How to Specify Data Type in Pandas CSV Reader
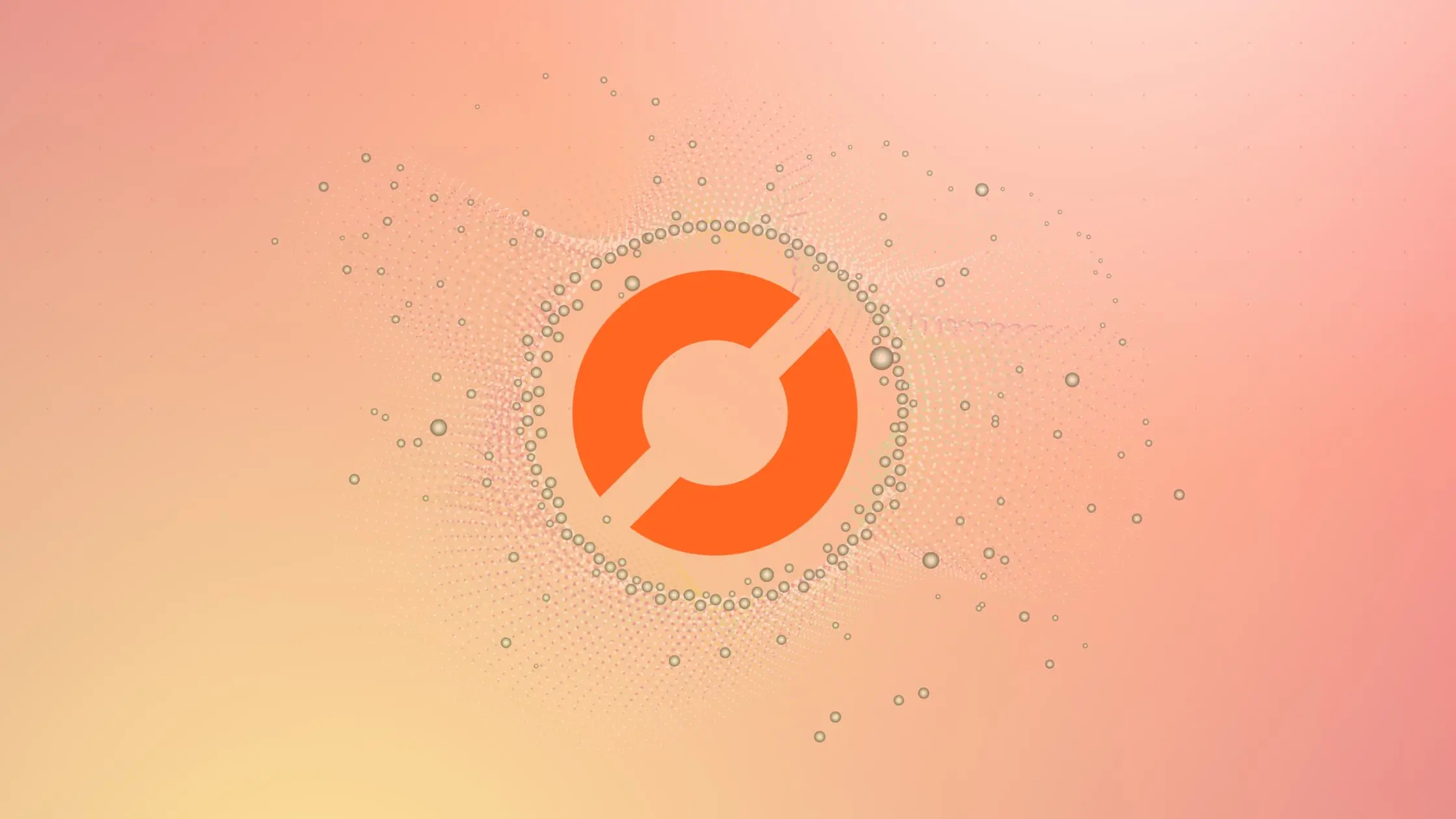
How to Specify Data Type in Pandas CSV Reader
As a data scientist, you frequently work with large datasets in various formats. CSV (Comma Separated Values) is one of the most common formats for storing and exchanging data. Often, you need to specify the data type of the columns in the CSV file to ensure that the data is correctly interpreted and processed by your analysis.
