How to Replace Strings with Numbers in Python Pandas Dataframe
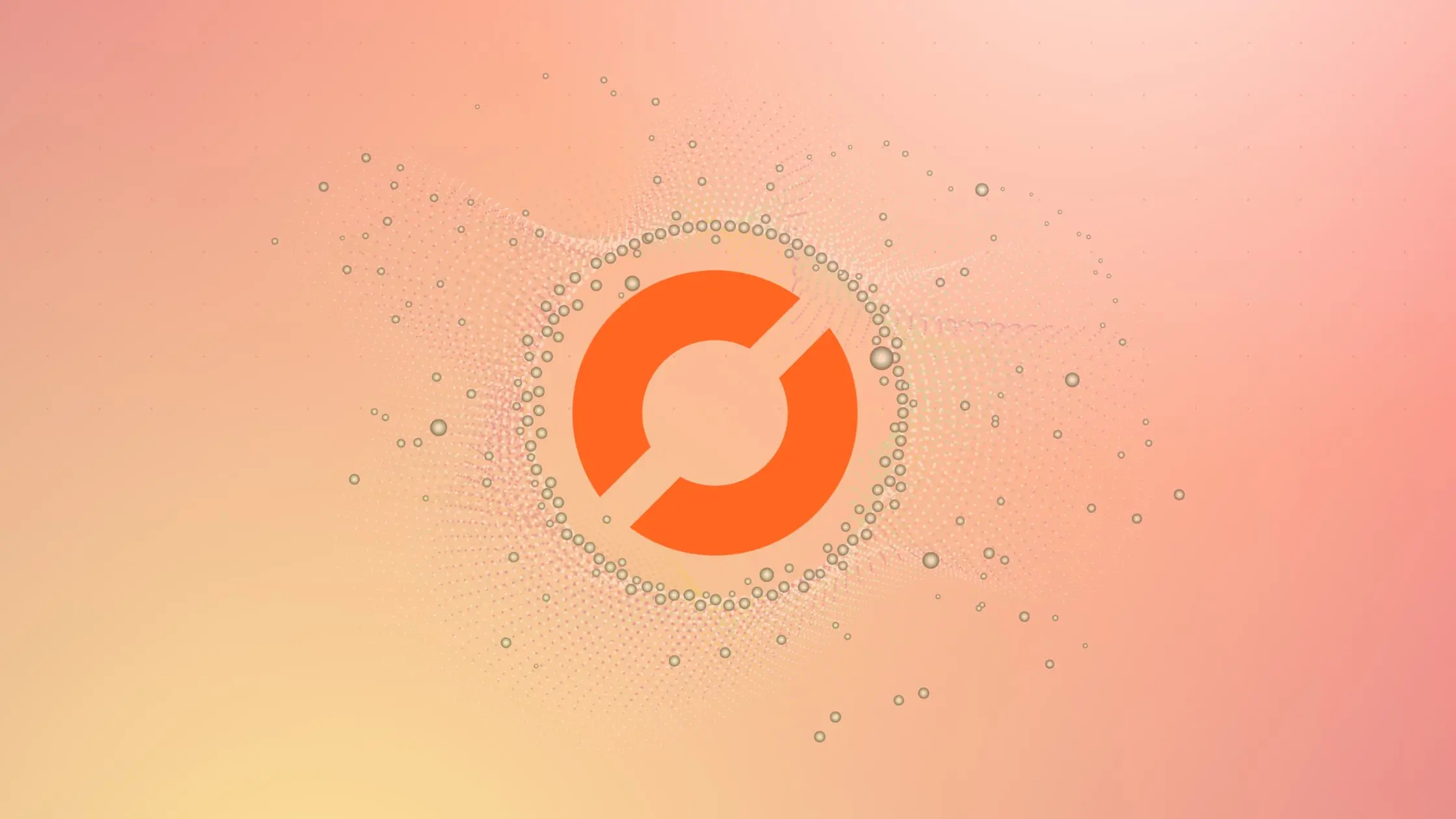
As a data scientist or software engineer, you may often encounter data in the form of strings that need to be converted to numbers for analysis or modeling. Python Pandas is a popular library for data manipulation and analysis, and it provides several methods for replacing strings with numbers in a dataframe. In this article, we will explore these methods and provide examples of their use.
Table of Contents
Introduction 1.1 Method 1: Using the replace() Method 1.2 Method 2: Using the map() Method 1.3 Method 3: Using the astype() Method
Method 1: Using the replace() Method
The replace() method is a convenient way to replace specific strings in a dataframe column with numbers. The syntax for using the replace() method is as follows:
df['column_name'].replace({'string_to_replace': 'replacement_value'}, inplace=True)
Let’s say we have a dataframe with a column named “score” that contains string values “A”, “B”, and “C”. We can replace these values with numeric values 1, 2, and 3, respectively, using the replace() method as follows:
import pandas as pd
df = pd.DataFrame({'score': ['A', 'B', 'C']})
df['score'].replace({'A': 1, 'B': 2, 'C': 3}, inplace=True)
print(df)
The output will be:
score
0 1
1 2
2 3
Note that we used the inplace=True parameter to modify the original dataframe. If you don’t use this parameter, the replace() method will return a new dataframe with the replaced values.
Method 2: Using the map() Method
The map() method is another way to replace strings with numbers in a dataframe column. The map() method takes a dictionary as an argument, where the keys are the strings to be replaced and the values are the replacement values. The syntax for using the map() method is as follows:
df['column_name'] = df['column_name'].map({'string_to_replace': 'replacement_value'})
Using the same example as before, we can replace the string values “A”, “B”, and “C” with numeric values 1, 2, and 3, respectively, using the map() method as follows:
import pandas as pd
df = pd.DataFrame({'score': ['A', 'B', 'C']})
df['score'] = df['score'].map({'A': 1, 'B': 2, 'C': 3})
print(df)
The output will be the same as before:
score
0 1
1 2
2 3
Method 3: Using the astype() Method
The astype() method is a more general way to convert a dataframe column from one data type to another. We can use the astype() method to replace strings with numbers by converting the column to a numeric data type. The syntax for using the astype() method is as follows:
df['column_name'] = df['column_name'].astype('numeric_data_type')
Let’s say we have a dataframe with a column named “age” that contains string values “25”, “30”, and “35”. We can convert these values to numeric values using the astype() method as follows:
import pandas as pd
df = pd.DataFrame({'age': ['25', '30', '35']})
df['age'] = df['age'].astype('int')
print(df)
The output will be:
age
0 25
1 30
2 35
Note that if there are any non-numeric values in the column, the astype() method will raise a ValueError. You can use the to_numeric() method with the ‘coerce’ parameter to convert non-numeric values to NaN:
df['age'] = pd.to_numeric(df['age'], errors='coerce')
Conclusion
Python Pandas provides several methods for replacing strings with numbers in a dataframe. The most common methods are the replace() method, the map() method, and the astype() method. The method you choose depends on the specific requirements of your analysis or modeling task. By using these methods, you can easily convert string values to numeric values and perform data analysis and modeling tasks with ease.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.