How to Solve GPU Out of Memory Error on Google Colab
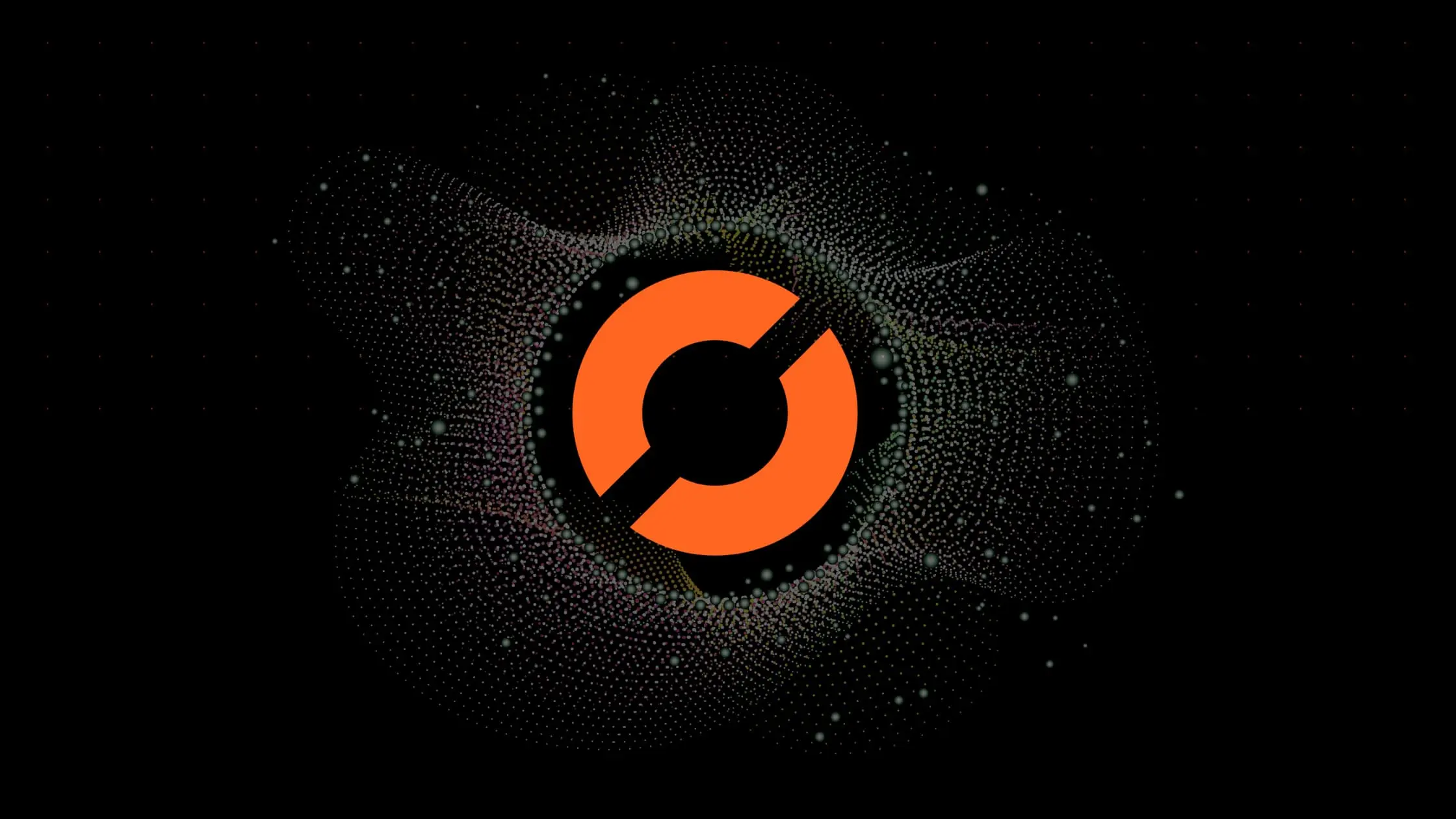
As a data scientist or software engineer, you have probably encountered the dreaded “GPU out of memory” error message while running your machine learning models on Google Colab. This error message can be frustrating and time-consuming, especially when you are working on a complex model that takes several hours to train. In this article, we will explore the causes of the GPU out of memory error and provide some tips on how to solve it.
