How to Set the First Column and Row as Index in Pandas
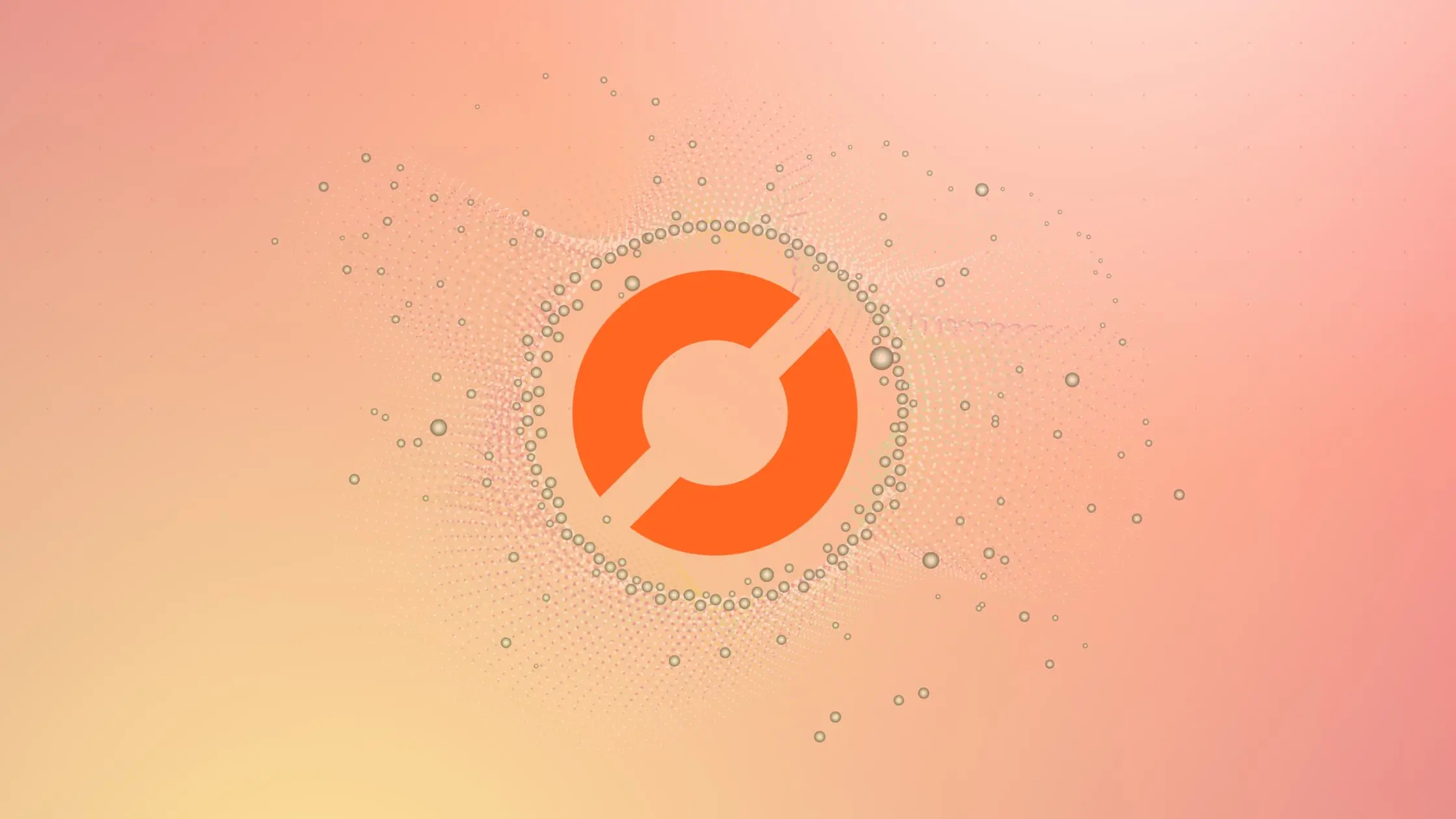
How to Set the First Column and Row as Index in Pandas
As a data scientist or software engineer, working with data is a crucial part of the job. One of the most popular tools for data manipulation and analysis is pandas, a powerful data manipulation library for Python. In this post, we will discuss how to set the first column and row as index in pandas, a common task when working with data.
