How to Shift a Column in Pandas DataFrame
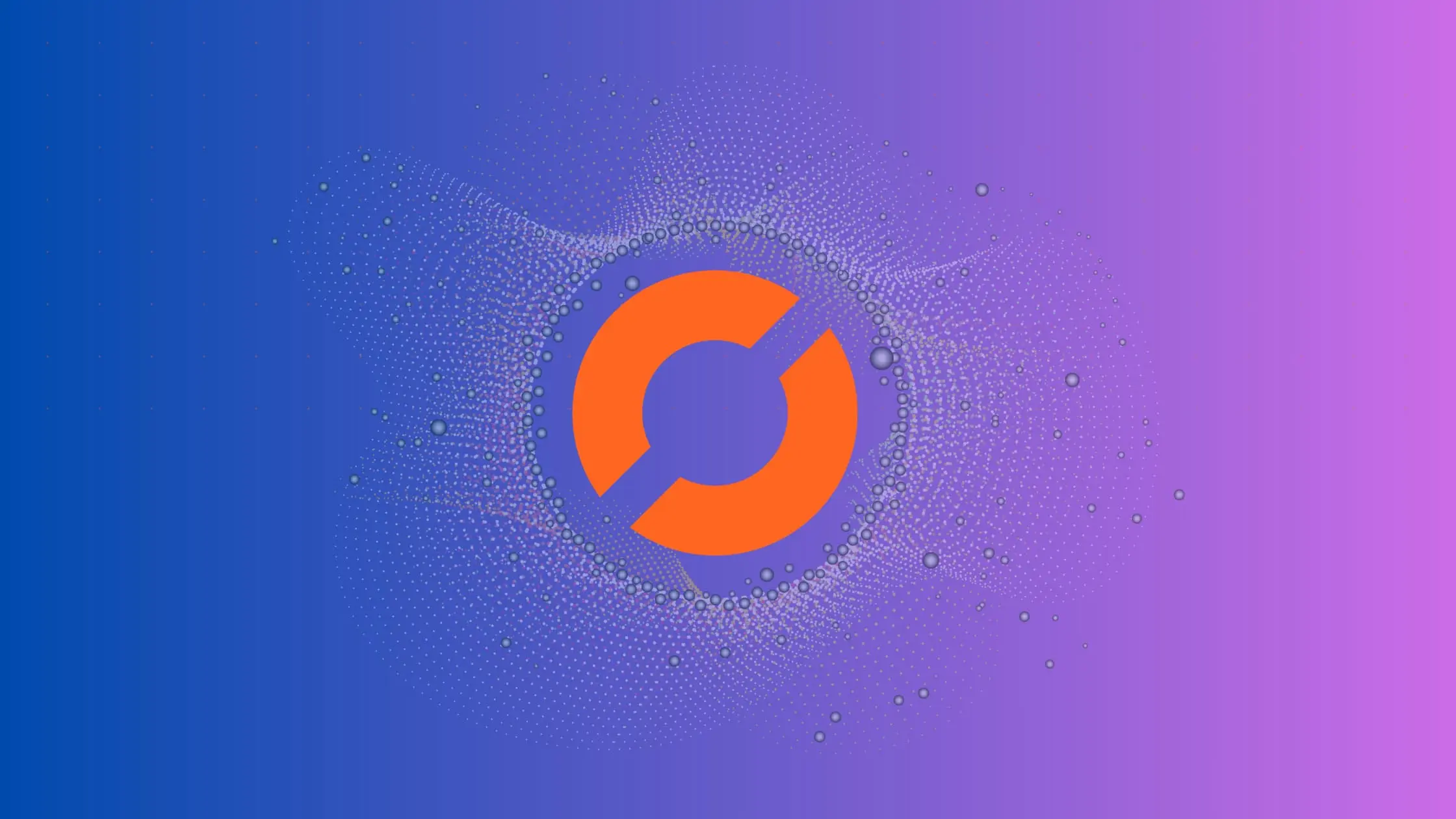
As a data scientist or software engineer working with data, you have probably come across the need to shift a column in a Pandas DataFrame. Whether you need to move a column to a different position or simply shift its values up or down, Pandas provides a simple and efficient way to achieve this.
In this article, we will explain how to shift a column in a Pandas DataFrame, including practical examples and best practices.
