How to Save a Trained Model in PyTorch?
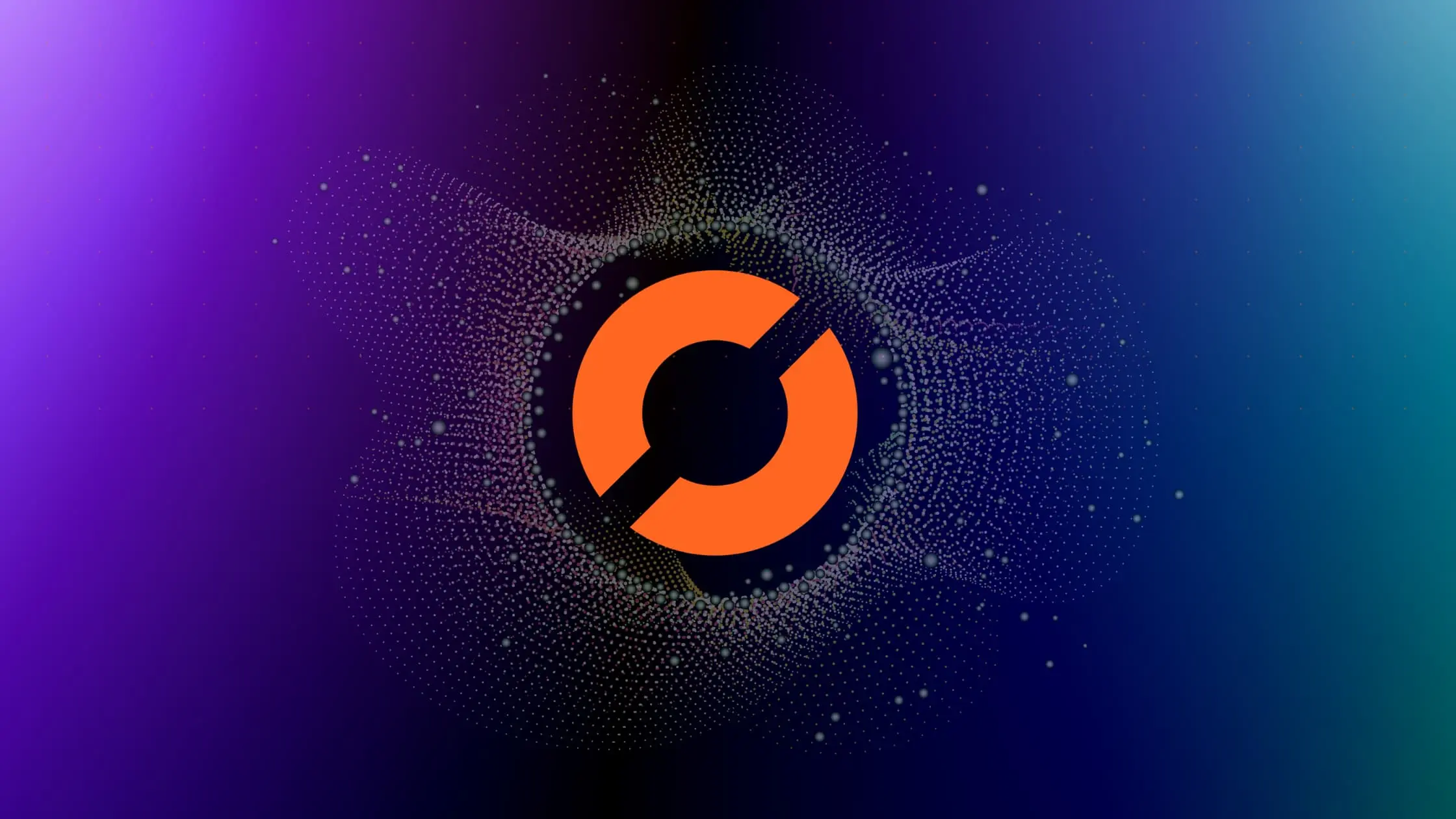
As a data scientist, one of the most important tasks in machine learning is to save a trained model so that it can be used in the future. In PyTorch, the process of saving a trained model is quite straightforward. In this post, we will walk you through the steps to save a trained model in PyTorch.