What is an Index in Pandas?
Before we dive into how to set the first column and row as index in pandas, let’s first understand what an index is in pandas. In pandas, an index is a way of identifying rows and columns in a DataFrame. An index can be a label or a numerical value that uniquely identifies each row or column in a DataFrame. By default, pandas assigns a numerical index to each row of a DataFrame, starting from zero.
However, in some cases, it may be more useful to assign a specific column or row as the index of a DataFrame. For example, if you have a DataFrame that contains sales data for different products, you may want to set the product names as the index so that you can easily look up sales data for a specific product.
How to Set the First Column as Index in Pandas
To set the first column as index in pandas, we can use the set_index()
method. The set_index()
method takes a column name or a list of column names as the argument and sets the specified column(s) as the index of the DataFrame. Here’s an example:
import pandas as pd
# create a sample DataFrame
data = {'Product': ['Product A', 'Product B', 'Product C', 'Product D'],
'Sales': [100, 200, 300, 400],
'Profit': [10, 20, 30, 40]}
df = pd.DataFrame(data)
# set the 'Product' column as index
df.set_index('Product', inplace=True)
print(df)
Output:
Sales Profit
Product
Product A 100 10
Product B 200 20
Product C 300 30
Product D 400 40
In this example, we created a sample DataFrame with three columns: Product, Sales, and Profit. We then used the set_index()
method to set the ‘Product’ column as the index of the DataFrame. The inplace=True
argument is used to modify the original DataFrame instead of creating a new one.
How to Set the First Row as Index in Pandas
To set the first row as index in pandas, we can use a combination of the header
and index_col
arguments of the read_csv()
function. The header
argument specifies the row number(s) to use as the column names, and the index_col
argument specifies the column(s) to use as the index.
Here’s an example:
import pandas as pd
# create a sample CSV file
csv_data = 'Product,Sales,Profit\nProduct A,100,10\nProduct B,200,20\nProduct C,300,30\nProduct D,400,40\n'
with open('sales_data.csv', 'w') as f:
f.write(csv_data)
# read the CSV file and set the first row as index
df = pd.read_csv('sales_data.csv', header=0, index_col=0)
print(df)
Output:
Sales Profit
Product
Product A 100 10
Product B 200 20
Product C 300 30
Product D 400 40
In this example, we created a sample CSV file with sales data for different products. We then used the read_csv()
function to read the CSV file and set the first row as the index of the DataFrame by specifying header=0
and index_col=0
arguments.
How to Set the First Column and Row as Index in Pandas
To set the first column and row as index in pandas, we can use a combination of the set_index()
method and the header
and index_col
arguments of the read_csv()
function.
Here’s an example:
import pandas as pd
# create a sample CSV file
csv_data = ',Product A,Product B,Product C,Product D\nSales,100,200,300,400\nProfit,10,20,30,40\n'
with open('sales_data.csv', 'w') as f:
f.write(csv_data)
# read the CSV file and set the first column and row as index
df = pd.read_csv('sales_data.csv', header=[0], index_col=[0])
df.index.name = None # remove index name
print(df)
Output:
Product A Product B Product C Product D
Sales 100 200 300 400
Profit 10 20 30 40
In this example, we created a sample CSV file with sales data for different products. We then used the read_csv()
function to read the CSV file and set the first row as the column names and the first column as the index of the DataFrame by specifying header=[0]
and index_col=[0]
arguments. We also removed the index name using the df.index.name = None
statement.
Conclusion
In this post, we discussed how to set the first column and row as index in pandas, a common task when working with data. We covered three different methods to achieve this: using the set_index()
method to set the first column as index, using a combination of the header
and index_col
arguments of the read_csv()
function to set the first row as index, and using a combination of the set_index()
method and the header
and index_col
arguments of the read_csv()
function to set the first column and row as index.
By mastering these techniques, you can become more efficient at working with data in pandas and better equipped to handle real-world data analysis tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.