In this article, we will explore how to specify data types in the Pandas CSV reader. We will cover the following topics:
- What is Pandas CSV reader?
- Why is it important to specify data types?
- How to specify data types in Pandas CSV reader?
- Examples of specifying data types in Pandas CSV reader
What is Pandas CSV reader?
Pandas is a popular Python library for data manipulation and analysis. It provides various data structures and functions to work with tabular data, such as data frames and series. Pandas also supports reading and writing data in various formats, including CSV.
The read_csv()
function in Pandas is used to read CSV files. It returns a data frame object, which is a two-dimensional table with rows and columns. Each column in the data frame has a label, and the rows represent the observations or samples.
Why is it important to specify data types?
When reading a CSV file, Pandas tries to infer the data type of each column automatically. However, this process may not always be accurate, especially when dealing with large datasets with many columns and complex data types.
Specifying the data type of each column in the CSV file helps to ensure that the data is correctly interpreted and processed by your analysis. For example, if a column contains numerical data, but Pandas infers it as a string, arithmetic operations may not work as expected. Similarly, if a column contains dates, but Pandas infers it as text, you may not be able to perform date-specific operations on it.
How to specify data types in Pandas CSV reader?
You can specify the data type of each column in the CSV file using the dtype
parameter in the read_csv()
function. The dtype
parameter takes a dictionary as its value, where the keys are the column names and the values are the data types.
For example, let’s say you have a CSV file data.csv
with the following content:
Name, Age, Height, Weight
John, 25, 180, 75.5
Mary, 28, 165, 58.2
To specify the data type of each column, you can use the following code:
import pandas as pd
data = pd.read_csv('data.csv', dtype={
'Name': 'string',
'Age': 'int64',
'Height': 'float64',
'Weight': 'float64'
})
In this example, we specify the data type of the Name
column as string
, Age
column as int64
, Height
column as float64
, and Weight
column as float64
. Note that we use string
instead of str
for the Name
column because str
is not a valid data type in Pandas.
If a column in the CSV file contains missing or invalid values, you can specify a custom value to replace them using the na_values
parameter. For example, if the Age
column in the data.csv
file contains missing values represented as -1
, you can use the following code:
Examples of specifying data types in Pandas CSV reader
Let’s look at some examples of specifying data types in Pandas CSV reader.
Example 1: Specifying data types for a CSV file with simple data types
Consider a CSV file simple.csv
with the following content:
A, B, C
1, 2, 3
4, 5, 6
7, 8, 9
To specify the data type of each column, you can use the following code:
import pandas as pd
data = pd.read_csv('simple.csv', dtype={
'A': 'int64',
'B': 'int64',
'C': 'int64'
})
In this example, we specify the data type of all columns as int64
.
Example 2: Specifying data types for a CSV file with complex data types
Consider a CSV file complex.csv
with the following content:
Name, Age, Height, Weight, Date
John, 25, 180.0, 75.5, 2022-01-01
Mary, 28, 165.5, 58.2, 2022-02-01
To specify the data type of each column, you can use the following code:
import pandas as pd
data = pd.read_csv('complex.csv', dtype={
'Name': 'string',
'Age': 'int64',
'Height': 'float64',
'Weight': 'float64',
'Date': 'datetime64[ns]'
})
In this example, we specify the data type of the Name
column as string
, Age
column as int64
, Height
column as float64
, Weight
column as float64
, and Date
column as datetime64[ns]
.
Conclusion
In this article, we learned how to specify data types in the Pandas CSV reader. We saw that specifying the data type of each column in the CSV file is important to ensure that the data is correctly interpreted and processed by your analysis. We also saw that we can specify data types using the dtype
parameter and handle missing values using the na_values
parameter. Finally, we saw some examples of specifying data types for CSV files with simple and complex data types.
By following the guidelines presented in this article, you can ensure that your analysis is based on accurate and reliable data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.