Table of Contents
What Causes the GPU Out of Memory Error?
Before we dive into the solutions, it is important to understand what causes the GPU out of memory error. The error message occurs when the GPU does not have enough memory to complete the task assigned to it. This can happen for several reasons, including:
- The model is too large for the GPU memory
- The batch size is too large
- The number of layers in the model is too high
- The GPU is being used by another process
How to Solve the GPU Out of Memory Error
There are several ways to solve the GPU out of memory error on Google Colab. Here are some of the most effective methods:
Method 1: Reduce the Batch Size
One of the easiest ways to reduce the memory usage of your model is to reduce the batch size. The batch size determines how many samples are processed at once during training. By reducing the batch size, you can reduce the amount of memory required to train the model. However, keep in mind that reducing the batch size may also increase the training time.
# Before
batch_size = 64
# After
batch_size = 32
Method 2: Reduce the Model Size
If reducing the batch size does not solve the problem, you may need to reduce the size of your model. This can be done by reducing the number of layers in the model or by using a smaller model architecture. You can also try using transfer learning to train your model on a pre-trained model, which can significantly reduce the memory usage.
Method 3: Use Mixed Precision Training
Mixed precision training is a technique that uses lower-precision data types to reduce the memory usage of the model. By using lower-precision data types, you can reduce the memory required to store the model parameters and activations. This technique can significantly reduce the memory usage without compromising the accuracy of the model.
from torch.cuda.amp import autocast, GradScaler
scaler = GradScaler()
# Before
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
# After
with autocast():
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
scaler.scale(loss).backward()
scaler.step(optimizer)
scaler.update()
Method 4: Use Gradient Checkpointing
Gradient checkpointing is a technique that allows you to trade-off compute for memory. Instead of storing all the intermediate activations during training, you can store only a subset of them. This can significantly reduce the memory usage of the model and allow you to train larger models.
# Training loop with gradient checkpointing
def train_epoch(model, train_loader, criterion, optimizer):
model.train()
for inputs, labels in train_loader:
inputs, labels = inputs.to(device), labels.to(device)
# Use gradient checkpointing
checkpoint_inputs = torch.utils.checkpoint.checkpoint(model, inputs)
optimizer.zero_grad()
outputs = model(checkpoint_inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Method 5: Use Multiple GPUs
If you have access to multiple GPUs, you can use them to train your model in parallel. This can significantly reduce the training time and also reduce the memory usage, as each GPU will be responsible for a smaller portion of the model.
# Initialize the model, move it to GPU, and use DataParallel
model = SimpleCNN().to(device)
if torch.cuda.device_count() > 1:
print(f"Using {torch.cuda.device_count()} GPUs")
model = nn.DataParallel(model)
Method 6: Use a Larger Memory GPU
If none of the above methods work, you may need to use a larger memory GPU. Google Colab provides access to several different types of GPUs, ranging from 12GB to 16GB of memory. By switching to a larger memory GPU, you can train larger models without running into memory issues.
Method 7: Utilizing Google Colab Pro
Google Colab Pro offers additional GPU memory compared to the free version. Upgrading to Colab Pro can be a viable solution for users consistently encountering GPU memory limitations.
Method 8: Transfer Learning
Transfer learning allows you to leverage pre-trained models, reducing the need for extensive training on your end. We’ll provide examples of how to implement transfer learning in Colab to save GPU memory.
# Code example for transfer learning
from tensorflow.keras.applications import VGG16
base_model = VGG16(weights='imagenet', include_top=False)
Conclusion
The GPU out of memory error on Google Colab can be a frustrating issue for data scientists and software engineers. However, by understanding the causes of the error and implementing the solutions outlined in this article, you can overcome this issue and train your machine learning models without running into memory issues. Remember to experiment with different methods and find the one that works best for your specific use case.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.