How to Select Specific CSV Columns Using Python and Pandas
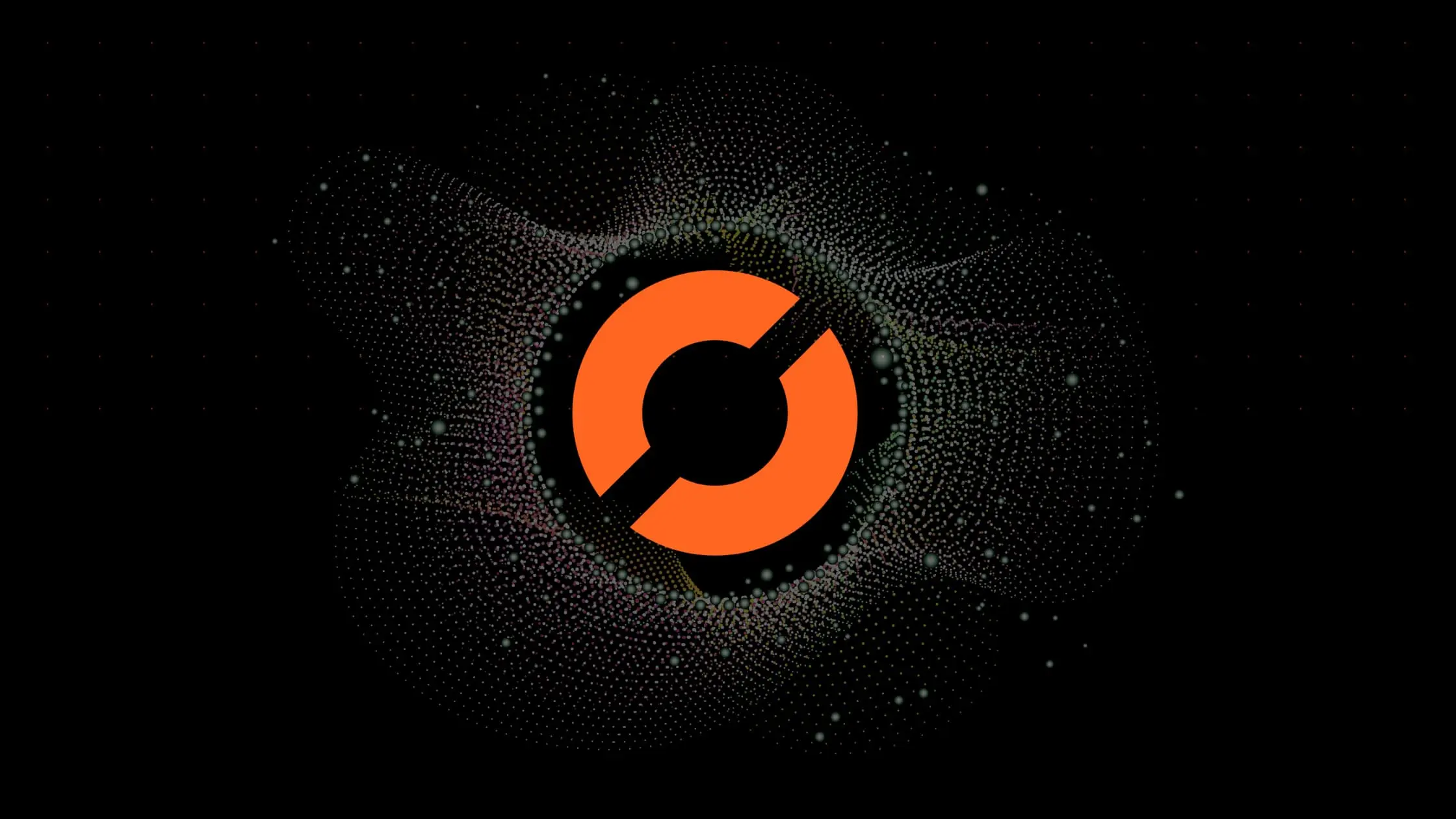
How to Select Specific CSV Columns Using Python and Pandas
As a data scientist or software engineer, you often work with large datasets in various formats, including CSV files. CSV files are common, and they are widely used to store tabular data. However, when working with CSV files, you might need to select specific columns of data from the file. This process is known as filtering, and it can be done using Python and Pandas.
In this article, we will explain how to select specific CSV columns using Python and Pandas. We will provide step-by-step instructions, examples, and code snippets to help you understand the process.
What Is Pandas?
Pandas is a powerful open-source data analysis library for Python. It provides high-performance data manipulation tools and data structures designed for working with structured data. Pandas is built on top of the NumPy library, which makes it easy to integrate with other scientific computing libraries.
Pandas is widely used for data analysis, data cleaning, data manipulation, and data visualization. It provides a wide range of functions and methods for working with data, including reading and writing data from various formats, selecting and filtering data, merging and joining data, grouping data, and much more.
How to Select Specific CSV Columns Using Pandas
To select specific CSV columns using Pandas, you need to follow these steps:
- Import the Pandas library
- Load the CSV file into a Pandas dataframe
- Select the specific columns you want to keep
- Save the filtered data to a new CSV file
Step 1: Import the Pandas Library
Before you can use Pandas, you need to import the library into your Python environment. You can do this by running the following code:
import pandas as pd
Step 2: Load the CSV File into a Pandas Dataframe
To work with the CSV file, you need to load it into a Pandas dataframe. You can use the read_csv()
function from Pandas to do this. The read_csv()
function takes the path to the CSV file as an argument and returns a dataframe containing the data.
df = pd.read_csv('path/to/csv/file.csv')
Step 3: Select the Specific Columns You Want to Keep
Once you have loaded the CSV file into a dataframe, you can select the specific columns you want to keep. To do this, you need to use the square bracket notation [ ]
with a list of column names as the argument.
df = df[['column_name_1', 'column_name_2', 'column_name_3']]
In the above code, we are selecting three specific columns: column_name_1
, column_name_2
, and column_name_3
. You can replace these column names with the names of the columns you want to keep.
Step 4: Save the Filtered Data to a New CSV File
Finally, you can save the filtered data to a new CSV file using the to_csv()
function from Pandas. The to_csv()
function takes the path to the new CSV file as an argument.
df.to_csv('path/to/new/csv/file.csv', index=False)
In the above code, we are saving the filtered data to a new CSV file located at path/to/new/csv/file.csv
. The index=False
argument tells Pandas not to include the index column in the new CSV file.
Conclusion
Filtering specific CSV columns using Python and Pandas is a common task in data analysis and data manipulation. In this article, we explained how to select specific CSV columns using Pandas. We provided step-by-step instructions, and code snippets to help you understand the process.
By following the steps outlined in this article, you should be able to filter specific CSV columns using Python and Pandas. This will help you work more efficiently with large datasets and extract the information you need from them.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.