How to Split a Column into Multiple Columns in PySpark Without Using Pandas
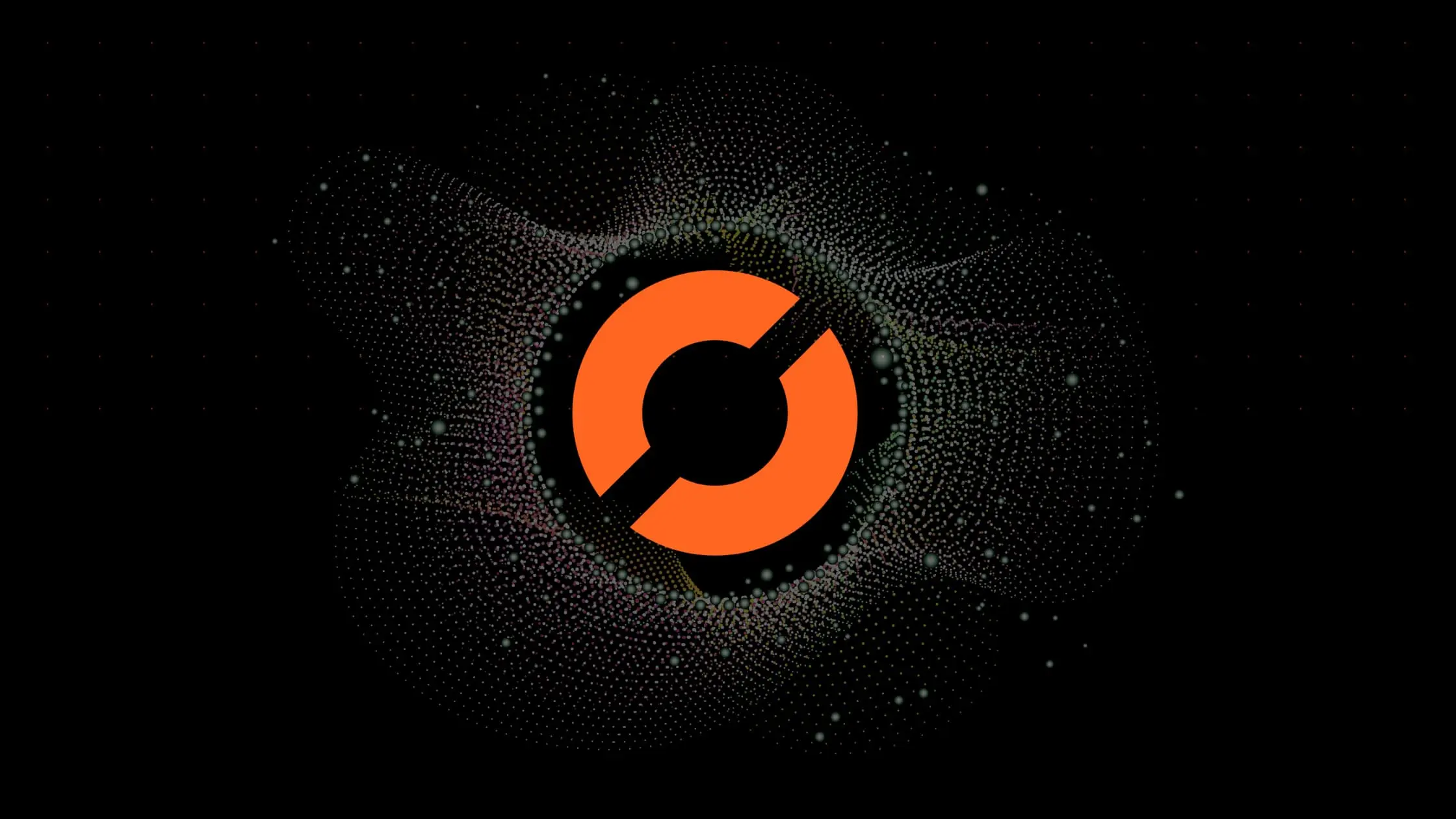
In data science, working with large datasets is a common occurrence. When working with big data, it’s essential to use tools that can handle the volume of data and process it efficiently. PySpark is a powerful tool for data processing and analysis, and it’s commonly used in big data applications.
