How to Sort a Pandas Dataframe by Date
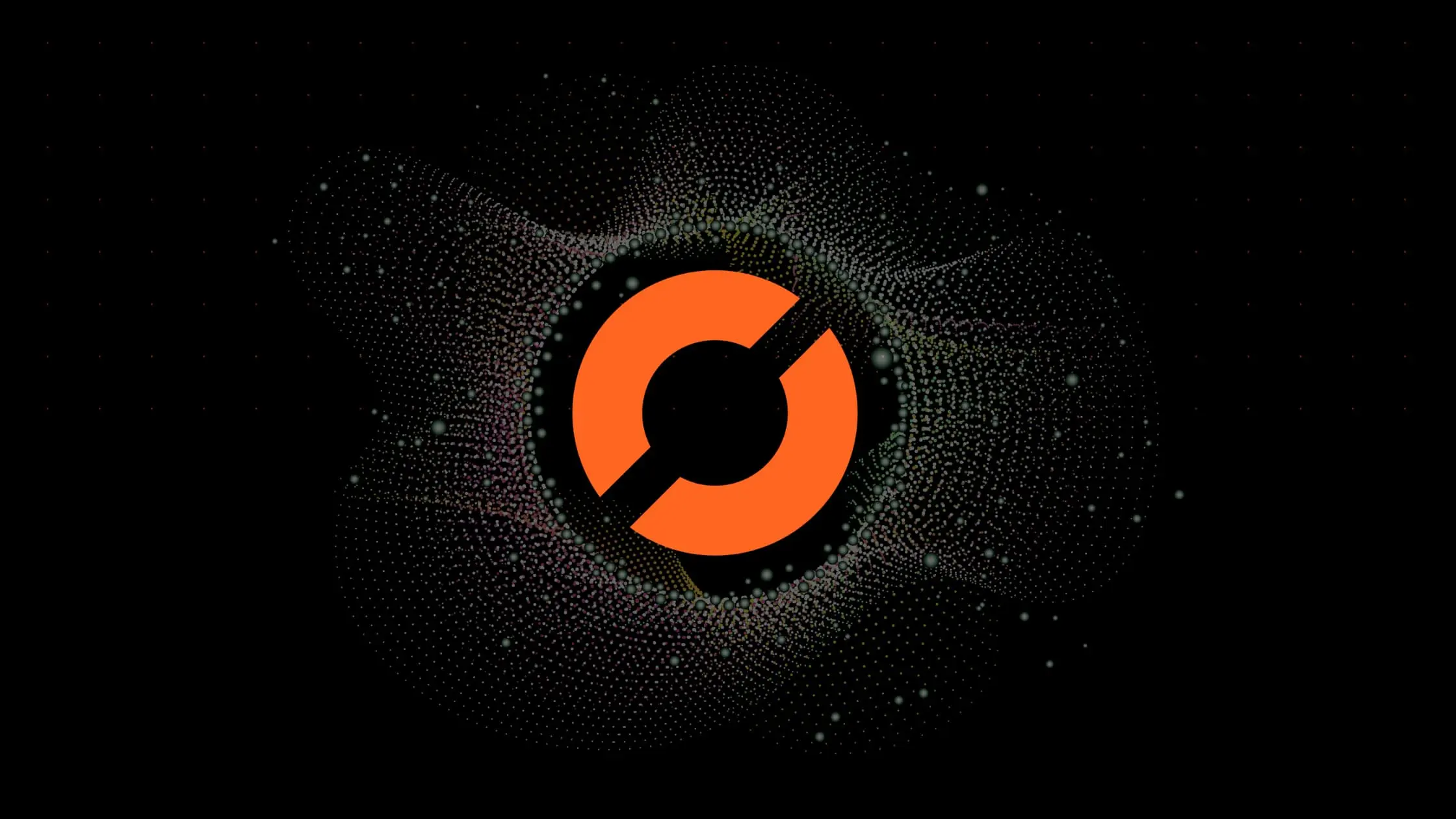
Table of Contents
As a data scientist or software engineer, you may often work with large datasets that contain time-series data. In these cases, sorting the data by date is a critical step in analyzing and visualizing the data. Pandas is a popular data manipulation library in Python that provides powerful tools to work with such data. In this article, we will discuss how to sort a Pandas dataframe by date.
What is a Pandas Dataframe?
