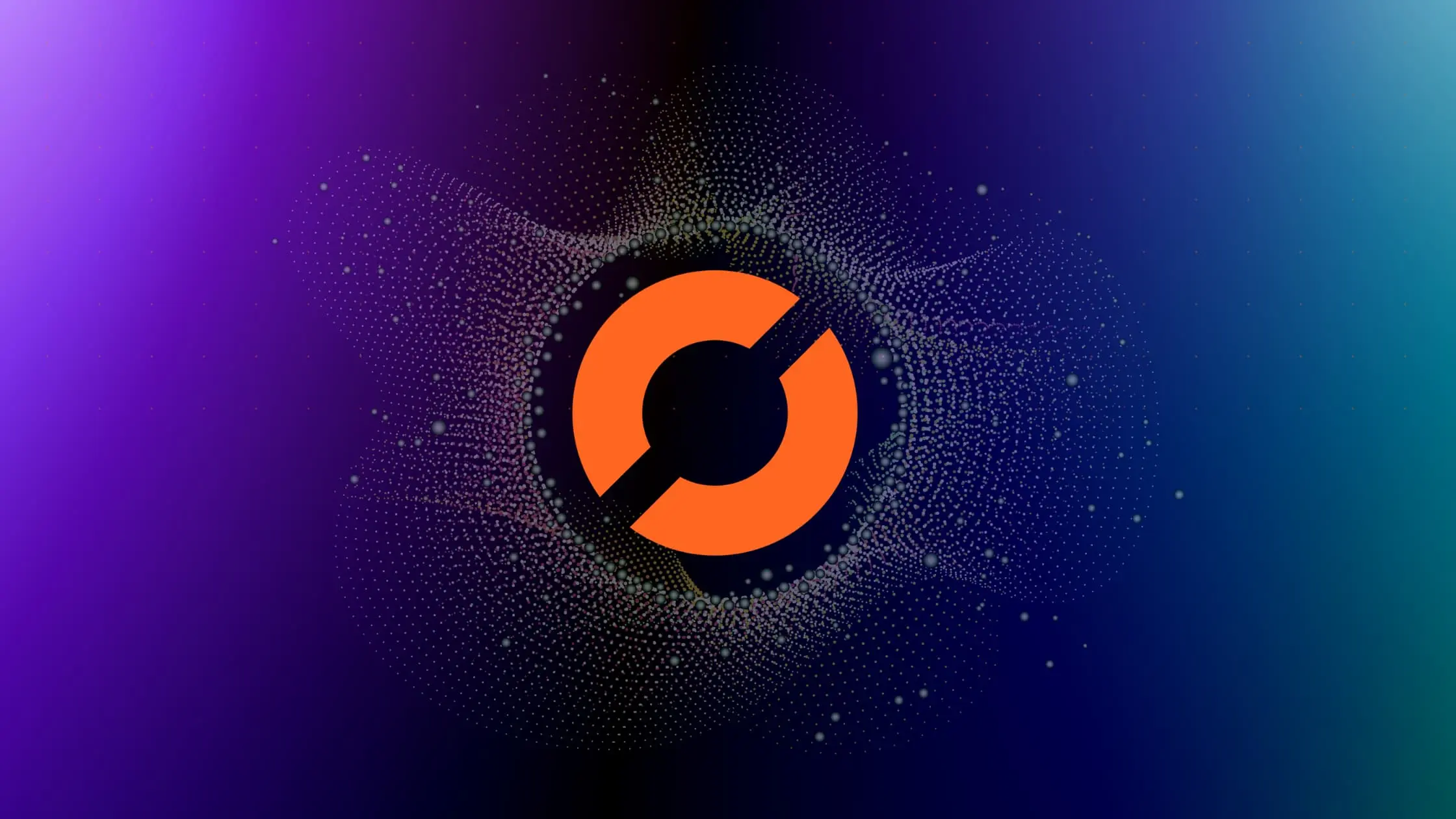
How to Split Pandas Dataframe Column Values in Python
As a data scientist or software engineer, you may come across a situation where you need to split the values in a Pandas dataframe column. This could be to extract specific information from the column or to create additional columns based on the split values. In this article, we will explore how to split Pandas dataframe column values in Python.
What is Pandas?
Pandas is a popular open-source data analysis library for Python. It provides easy-to-use data structures and data analysis tools for handling and manipulating data. Pandas dataframes are two-dimensional tables with rows and columns, similar to spreadsheets or SQL tables.
Understanding Pandas Dataframe Column Values
Before we dive into splitting column values, let’s first understand how Pandas dataframe column values are represented.
Pandas dataframe columns can contain different types of data such as text, numbers, and dates. Each column can have a specific data type, such as string, integer, float, or datetime. The data type determines how the column values are stored and how operations can be performed on the column.
For example, a column with string values can be manipulated using string methods such as split(), strip(), and replace(). A column with numerical values can be manipulated using mathematical operations such as addition, subtraction, and multiplication.
Splitting Pandas Dataframe Column Values
Splitting Pandas dataframe column values can be done using the split() method. The split() method splits a string into a list of strings based on a specified separator. The separator can be a single character, a string, or a regular expression.
Let’s take an example dataframe with a column named “Name” containing full names of individuals:
import pandas as pd
data = {'Name': ['John Smith', 'Jane Doe', 'Bob Johnson']}
df = pd.DataFrame(data)
print(df)
Output:
Name
0 John Smith
1 Jane Doe
2 Bob Johnson
Now, let’s split the “Name” column into two columns: “First Name” and “Last Name”.
df[['First Name', 'Last Name']] = df['Name'].str.split(' ', expand=True)
print(df)
Output:
Name First Name Last Name
0 John Smith John Smith
1 Jane Doe Jane Doe
2 Bob Johnson Bob Johnson
In the above example, we used the str.split() method to split the “Name” column into two columns using the space character as the separator. We then used the expand=True parameter to create two new columns with the split values.
Splitting Pandas Dataframe Column Values into Multiple Columns
Sometimes, you may need to split a column into multiple columns based on multiple separators. For example, you may have a column containing addresses in the format “Street, City, State, Zip”. You may want to split this column into four columns: “Street”, “City”, “State”, and “Zip”.
Let’s take an example dataframe with a column named “Address” containing addresses:
data = {'Address': ['123 Main St, Anytown, CA, 12345', '456 1st Ave, Anycity, NY, 67890']}
df = pd.DataFrame(data)
print(df)
Output:
Address
0 123 Main St, Anytown, CA, 12345
1 456 1st Ave, Anycity, NY, 67890
Now, let’s split the “Address” column into four columns: “Street”, “City”, “State”, and “Zip”.
df[['Street', 'City', 'State', 'Zip']] = df['Address'].str.split(', ', expand=True)
print(df)
Output:
Address Street City State Zip
0 123 Main St, Anytown, CA, 12345 123 Main St Anytown CA 12345
1 456 1st Ave, Anycity, NY, 67890 456 1st Ave Anycity NY 67890
In the above example, we used the str.split() method to split the “Address” column into four columns using the “, " separator. We then used the expand=True parameter to create four new columns with the split values.
Conclusion
In this article, we explored how to split Pandas dataframe column values in Python. We learned how to split a column into two columns using a single separator and how to split a column into multiple columns using multiple separators. The split() method is a powerful tool for manipulating Pandas dataframe columns and can be used to extract specific information or create new columns based on split values.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.