How to Show All Column Names on a Large Pandas DataFrame
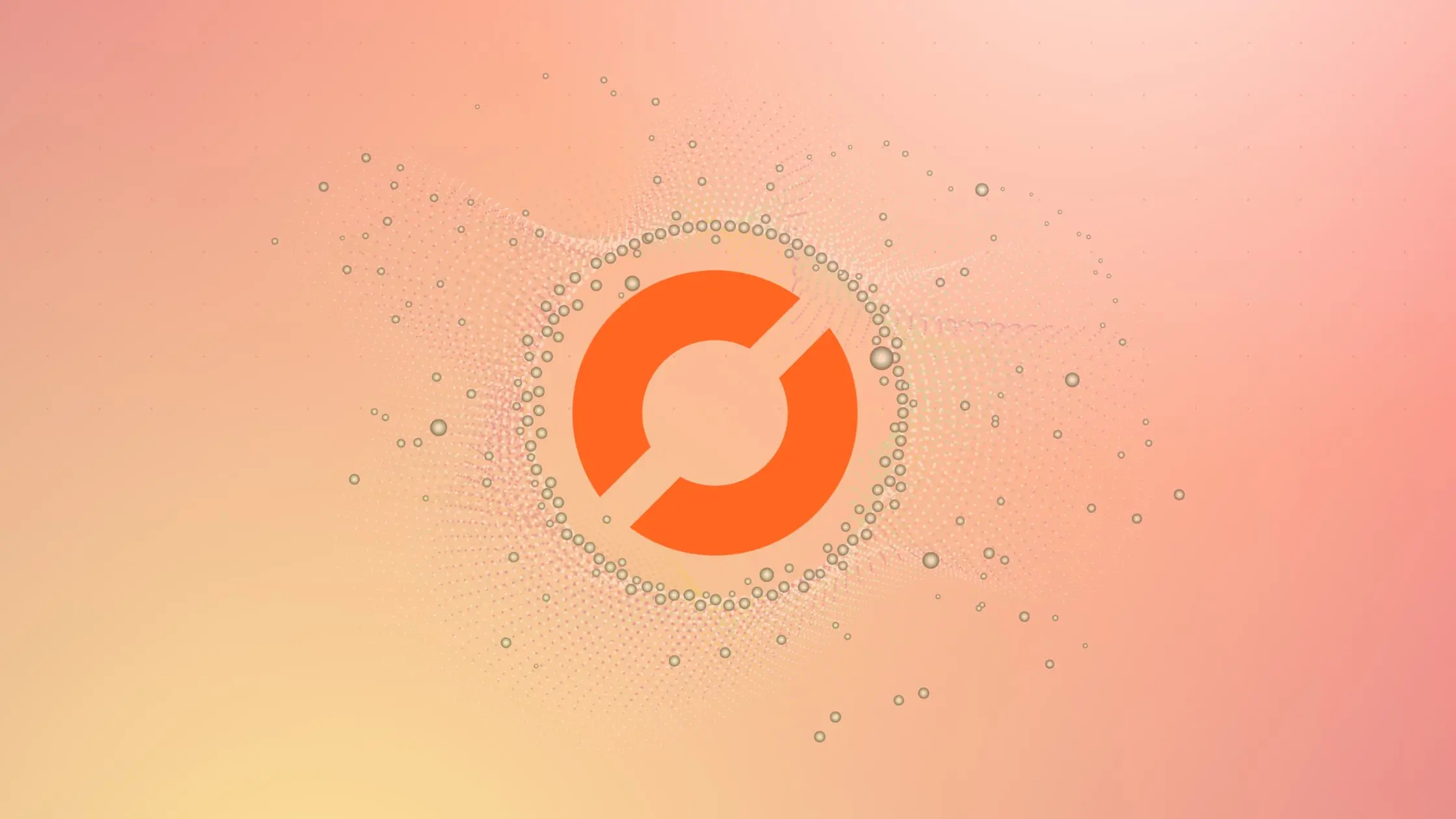
As a data scientist or software engineer, you have probably encountered a situation where you need to work with a large pandas dataframe with many columns. When working with such a dataframe, it can be difficult to view all the column names, especially if the columns are not displayed due to the width of the dataframe.
