How to Select a Range of Values in a Pandas Dataframe Column
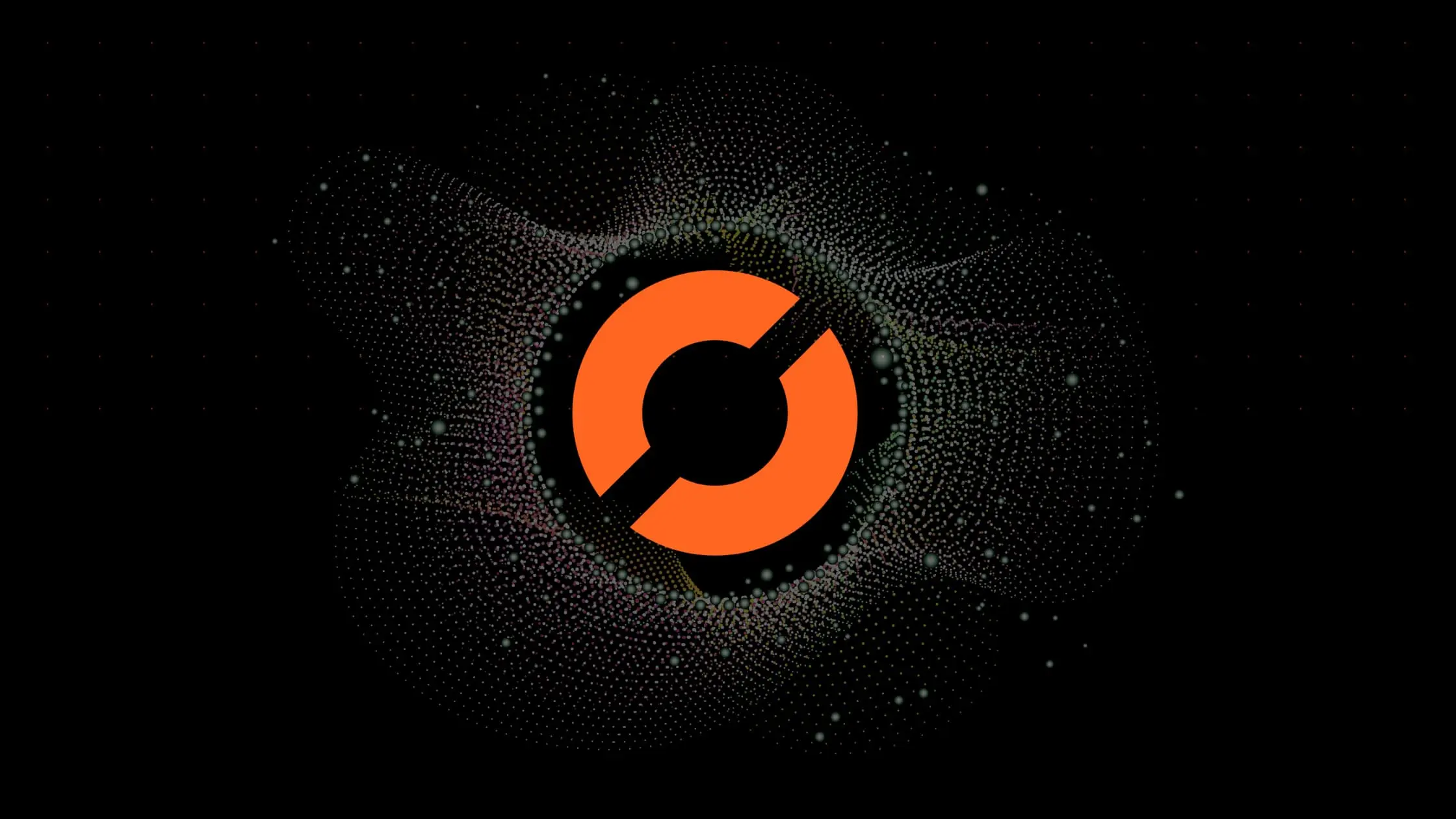
As a data scientist or software engineer working with data, one of the most common tasks you’ll face is selecting a range of values from a pandas dataframe column. This can be done using various methods, but in this article, we’ll explore some of the most efficient and effective techniques for selecting a range of values in a pandas dataframe column.
Table of Contents
- What is Pandas?
- Selecting a Range of Values in a Pandas Dataframe Column
- Common Errors and Solutions
- Conclusion
What is Pandas?
Before we dive into the specifics of selecting a range of values in a pandas dataframe column, let’s take a moment to define what pandas is. Pandas is a popular data analysis library for Python that provides fast, flexible, and expressive data structures designed to make working with data easy and intuitive. It is built on top of the NumPy library and integrates well with other libraries such as Matplotlib and Scikit-Learn.
Selecting a Range of Values in a Pandas Dataframe Column
When working with data in pandas, you’ll often need to select a subset of the data that meets certain criteria or falls within a certain range. In this section, we’ll explore some of the most common techniques for selecting a range of values in a pandas dataframe column.
