How to Replace None with NaN in Pandas DataFrame
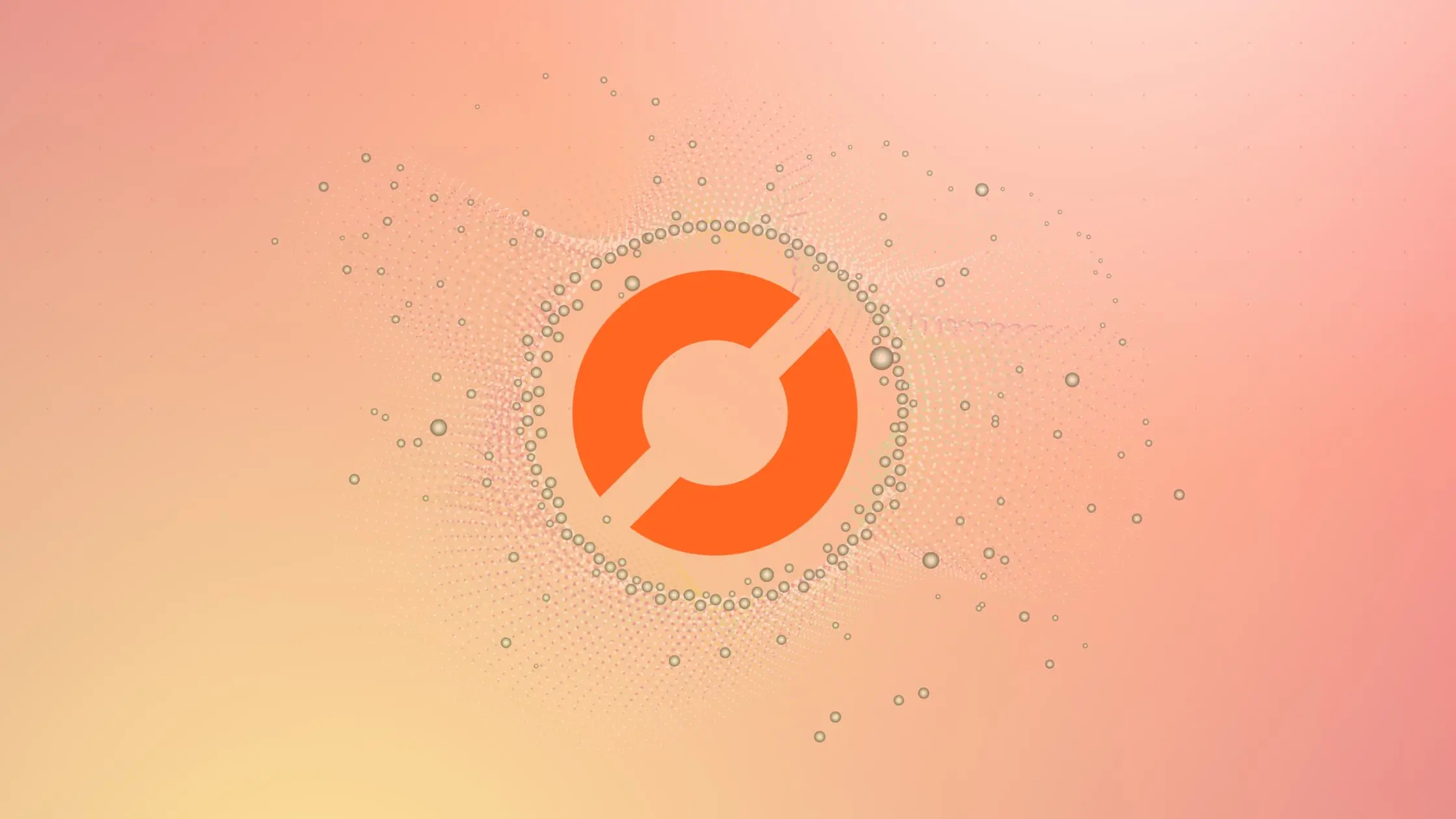
As a data scientist or software engineer, you may come across a situation where you need to replace None
values with NaN
in a Pandas DataFrame. This is a common task when working with data, as NaN
values are often used to represent missing data in Pandas.