Table of Contents
- Why Save a Trained Model?
- How to Save a Trained Model in PyTorch
- Pros and Cons of each method
- Common Errors and Solutions
- Conclusion
Why Save a Trained Model?
Before we dive into the details of how to save a trained model in PyTorch, let’s first understand why you should save a trained model.
When you train a machine learning model, you invest a lot of time, effort, and resources into it. Once you have trained the model, it is important to save it so that you can use it in the future without having to retrain it again. Saving a trained model allows you to:
- Share the model with others
- Use the model to make predictions on new data
- Continue training the model at a later time
How to Save a Trained Model in PyTorch
Now that we understand the importance of saving a trained model, let’s dive into the steps to save a trained model in PyTorch.
Step 1: Define Your Model
To save a trained model, you first need to define your model. In PyTorch, you can define your model using the nn.Module
class. Here’s an example of how to define a simple neural network in PyTorch:
import torch
import torch.nn as nn
class NeuralNet(nn.Module):
def __init__(self):
super(NeuralNet, self).__init__()
self.fc1 = nn.Linear(2, 3)
self.fc2 = nn.Linear(3, 1)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
x = self.fc1(x)
x = self.sigmoid(x)
x = self.fc2(x)
x = self.sigmoid(x)
return x
Step 2: Train Your Model
Once you have defined your model, you need to train it on your data. This involves defining your loss function and optimizer, and then iterating over your data to update the model’s parameters.
model = NeuralNet()
criterion = nn.BCELoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
for epoch in range(num_epochs):
for inputs, labels in dataloader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Step 3: Save Your Trained Model
You can save your trained model using PyTorch’s torch.save()
function. Two options are available: saving only the state dictionary or saving the entire model.
Save the state_dict
only
Once you have trained your model, you can save it to a file using PyTorch’s torch.save()
function. This function takes two arguments: the model you want to save and the file path where you want to save the model.
torch.save(model.state_dict(), 'saved_model.pth')
The state_dict()
method returns a dictionary containing the model’s parameters and their corresponding values. By default, PyTorch saves the model’s state dictionary in a binary format.
Save the entire model
Another option is to save the entire PyTorch model is by using the torch.save()
function. This method serializes the entire model, including its architecture and learned parameters, into a single file.
torch.save(model, 'saved_model.pth')
Step 4: Load Your Trained Model
To load your trained model from the saved file, you can use PyTorch’s torch.load()
function. This function takes one argument: the file path where you saved your model. In case you save the state dict only, you need to define the model before loading the weights.
model = NeuralNet()
model.load_state_dict(torch.load('saved_model.pth'))
This will load the saved model’s state dictionary into your model object. On the other hand, if you saved the entire model, loading is simpler:
model.load_state_dict(torch.load('saved_model.pth'))
Pros and Cons of each method
Saving the state dicts only
Pros:
- Smaller File Size: The saved file is smaller since it only contains the model parameters.
- Flexibility: Separates the model architecture from the parameters, allowing for easier model switching or modification.
Cons:
- Requires Model Architecture: When loading, you need to have the model architecture available for reconstruction.
Saving the entire model
Pros:
- Easy Implementation: It’s a one-liner solution that is easy to implement.
- Complete Serialization: Saves the entire model, making it suitable for transferring to different environments.
Cons:
- Large File Size: The serialized file can be large, especially for complex models.
Common Errors and Solutions
Error 1: Missing Model Architecture
# Loading model state dict without defining the model architecture
model.load_state_dict(torch.load('saved_model.pth')) # Raises an error
Solution: Define the model architecture before loading the state dictionary.
model = SomeModel()
model.load_state_dict(torch.load('saved_model.pth'))
model.eval()
Error 2: Version Mismatch
# Loading a model saved with a different PyTorch version
model = torch.load('saved_model.pth') # Raises an error
Solution: Ensure the PyTorch version is consistent between saving and loading.
Conclusion
Saving a trained model in PyTorch is a crucial step in the machine learning pipeline. In this post, we walked through the steps to save a trained model in PyTorch. By following these steps, you can easily save your trained model and use it in the future for making predictions on new data or continuing to train the model.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.