How to Select Data from a Pandas Dataframe using Startswith
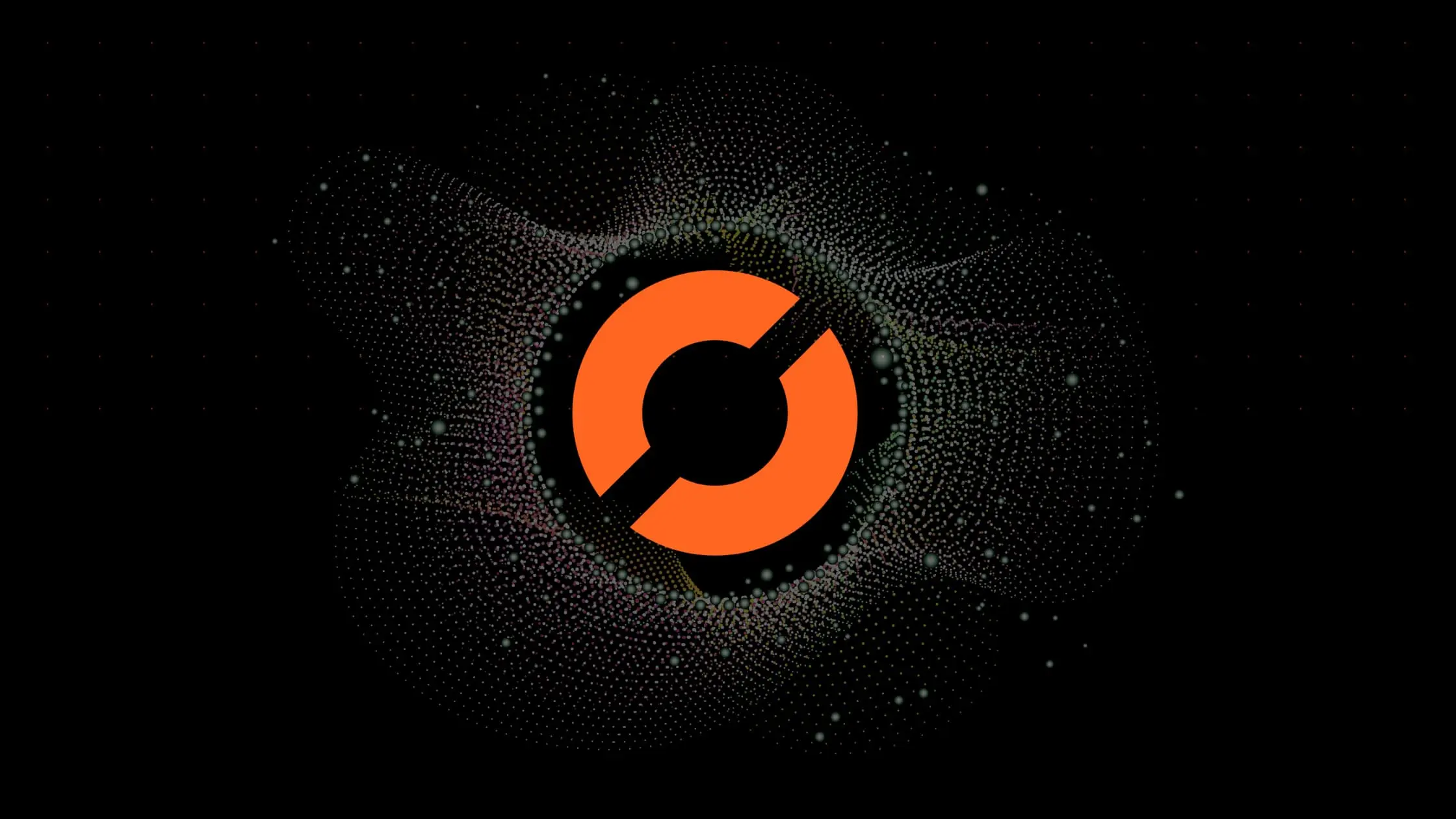
As a data scientist or software engineer, working with large datasets is a common occurrence. One of the most popular tools for working with data in Python is the Pandas library. Pandas is a powerful library that provides data structures and functions that help you manipulate and analyze data efficiently.
In this article, we will discuss how to select data from a Pandas Dataframe using startswith
. We will cover the following topics:
- What is a Pandas Dataframe?
- What is startswith?
- How to select data from a Pandas Dataframe using startswith?
- Examples of using startswith in Pandas Dataframes
Table of Contents
- What is a Pandas Dataframe?
- What is startswith?
- How to select data from a Pandas Dataframe using startswith?