One common task in data processing is splitting a column into multiple columns. In this blog post, we’ll explore how to split a column into multiple columns in PySpark without using Pandas.
Table of Contents
What is PySpark?
PySpark is the Python API for Apache Spark, an open-source distributed computing system that can process large datasets quickly. PySpark is a powerful tool for data processing and analysis, and it’s commonly used in big data applications.
PySpark provides an easy-to-use programming interface for Spark, allowing programmers to write Spark applications in Python. PySpark can handle large datasets in a distributed computing environment, making it an ideal tool for big data processing.
Why Use PySpark?
PySpark offers several advantages over traditional data processing tools. Some of the key benefits of using PySpark include:
Speed: PySpark can process large datasets significantly faster than traditional data processing tools.
Scalability: PySpark can handle large datasets and scale to meet the needs of big data applications.
Ease of use: PySpark provides an easy-to-use programming interface for Spark, making it accessible to programmers with Python experience.
Flexibility: PySpark can be used for a wide range of data processing tasks, including data cleaning, transformation, and analysis.
Now that we’ve covered what PySpark is and why it’s useful let’s dive into how to split a column into multiple columns without using Pandas.
The Problem
Suppose we have a PySpark DataFrame that contains a column with comma-separated values. We want to split the column into multiple columns based on the comma delimiter. First, let’s create a DataFrame.
# Importing necessary modules from PySpark
from pyspark.sql import SparkSession
from pyspark.sql.functions import split
# Creating a Spark session with the application name "split-column"
spark = SparkSession.builder.appName("split-column").getOrCreate()
# Sample data with columns "id" and "values"
data = [(1, "1,2,3"), (2, "4,5,6"), (3, "7,8,9")]
# Defining column names for the DataFrame
columns = ["id", "values"]
# Creating a PySpark DataFrame from the provided data and columns
df = spark.createDataFrame(data, columns)
# Displaying the DataFrame
df.show()
Here’s an example of what the DataFrame might look like:
+----+---------------+
| id | values |
+----+---------------+
| 1 | 1,2,3 |
| 2 | 4,5,6 |
| 3 | 7,8,9 |
+----+---------------+
Our goal is to split the values
column into three columns, like this:
+----+---+---+---+
| id | 1 | 2 | 3 |
+----+---+---+---+
| 1 | 1 | 2 | 3 |
| 2 | 4 | 5 | 6 |
| 3 | 7 | 8 | 9 |
+----+---+---+---+
The Solution
To split a column into multiple columns in PySpark without using Pandas, we can use the built-in split
function available in PySpark’s functions
module.
Here’s the PySpark code to accomplish this:
from pyspark.sql.functions import split
# Split the values column based on the comma delimiter
split_col = split(df['values'], ',')
# Add the split columns to the DataFrame
df = df.withColumn('col1', split_col.getItem(0))
df = df.withColumn('col2', split_col.getItem(1))
df = df.withColumn('col3', split_col.getItem(2))
# Drop the original values column
df = df.drop('values')
Let’s break down what’s happening in this code:
First, we import the
split
function from PySpark’sfunctions
module.We use the
split
function to split thevalues
column into separate columns based on the comma delimiter. The result is aColumn
object that contains an array of values.We use the
getItem
function to extract the individual values from the array and add them to the DataFrame as separate columns.Finally, we drop the original
values
column from the DataFrame.
And that’s it! We’ve successfully split a column into multiple columns in PySpark without using Pandas.
Exploring Common Errors
Error 1: Incorrect Delimiter
One common mistake is using the wrong delimiter. Ensure that the delimiter used in the split
function matches the actual delimiter in your data.
Error 2: Handling Null Values
If your DataFrame contains null values, be cautious when applying the split operation. Handle nulls appropriately to avoid unexpected behavior.
Error 3: Inconsistent Number of Values
If the number of values in the ‘values’ column is inconsistent across rows, the split operation may result in errors. Address this by either ensuring consistency or handling variations appropriately.
Conclusion
In this blog post, we’ve explored how to split a column into multiple columns in PySpark without using Pandas. PySpark is a powerful tool for data processing and analysis, and it’s commonly used in big data applications.
By using PySpark’s built-in split
function, we can split a column into multiple columns quickly and efficiently. This technique is useful when working with large datasets and can help streamline data processing tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.