Using the loc
Method
The loc
method is one of the most commonly used techniques for selecting data in pandas. It allows you to select data from a dataframe based on row labels and column labels, and can be used to select a range of values from a dataframe column. Here’s an example:
import pandas as pd
# create a dataframe
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emily'],
'Age': [25, 30, 35, 40, 45],
'Salary': [50000, 60000, 70000, 80000, 90000]}
df = pd.DataFrame(data)
# select a range of values from the Age column
age_range = df.loc[(df['Age'] >= 30) & (df['Age'] <= 40), 'Age']
print(age_range)
In this example, we create a dataframe with three columns: Name, Age, and Salary. We then use the loc
method to select a range of values from the Age column, where the age is between 30 and 40. The resulting output is a pandas series containing the selected values from the Age column.
Output:
1 30
2 35
3 40
Name: Age, dtype: int64
Using the iloc
Method
The iloc
method is similar to the loc method, but instead of selecting data based on row labels and column labels, it selects data based on integer positions. Here’s an example:
import pandas as pd
# create a dataframe
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emily'],
'Age': [25, 30, 35, 40, 45],
'Salary': [50000, 60000, 70000, 80000, 90000]}
df = pd.DataFrame(data)
# select a range of values from the Age column
age_range = df.iloc[1:4, 1]
print(age_range)
In this example, we create a dataframe with three columns: Name, Age, and Salary. We then use the iloc
method to select a range of values from the Age column, where the age is between the second and fourth row. The resulting output is a pandas series containing the selected values from the Age column.
Output:
1 30
2 35
3 40
Name: Age, dtype: int64
Using Boolean Indexing
Boolean indexing is another technique that can be used to select a range of values in a pandas dataframe column. It involves creating a boolean mask that indicates which values in the dataframe meet certain criteria, and then using that mask to select the desired values. Here’s an example:
import pandas as pd
# create a dataframe
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emily'],
'Age': [25, 30, 35, 40, 45],
'Salary': [50000, 60000, 70000, 80000, 90000]}
df = pd.DataFrame(data)
# create a boolean mask to select values in the Age column
mask = (df['Age'] >= 30) & (df['Age'] <= 40)
# select values in the Age column based on the boolean mask
age_range = df.loc[mask, 'Age']
print(age_range)
In this example, we create a dataframe with three columns: Name, Age, and Salary. We then create a boolean mask to select values in the Age column where the age is between 30 and 40. We use the loc method to select the desired values from the Age column, based on the boolean mask. The resulting output is a pandas series containing the selected values from the Age column.
Output:
1 30
2 35
3 40
Name: Age, dtype: int64
Common Errors and Solutions
Incorrect Syntax in Boolean Indexing:
- Error: One common mistake is using incorrect syntax when creating a boolean mask.
- Solution: Ensure that the conditions in the boolean mask are properly enclosed in parentheses. For example, in the boolean mask creation, use
(df['Age'] >= 30) & (df['Age'] <= 40)
instead ofdf['Age'] >= 30 & df['Age'] <= 40
. The correct use of parentheses is crucial to avoid logical errors.
Indexing Out of Range in iloc Method:
- Error: When using the
iloc
method, indexing beyond the dataframe’s range can lead to errors. - Solution: Be cautious with the start and end index values in the
iloc
method. Ensure that the specified range is within the actual number of rows and columns in your dataframe. In the example provided,df.iloc[1:4, 1]
selects the second to fourth rows from the second column. If the dataframe had only three rows or two columns, this would result in an error. Always check the dimensions of your dataframe before using theiloc
method.
- Error: When using the
Using loc on Nonexistent Columns:
- Error: When using the
loc
method, attempting to select values from a column that doesn’t exist in the dataframe can lead to errors. - Solution: Double-check the column name and make sure it exists in the dataframe. In the example, if you try to select
df.loc[mask, 'NonexistentColumn']
, and ‘NonexistentColumn’ is not a valid column in your dataframe, an error will occur. Always verify column names and ensure they match the actual columns in your dataframe.
- Error: When using the
Mixing up DataFrame and Series Operations:
- Error: Incorrectly mixing DataFrame and Series operations can lead to unexpected results.
- Solution: When using methods like
loc
oriloc
, make sure you are selecting and working with DataFrames, not Series. For instance, in the examples provided, the selected data should be assigned to a DataFrame (df.loc[mask, ['Age']]
) rather than just a Series (df.loc[mask, 'Age']
). This ensures consistency in data structures and avoids potential errors in subsequent operations.
Conclusion
Selecting a range of values in a pandas dataframe column is a common task that data scientists and software engineers face when working with data. In this article, we explored some of the most efficient and effective techniques for selecting a range of values in a pandas dataframe column, including using the loc method, the iloc method, and boolean indexing. By using these techniques, you can quickly and easily select the desired subset of data from your pandas dataframe, making it easier to analyze and gain insights from your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.