- Examples of using startswith in Pandas Dataframes
- Common Errors and How to Handle Them
- Conclusion
1. What is a Pandas Dataframe?
A Pandas Dataframe is a two-dimensional data structure that consists of rows and columns. It is a table-like data structure that is used to store and manipulate data in Python. A Pandas Dataframe can be created using various data sources like CSV, Excel, SQL databases, and more. Once a Dataframe is created, you can perform various operations like filtering, grouping, sorting, and aggregating the data.
2. What is startswith?
startswith
is a string method in Python that returns True
if a string starts with a specified prefix. It returns False
otherwise. The syntax of startswith
is as follows:
string.startswith(prefix, start, end)
Here, string
is the string to be checked, prefix
is the string to be searched at the beginning of string
, start
is the starting index of the search, and end
is the ending index of the search.
3. How to select data from a Pandas Dataframe using startswith?
Now that we know what a Pandas Dataframe is and what startswith
is, let’s see how to use startswith
to select data from a Pandas Dataframe.
To select data from a Pandas Dataframe using startswith
, we can use the str.startswith()
method provided by Pandas. This method returns a Boolean series that indicates whether each string in the specified column starts with the specified prefix. We can use this Boolean series to filter the rows of the Dataframe.
The syntax of str.startswith()
is as follows:
df['column_name'].str.startswith('prefix')
Here, df
is the Pandas Dataframe, column_name
is the name of the column to be searched, and prefix
is the prefix to be searched for.
Let’s see an example. Suppose we have a Pandas Dataframe named df
with the following data:
import pandas as pd
df = pd.DataFrame({
'Name': ['John', 'Jane', 'Alex', 'Mary', 'David'],
'Age': [25, 30, 35, 40, 45],
'Country': ['USA', 'Canada', 'USA', 'UK', 'USA']
})
Now, let’s say we want to select all the rows where the Country
column starts with the prefix "U"
. We can use the following code:
df[df['Country'].str.startswith('U')]
This will return the following output:
Name Age Country
0 John 25 USA
2 Alex 35 USA
3 Mary 40 UK
4 David 45 USA
As you can see, only the rows where the Country
column starts with the prefix "U"
are selected.
4. Examples of using startswith in Pandas Dataframes
Let’s see some more examples of using startswith
in Pandas Dataframes.
Example 1: Selecting rows based on a prefix in a column
Suppose we have a Pandas Dataframe with the following data:
import pandas as pd
df = pd.DataFrame({
'Name': ['John', 'Jane', 'Alex', 'Mary', 'David'],
'Age': [25, 30, 35, 40, 45],
'Country': ['USA', 'Canada', 'USA', 'UK', 'USA']
})
If we want to select all the rows where the Name
column starts with the prefix "J"
, we can use the following code:
df[df['Name'].str.startswith('J')]
This will return the following output:
Name Age Country
0 John 25 USA
1 Jane 30 Canada
Example 2: Counting the number of rows based on a prefix in a column
Suppose we have a Pandas Dataframe with the following data:
import pandas as pd
df = pd.DataFrame({
'Name': ['John', 'Jane', 'Alex', 'Mary', 'David'],
'Age': [25, 30, 35, 40, 45],
'Country': ['USA', 'Canada', 'USA', 'UK', 'USA']
})
If we want to count the number of rows where the Country
column starts with the prefix "U"
, we can use the following code:
df['Country'].str.startswith('U').sum()
This will return the following output:
3
Example 3: Modifying values based on a prefix in a column
Suppose we have a Pandas Dataframe with the following data:
import pandas as pd
df = pd.DataFrame({
'Name': ['John', 'Jane', 'Alex', 'Mary', 'David'],
'Age': [25, 30, 35, 40, 45],
'Country': ['USA', 'Canada', 'USA', 'UK', 'USA']
})
If we want to replace all the values in the Country
column that start with the prefix "U"
with "United States"
, we can use the following code:
df.loc[df['Country'].str.startswith('U'), 'Country'] = 'United States'
This will modify the Country
column as follows:
Name Age Country
0 John 25 United States
1 Jane 30 Canada
2 Alex 35 United States
3 Mary 40 United States
4 David 45 United States
5. Common Errors and How to Handle Them
Error 1: Incorrect Column Name
Ensure that the column name used in df['column_name'].str.startswith('prefix')
is spelled correctly. A misspelled column name will result in a KeyError.
Error 2: Case Sensitivity
startswith
is case-sensitive. Double-check the case of your prefix to ensure accurate matching.
Error 3: Missing or Null Values
If there are missing or null values in the column, they might lead to unexpected results. Consider handling or removing such values before using startswith
.
6. Conclusion
In this article, we discussed how to select data from a Pandas Dataframe using startswith
. We learned that we can use the str.startswith()
method provided by Pandas to filter the rows of the Dataframe based on a prefix in a column. We also saw some examples of using startswith
in Pandas Dataframes to select, count, and modify data. Using startswith
in Pandas Dataframes can help you manipulate and analyze data efficiently, and can be a useful tool in your data science and software engineering projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.