In this article, we will explore the various methods to replace None
with NaN
in a Pandas DataFrame. We will cover the following topics:
- Understanding
None
andNaN
- Identifying
None
values in a Pandas DataFrame - Replacing
None
withNaN
using thefillna()
method - Replacing
None
withNaN
using thereplace()
method - Replacing
None
withNaN
using thewhere()
method
Table of Contents
- Understanding None and NaN
- Identifying None values in a Pandas DataFrame
- Replacing None with NaN using the fillna() method
- Replacing None with NaN using the replace() method
- Replacing None with NaN using the where() method
- Conclusion
Understanding None and NaN
Before we dive into the methods to replace None
with NaN
, let’s first understand what None
and NaN
are.
None
is a Python object that represents the absence of a value. It is often used as a placeholder to indicate that a variable has no value. In Pandas, None
is used to represent missing data, but it is not the same as NaN
.
NaN
stands for “Not a Number” and is a special floating-point value that represents undefined or unrepresentable values. In Pandas, NaN
is used to represent missing or null values in a DataFrame.
Identifying None values in a Pandas DataFrame
The first step in replacing None
with NaN
in a Pandas DataFrame is to identify all the None
values in the DataFrame. We can use the isna()
method to check for missing values in a DataFrame. However, isna()
does not consider None
values as missing values, so we need to convert None
values to NaN
before using isna()
.
import pandas as pd
import numpy as np
# Create a sample DataFrame with None values
df = pd.DataFrame({'A': [1, 2, None, 4],
'B': [None, 6, 7, None]})
# Convert None to NaN
df = df.replace({None: np.nan})
# Check for missing values
print(df.isna())
Output:
A B
0 False True
1 False False
2 True False
3 False True
As we can see from the output, the isna()
method now correctly identifies the None
values as missing values.
Replacing None with NaN using the fillna() method
The simplest way to replace None
with NaN
in a Pandas DataFrame is to use the fillna()
method. The fillna()
method replaces missing values with a specified value. We can use np.nan
as the value to replace None
values with NaN
.
# Create a sample DataFrame with None values
df = pd.DataFrame({'A': [1, 2, None, 4],
'B': [None, 6, 7, None]})
# Replace None with NaN using fillna()
df = df.fillna(value=np.nan)
# Check for missing values
print(df.isna())
Output:
A B
0 False True
1 False False
2 True False
3 False True
As we can see from the output, the fillna()
method replaces None
values with NaN
.
Replacing None with NaN using the replace() method
Another way to replace None
with NaN
in a Pandas DataFrame is to use the replace()
method. The replace()
method replaces a specified value with another value in a DataFrame. We can use None
as the value to replace and np.nan
as the value to replace it with.
# Create a sample DataFrame with None values
df = pd.DataFrame({'A': [1, 2, None, 4],
'B': [None, 6, 7, None]})
# Replace None with NaN using replace()
df = df.replace(to_replace=None, value=np.nan)
# Check for missing values
print(df.isna())
Output:
A B
0 False True
1 False False
2 True False
3 False True
As we can see from the output, the replace()
method replaces None
values with NaN
.
Replacing None with NaN using the where() method
The where()
method is another way to replace None
with NaN
in a Pandas DataFrame. The where()
method replaces values where a condition is true. We can use the isnull()
method to create a condition that identifies None
values and replace them with NaN
.
# Create a sample DataFrame with None values
df = pd.DataFrame({'A': [1, 2, None, 4],
'B': [None, 6, 7, None]})
# Replace None with NaN using where()
df = df.where(pd.notna(df), np.nan)
# Check for missing values
print(df.isna())
Output:
A B
0 False True
1 False False
2 True False
3 False True
As we can see from the output, the where()
method replaces None
values with NaN
.
Conclusion
Replacing None
with NaN
in a Pandas DataFrame is a common task when working with data. In this article, we explored various methods to replace None
with NaN
in a Pandas DataFrame. We learned how to identify None
values in a DataFrame and how to replace them using the fillna()
, replace()
, and where()
methods.
By using these methods, you can ensure that your data is clean and ready for analysis, without the risk of missing data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.