In this blog post, we will explore how to show all column names on a large pandas dataframe using simple and efficient methods.
Table of Contents
- What is Pandas?
- The Challenge
- Method 1: Using the pd.options.display.max_columns Parameter
- Method 2: Using the DataFrame.columns Attribute
- Method 3: Using the DataFrame.info() Method
- Conclusion
What is Pandas?
Pandas is a popular data analysis library in Python that provides data structures and tools for data manipulation and analysis. It is widely used in data science and machine learning projects.
One of the core data structures in Pandas is the DataFrame, which is a two-dimensional table-like data structure with rows and columns. A DataFrame is a powerful tool that allows data scientists to work with large datasets.
The Challenge of Displaying All Column Names on a Large Pandas DataFrame
When working with a large pandas dataframe, it can be challenging to view all column names if the columns are not displayed due to the width of the dataframe. By default, the pandas dataframe shows only a subset of the columns, and the rest are truncated.
This can be frustrating, especially when you need to work with a large dataset with many columns. Fortunately, there are several ways to show all column names on a large pandas dataframe.
Let’s say we have a random DataFrame that has 1000 rows and 50 columns. When you display it, the output will be truncated as shown below:
# Create a large DataFrame with 1000 rows and 50 columns
df = pd.DataFrame(np.random.rand(1000, 50), columns=[f'col_{i}' for i in range(50)])
print(df)
Output:
col_0 col_1 col_2 col_3 col_4 ... col_45 \
0 0.964616 0.236512 0.714823 0.451715 0.161998 ... 0.759156
1 0.160201 0.593552 0.410760 0.949468 0.687854 ... 0.165778
2 0.085469 0.677406 0.996336 0.156234 0.038921 ... 0.702069
3 0.404221 0.524581 0.541092 0.525515 0.541101 ... 0.712994
4 0.765372 0.294593 0.518726 0.368666 0.102627 ... 0.794924
.. ... ... ... ... ... ... ...
995 0.318816 0.607031 0.961981 0.449906 0.605990 ... 0.413964
996 0.408033 0.521799 0.816650 0.950018 0.154797 ... 0.386669
997 0.229494 0.265283 0.581489 0.521771 0.044155 ... 0.906805
998 0.916877 0.229309 0.485294 0.711102 0.299946 ... 0.611196
999 0.947845 0.463052 0.695537 0.852888 0.826098 ... 0.858327
col_46 col_47 col_48 col_49
0 0.236325 0.093809 0.615526 0.078678
1 0.761265 0.657471 0.933473 0.139356
2 0.016272 0.031140 0.657813 0.434276
3 0.268237 0.308290 0.945084 0.224147
4 0.369746 0.427940 0.356022 0.505767
.. ... ... ... ...
995 0.935928 0.686979 0.349809 0.795637
996 0.466581 0.812713 0.375606 0.675781
997 0.407140 0.464321 0.876408 0.989232
998 0.353962 0.656394 0.675275 0.821299
999 0.688065 0.678142 0.496741 0.225537
[1000 rows x 50 columns]
Method 1: Using the pd.options.display.max_columns Parameter
The simplest way to display all column names on a large pandas dataframe is to use the pd.options.display.max_columns
parameter. This parameter controls the maximum number of columns that Pandas displays.
To set the pd.options.display.max_columns
parameter to show all columns, you can use the following code:
import pandas as pd
pd.options.display.max_columns = None
print(df)
This code sets the pd.options.display.max_columns
parameter to None, which means that Pandas will display all columns.
