How to Select Date Range from Pandas DataFrame
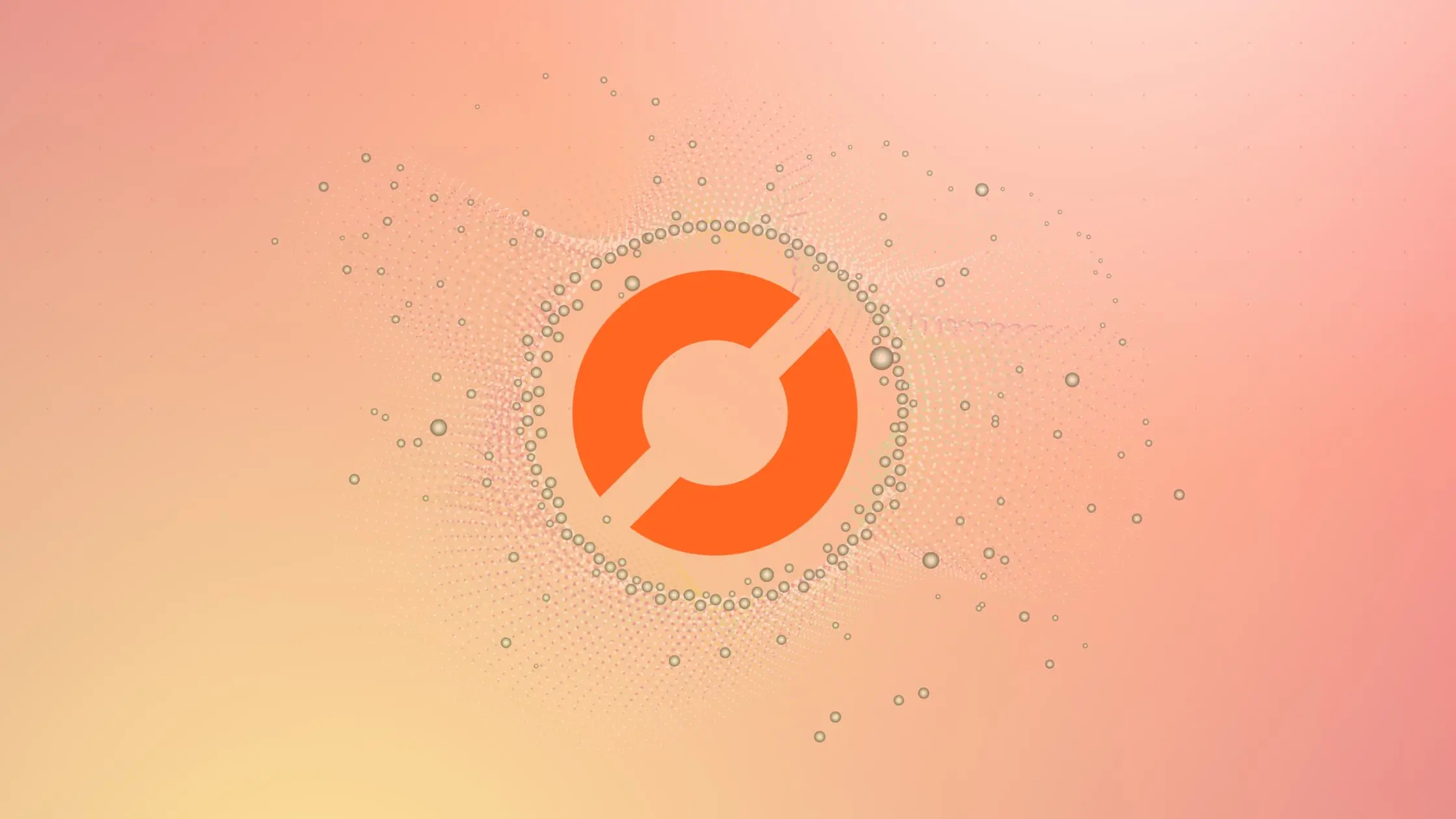
As a data scientist or software engineer, you may often encounter the need to select a specific date range from a dataset. Pandas is a powerful Python library that provides various functionalities for data manipulation, including selecting date ranges from DataFrames. In this article, we will explore how to select date ranges from a Pandas DataFrame.
Table of Contents
- Introduction
- Selecting a Date Range from a Pandas DataFrame
- Filtering a Pandas DataFrame by Date Range Using
.loc
- Conclusion
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table, where data is organized in rows and columns. The rows are labeled with an index, and the columns are labeled with column names. A Pandas DataFrame can be created from various data sources, such as CSV files, Excel spreadsheets, SQL databases, and more.
Selecting a Date Range from a Pandas DataFrame
To select a date range from a Pandas DataFrame, we first need to ensure that the DataFrame contains a column with dates. We can convert a column with dates to a Pandas DateTimeIndex using the pd.to_datetime()
function.
import pandas as pd
# Create a sample DataFrame with dates
df = pd.DataFrame({'date': ['2022-01-01', '2022-01-02', '2022-01-03', '2022-01-04'],
'value': [1, 2, 3, 4]})
# Convert the 'date' column to a Pandas DateTimeIndex
df['date'] = pd.to_datetime(df['date'])
df = df.set_index('date')
In the code above, we created a sample DataFrame with a ‘date’ column and a ‘value’ column. We then converted the ‘date’ column to a Pandas DateTimeIndex using the pd.to_datetime()
function and set it as the index of the DataFrame using the df.set_index()
method.
Now that we have a DataFrame with a DateTimeIndex, we can select a date range using the df.loc[]
method. The df.loc[]
method is used to access rows and columns by label or boolean array. We can use the :
operator to select a range of dates.
# Select a date range from the DataFrame
date_range = df.loc['2022-01-02':'2022-01-03']
print(date_range)
Output:
value
date
2022-01-02 2
2022-01-03 3
In the code above, we selected a date range from the DataFrame using the df.loc[]
method. We specified the start date and end date of the range using the :
operator. The resulting date_range
variable contains the rows of the DataFrame that fall within the specified date range.
Filtering a Pandas DataFrame by Date Range Using .loc
When working with time-series data in Pandas, it is common to filter a DataFrame based on a specific date range. While one approach involves creating a boolean array directly from the df['date']
column, a more robust and recommended method, especially when the DataFrame’s index is a DateTimeIndex, is to use the .loc accessor.
Here’s an example:
# Select a date range from the DataFrame
date_range = df.loc['2022-01-02':'2022-01-03']
print(date_range)
Output:
value
date
2022-01-02 2
2022-01-03 3
In this revised code snippet, we employ the .loc
accessor to filter the DataFrame based on the DateTimeIndex. The syntax df.loc[start_date:end_date]
allows us to specify a range of dates directly. The resulting date_range
DataFrame contains only the rows corresponding to the selected date range.
Using .loc
for date-based indexing not only enhances readability but also ensures compatibility with various datetime-related operations. This approach is particularly advantageous when working with large datasets or when multiple date-based operations are performed, making it a recommended practice for filtering Pandas DataFrames by date range
Conclusion
In this article, we explored how to select a date range from a Pandas DataFrame. We learned how to convert a column with dates to a Pandas DateTimeIndex and how to use the df.loc[]
method and boolean indexing to select a date range. Pandas provides a flexible and powerful way to manipulate and analyze data, and selecting date ranges is just one of the many functionalities it offers. With this knowledge, you can now easily select the date ranges you need for your data analysis projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.