A Pandas dataframe is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table, but with more powerful indexing functionality. A dataframe can be created from a variety of data sources, including CSV files, Excel files, and SQL databases.
Sorting a Pandas Dataframe by Date
Sorting a Pandas dataframe by date can be accomplished in several ways, depending on the format of the date column. In general, Pandas can handle dates represented as strings, datetime objects, or Unix timestamps. In this article, we will focus on sorting dataframes with datetime objects.
Step 1: Import the Pandas Library
Before we can work with Pandas, we need to import the library. This can be done with the following code:
import pandas as pd
Step 2: Create a Sample Dataframe
To illustrate how to sort by date, we will create a sample dataframe with two columns: “Date” and “Value”. The “Date” column will contain datetime objects representing dates, and the “Value” column will contain random numbers.
import numpy as np
# create DF
dates = pd.date_range('2022-01-01', periods=10)
values = np.random.rand(10)
df = pd.DataFrame({'Date': dates, 'Value': values})
print(df)
This will create a dataframe with 10 rows and 2 columns:
Date Value
0 2022-01-01 0.339057
1 2022-01-02 0.536268
2 2022-01-03 0.994324
3 2022-01-04 0.221931
4 2022-01-05 0.587187
5 2022-01-06 0.200858
6 2022-01-07 0.534712
7 2022-01-08 0.761072
8 2022-01-09 0.360308
9 2022-01-10 0.282459
Step 3: Sort the Dataframe by Date
To sort the dataframe by date, we can use the sort_values()
method of the dataframe. We need to specify the column to sort by, which in this case is "Date"
. We also need to set the inplace
parameter to True
to modify the original dataframe.
df.sort_values(by=['Date'], inplace=True)
print(df)
This will sort the dataframe by the "Date"
column in ascending order:
Date Value
0 2022-01-01 0.339057
1 2022-01-02 0.536268
2 2022-01-03 0.994324
3 2022-01-04 0.221931
4 2022-01-05 0.587187
5 2022-01-06 0.200858
6 2022-01-07 0.534712
7 2022-01-08 0.761072
8 2022-01-09 0.360308
9 2022-01-10 0.282459
If we want to sort in descending order, we can set the ascending
parameter to False
.
df.sort_values(by=['Date'], inplace=True, ascending=False)
print(df)
This will sort the dataframe by the “Date” column in descending order:
Date Value
9 2022-01-10 0.282459
8 2022-01-09 0.360308
7 2022-01-08 0.761072
6 2022-01-07 0.534712
5 2022-01-06 0.200858
4 2022-01-05 0.587187
3 2022-01-04 0.221931
2 2022-01-03 0.994324
1 2022-01-02 0.536268
0 2022-01-01 0.339057
Handling Common Errors
Mixed Data Types in the Date Column
Example Scenario:
Consider a DataFrame where the date column contains mixed data types.
import pandas as pd
data = {'Date': ['2023-01-15', '2023-01-10', '2023-01-20', 20230125],
'Value': [25, 30, 15, 40]}
df = pd.DataFrame(data)
df.sort_values(by=['Date'], inplace=True, ascending=False)
print(df)
Output:
TypeError: '<' not supported between instances of 'str' and 'int'
Handling the Error:
Convert the entire "Date"
column to datetime format to ensure consistency.
df['Date'] = pd.to_datetime(df['Date'], errors='coerce')
print(df)
Output:
Date Value
0 2023-01-15 25
1 2023-01-10 30
2 2023-01-20 15
3 2023-01-25 40
Incorrect Sorting Order
Suppose you want to sort your DataFrame in descending order by date, but the default is ascending.
import pandas as pd
data = {'Date': ['2023-01-15', '2023-01-10', '2023-01-20'],
'Value': [25, 30, 15]}
df = pd.DataFrame(data)
df['Date'] = pd.to_datetime(df['Date'])
Handling the Error:
Specify the ascending parameter to control the sorting order.
# Sorting in descending order
df_sorted_desc = df.sort_values(by='Date', ascending=False)
print(df_sorted_desc)
This will output:
Date Value
2 2023-01-20 15
0 2023-01-15 25
1 2023-01-10 30
Conclusion
Sorting a Pandas dataframe by date is a crucial step in analyzing and visualizing time-series data. In this article, we discussed how to sort a Pandas dataframe by date using datetime objects. We showed how to create a sample dataframe and sort it in ascending and descending order. With these techniques, you can easily sort large datasets by date and gain insights into your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.