Output:
col_0 col_1 col_2 col_3 col_4 col_5 col_6 \
0 0.153717 0.482612 0.831060 0.077882 0.289472 0.467470 0.965212
1 0.753398 0.592403 0.256186 0.385437 0.974246 0.311077 0.298807
2 0.494298 0.667306 0.099722 0.329681 0.334417 0.686789 0.743527
3 0.706999 0.923867 0.341464 0.815451 0.190278 0.354622 0.781376
4 0.161814 0.847634 0.162079 0.116882 0.234656 0.774938 0.567430
.. ... ... ... ... ... ... ...
995 0.343012 0.916642 0.206359 0.487503 0.666251 0.530213 0.673208
996 0.386813 0.794457 0.689360 0.815614 0.956183 0.521029 0.675205
997 0.061997 0.998701 0.795018 0.955766 0.087892 0.752429 0.078475
998 0.586326 0.689030 0.133247 0.785062 0.929016 0.127573 0.860095
999 0.152820 0.722834 0.388162 0.174324 0.070073 0.261502 0.555507
col_7 col_8 col_9 col_10 col_11 col_12 col_13 \
0 0.064808 0.276789 0.977565 0.540970 0.581865 0.846636 0.446658
1 0.902976 0.551107 0.496794 0.282331 0.689882 0.109611 0.716832
2 0.774651 0.322931 0.263034 0.690746 0.818504 0.526758 0.528841
3 0.037405 0.818513 0.689578 0.102399 0.795942 0.021479 0.770433
4 0.180333 0.119362 0.261067 0.067885 0.444463 0.668978 0.547032
.. ... ... ... ... ... ... ...
995 0.867552 0.204308 0.157197 0.610779 0.058301 0.548629 0.105525
996 0.378360 0.795320 0.560457 0.454346 0.059251 0.940875 0.471719
997 0.606062 0.094666 0.286443 0.274784 0.552347 0.534680 0.028932
998 0.095685 0.608516 0.466998 0.136260 0.543215 0.530210 0.201358
999 0.672376 0.288557 0.844070 0.219242 0.594211 0.774128 0.758904
col_14 col_15 col_16 col_17 col_18 col_19 col_20 \
0 0.157853 0.858069 0.691384 0.097937 0.529731 0.335217 0.516953
1 0.796044 0.483558 0.129574 0.311156 0.011691 0.989641 0.034566
2 0.385424 0.301513 0.535031 0.960461 0.491448 0.317463 0.919824
3 0.618826 0.395679 0.004772 0.667339 0.200393 0.922259 0.758442
4 0.948167 0.797001 0.313806 0.195133 0.800427 0.063825 0.287324
.. ... ... ... ... ... ... ...
995 0.336403 0.160305 0.902384 0.333265 0.031580 0.030954 0.722896
996 0.444248 0.720805 0.668845 0.920229 0.892215 0.866361 0.763199
997 0.649125 0.644163 0.309692 0.097460 0.993488 0.885684 0.193746
998 0.872590 0.123234 0.500231 0.658634 0.752794 0.594370 0.554094
999 0.343469 0.606472 0.724897 0.150611 0.747608 0.049472 0.924962
col_21 col_22 col_23 col_24 col_25 col_26 col_27 \
0 0.869054 0.115983 0.781765 0.456945 0.998403 0.546874 0.128295
1 0.262757 0.124925 0.263503 0.417877 0.804679 0.355874 0.357750
2 0.486958 0.763892 0.594275 0.786688 0.064658 0.293554 0.691706
3 0.236898 0.022522 0.186116 0.192795 0.414632 0.256097 0.840114
4 0.812371 0.394920 0.376737 0.397080 0.976586 0.868973 0.041196
.. ... ... ... ... ... ... ...