Table of Contents
- What is Pandas?
- Shifting a Column in Pandas DataFrame
- Shifting Multiple Columns in Pandas DataFrame
- Shifting a Column to a Different Position
- Conclusion
What is Pandas?
Pandas is a powerful Python library for data manipulation and analysis. It provides data structures and functions for handling and processing large amounts of data in a flexible and efficient way. One of the main data structures in Pandas is the DataFrame, which is similar to a table in a relational database. DataFrames are widely used in data science and software engineering for cleaning, transforming, and analyzing data.
Shifting a Column in Pandas DataFrame
Shifting a column in a Pandas DataFrame means moving its values up or down by a certain number of rows. This can be useful for various reasons, such as aligning data with different time intervals or preparing data for time series analysis.
To shift a column in a Pandas DataFrame, we can use the shift()
function. This function takes a single argument, which is the number of rows to shift the column by. If the argument is positive, the column values are shifted downwards, and if it is negative, the column values are shifted upwards.
Original DataFrame:
Name Age Salary
0 John 30 50000
1 Jane 25 60000
2 Alice 35 70000
3 Bob 40 80000
Here is an example of shifting a column downwards by one row:
import pandas as pd
# create a sample DataFrame
data = {'Name': ['John', 'Jane', 'Alice', 'Bob'],
'Age': [30, 25, 35, 40],
'Salary': [50000, 60000, 70000, 80000]}
df = pd.DataFrame(data)
# shift the 'Salary' column downwards by one row
df['Salary'] = df['Salary'].shift(1)
print(df)
The output of this code will be:
Name Age Salary
0 John 30 NaN
1 Jane 25 50000.0
2 Alice 35 60000.0
3 Bob 40 70000.0
As you can see, the shift()
function has moved the values in the ‘Salary’ column downwards by one row. The first row of the column now contains a NaN
value, which is the result of shifting the first value downwards.
We can also shift a column upwards by using a negative argument to the shift()
function. Here is an example:
# shift the 'Age' column upwards by two rows
df['Age'] = df['Age'].shift(-2)
print(df)
The output of this code will be:
Name Age Salary
0 John 35.0 NaN
1 Jane 40.0 50000.0
2 Alice NaN 60000.0
3 Bob NaN 70000.0
As you can see, the shift()
function has moved the values in the ‘Age’ column upwards by two rows. The last two rows of the column now contain NaN
values, which are the result of shifting the last values upwards.
Shifting Multiple Columns in Pandas DataFrame
In addition to shifting a single column, we can also shift multiple columns in a Pandas DataFrame. To do this, we can simply pass a list of column names to the shift()
function.
Here is an example of shifting two columns downwards by one row:
# shift the 'Age' and 'Salary' columns downwards by one row
df[['Age', 'Salary']] = df[['Age', 'Salary']].shift(1)
print(df)
The output of this code will be:
Name Age Salary
0 John NaN NaN
1 Jane 30.0 50000.0
2 Alice 25.0 60000.0
3 Bob 35.0 70000.0
As you can see, the shift()
function has moved the values in the ‘Age’ and ‘Salary’ columns downwards by one row.
Shifting a Column to a Different Position
In addition to shifting a column up or down, we may also want to move a column to a different position in the DataFrame. To do this, we can use the insert()
function, which allows us to insert a column at a specific position in the DataFrame.
Here is an example of moving the ‘Salary’ column to the first position in the DataFrame:
# move the 'Salary' column to the first position in the DataFrame
salary_col = df.pop('Salary')
df.insert(0, 'Salary', salary_col)
print(df)
The output of this code will be:
Salary Name Age
0 NaN John NaN
1 50000.0 Jane 30.0
2 60000.0 Alice 25.0
3 70000.0 Bob 35.0
As you can see, the insert()
function has moved the ‘Salary’ column to the first position in the DataFrame.
Conclusion
Shifting a column in a Pandas DataFrame is a simple and useful operation that can be used for various data manipulation tasks. By using the shift()
function, we can move column values up or down by a certain number of rows. We can also shift multiple columns at once and move a column to a different position in the DataFrame.
We hope this article has been helpful in explaining how to shift a column in Pandas DataFrame. By mastering this technique, you can improve your data manipulation and analysis skills and become a more efficient data scientist or software engineer.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.