995 0.541643 0.505638 0.474656 0.642070 0.654075 0.372817 0.823473
996 0.584527 0.268562 0.694579 0.544153 0.399260 0.260127 0.440994
997 0.360960 0.006208 0.429259 0.802490 0.405759 0.393156 0.155887
998 0.891960 0.974717 0.519034 0.354690 0.642086 0.106520 0.358574
999 0.676138 0.422191 0.531630 0.053351 0.436653 0.850496 0.955167
col_28 col_29 col_30 col_31 col_32 col_33 col_34 \
0 0.612715 0.289769 0.262615 0.397411 0.973778 0.229357 0.027246
1 0.836455 0.131338 0.460692 0.874707 0.497715 0.144222 0.431756
2 0.526487 0.421510 0.772382 0.170965 0.884607 0.877887 0.090390
3 0.406056 0.871964 0.246394 0.061501 0.548550 0.280167 0.114024
4 0.167982 0.556702 0.453225 0.321289 0.086918 0.142730 0.509901
.. ... ... ... ... ... ... ...
995 0.313636 0.252033 0.352655 0.513922 0.712625 0.676948 0.965147
996 0.380124 0.677867 0.144330 0.297111 0.402275 0.753710 0.513965
997 0.732346 0.041922 0.263013 0.637607 0.554263 0.112356 0.069624
998 0.415643 0.764130 0.018093 0.536630 0.496172 0.085398 0.795797
999 0.851988 0.241698 0.076521 0.376431 0.204558 0.003570 0.490827
col_35 col_36 col_37 col_38 col_39 col_40 col_41 \
0 0.969778 0.890687 0.252725 0.958133 0.955823 0.694491 0.357555
1 0.211286 0.738304 0.956136 0.332277 0.709432 0.977291 0.338518
2 0.382878 0.341724 0.505131 0.969127 0.254081 0.420203 0.873372
3 0.849604 0.587547 0.619855 0.879578 0.504818 0.169264 0.320221
4 0.368632 0.134104 0.901714 0.921227 0.006675 0.737873 0.873520
.. ... ... ... ... ... ... ...
995 0.332006 0.589716 0.286749 0.735156 0.254230 0.766034 0.187530
996 0.541045 0.007886 0.566133 0.717299 0.310796 0.893926 0.006056
997 0.986283 0.692556 0.537410 0.162068 0.461754 0.984682 0.936976
998 0.466028 0.485508 0.285970 0.865334 0.254768 0.717602 0.198167
999 0.907145 0.787205 0.398355 0.221750 0.154364 0.601802 0.580466
col_42 col_43 col_44 col_45 col_46 col_47 col_48 \
0 0.664041 0.422512 0.646385 0.107367 0.442005 0.570606 0.604091
1 0.298868 0.370593 0.365926 0.911279 0.809941 0.902058 0.939920
2 0.499322 0.532290 0.278293 0.933268 0.802350 0.422360 0.113506
3 0.393836 0.680546 0.361885 0.549631 0.259546 0.476253 0.508761
4 0.470585 0.548421 0.456551 0.032836 0.204966 0.718750 0.309230
.. ... ... ... ... ... ... ...
995 0.231127 0.344774 0.217120 0.695439 0.525060 0.617114 0.618750
996 0.128004 0.369993 0.885262 0.665682 0.589731 0.852448 0.528447
997 0.640847 0.470862 0.713722 0.246703 0.030507 0.944230 0.972758
998 0.061010 0.991973 0.567517 0.328101 0.715328 0.974949 0.646360
999 0.618688 0.601053 0.491485 0.882420 0.383666 0.246607 0.338111
col_49
0 0.610033
1 0.851141
2 0.944832
3 0.836301
4 0.973903
.. ...
995 0.947736
996 0.366277
997 0.205147
998 0.672895
999 0.459406
[1000 rows x 50 columns]
Method 2: Using the DataFrame.columns Attribute
Another way to display all column names on a large pandas dataframe is to use the DataFrame.columns
attribute. This attribute returns a list of all column names in the dataframe.
To use this method, you can simply call the DataFrame.columns
attribute on your dataframe, as shown below:
import pandas as pd
df = pd.read_csv('large_dataframe.csv')
print(df.columns)
This code will print all column names in the df
dataframe.
Output:
Index(['col_0', 'col_1', 'col_2', 'col_3', 'col_4', 'col_5', 'col_6', 'col_7',
'col_8', 'col_9', 'col_10', 'col_11', 'col_12', 'col_13', 'col_14',
'col_15', 'col_16', 'col_17', 'col_18', 'col_19', 'col_20', 'col_21',
'col_22', 'col_23', 'col_24', 'col_25', 'col_26', 'col_27', 'col_28',
'col_29', 'col_30', 'col_31', 'col_32', 'col_33', 'col_34', 'col_35',
'col_36', 'col_37', 'col_38', 'col_39', 'col_40', 'col_41', 'col_42',
'col_43', 'col_44', 'col_45', 'col_46', 'col_47', 'col_48', 'col_49'],
dtype='object')
Method 3: Using the DataFrame.info() Method
The DataFrame.info()
method is another way to display all column names on a large pandas dataframe. This method provides a summary of the dataframe, including the column names, data types, and memory usage.
To use this method, you can call the DataFrame.info()
method on your dataframe, as shown below:
import pandas as pd
df = pd.read_csv('large_dataframe.csv')
df.info()
This code will print a summary of the df
dataframe, including all column names.
Output:
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 1000 entries, 0 to 999
Data columns (total 50 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 col_0 1000 non-null float64
1 col_1 1000 non-null float64
2 col_2 1000 non-null float64
3 col_3 1000 non-null float64
4 col_4 1000 non-null float64
5 col_5 1000 non-null float64
6 col_6 1000 non-null float64
7 col_7 1000 non-null float64
8 col_8 1000 non-null float64
9 col_9 1000 non-null float64
10 col_10 1000 non-null float64
11 col_11 1000 non-null float64
12 col_12 1000 non-null float64
13 col_13 1000 non-null float64
14 col_14 1000 non-null float64
15 col_15 1000 non-null float64
16 col_16 1000 non-null float64
17 col_17 1000 non-null float64
18 col_18 1000 non-null float64
19 col_19 1000 non-null float64
20 col_20 1000 non-null float64
21 col_21 1000 non-null float64
22 col_22 1000 non-null float64
23 col_23 1000 non-null float64
24 col_24 1000 non-null float64
25 col_25 1000 non-null float64
26 col_26 1000 non-null float64
27 col_27 1000 non-null float64
28 col_28 1000 non-null float64
29 col_29 1000 non-null float64
30 col_30 1000 non-null float64
31 col_31 1000 non-null float64
32 col_32 1000 non-null float64
33 col_33 1000 non-null float64
34 col_34 1000 non-null float64
35 col_35 1000 non-null float64
36 col_36 1000 non-null float64
37 col_37 1000 non-null float64
38 col_38 1000 non-null float64
39 col_39 1000 non-null float64
40 col_40 1000 non-null float64
41 col_41 1000 non-null float64
42 col_42 1000 non-null float64
43 col_43 1000 non-null float64
44 col_44 1000 non-null float64
45 col_45 1000 non-null float64
46 col_46 1000 non-null float64
47 col_47 1000 non-null float64
48 col_48 1000 non-null float64
49 col_49 1000 non-null float64
dtypes: float64(50)
memory usage: 390.8 KB
Conclusion
In this blog post, we have explored different methods of displaying all column names on a large pandas dataframe. By using the pd.options.display.max_columns
parameter, the DataFrame.columns
attribute, and the DataFrame.info()
method, data scientists and software engineers can easily view all column names in a dataframe without truncation.
When working with large datasets, it is essential to have a good understanding of the tools and techniques available to manipulate and analyze the data. Pandas is a powerful library that provides data scientists with a rich set of tools for working with data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.