How to replace NaN values with the average of columns in pandas DataFrame
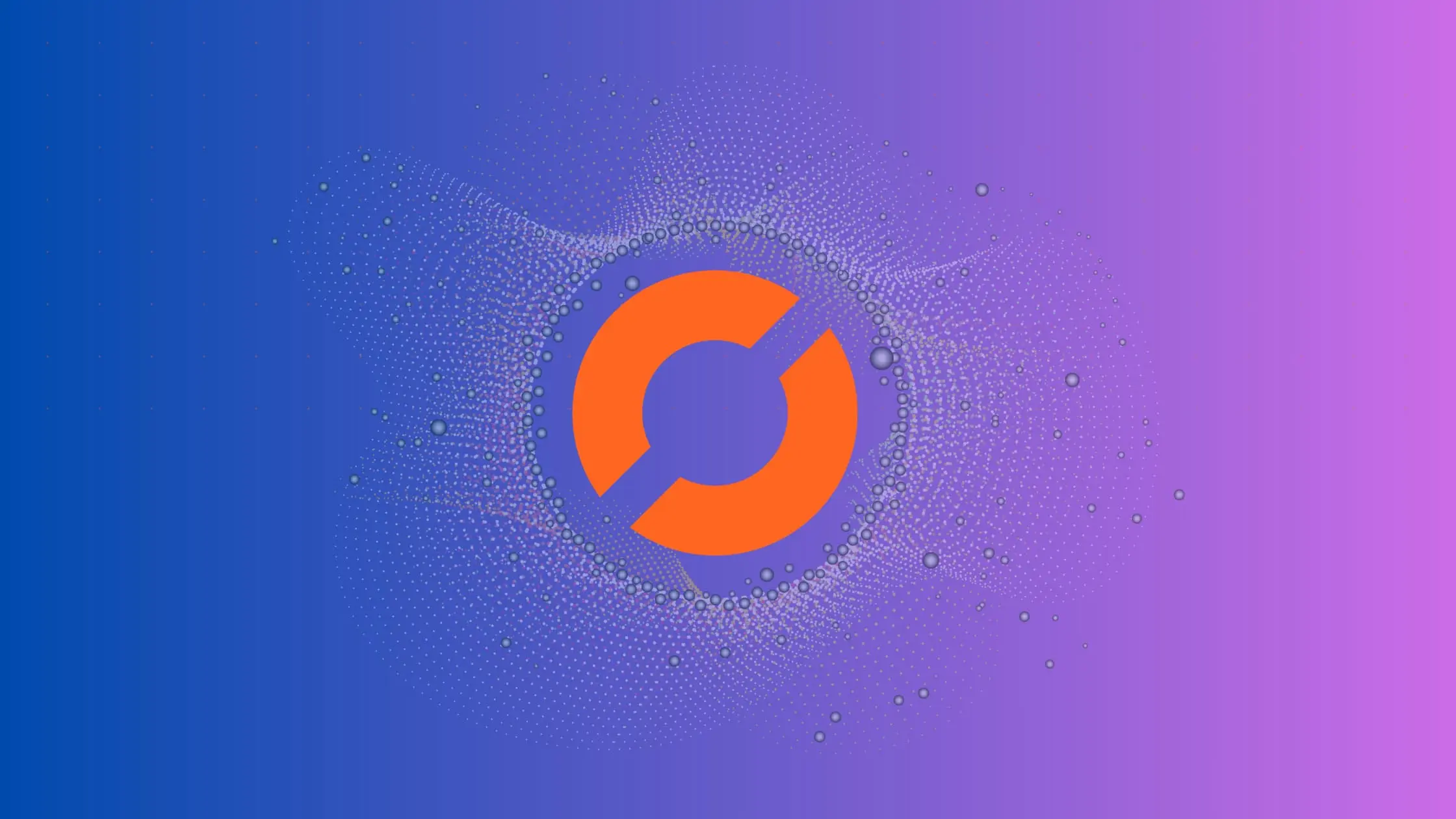
As a data scientist or software engineer, you’ll often encounter datasets that have missing or NaN values. These values can be problematic when running analysis or building machine learning models. One common approach to handling these missing values is to replace them with the average value of the column. In this blog post, we’ll walk through how to do this using the pandas library in Python.
What is pandas?
Pandas is a popular Python library for data manipulation and analysis. It provides data structures and functions for working with tabular data, such as spreadsheets or SQL tables. One of the main data structures in pandas is the DataFrame, which is a two-dimensional table with labeled columns and rows.
How to replace NaN values with the average of columns in pandas DataFrame
To replace NaN values with the average of columns in a pandas DataFrame, we can use the fillna()
method. This method replaces all NaN values with a specified value. We can calculate the average of each column using the mean()
method, which returns a Series containing the average value for each column.
Here’s an example:
import pandas as pd
import numpy as np
# create a sample DataFrame with NaN values
df = pd.DataFrame({
'A': [1, 2, np.nan, 4],
'B': [5, np.nan, 7, 8],
'C': [9, 10, 11, np.nan]
})
# calculate the mean of each column
mean = df.mean()
# fill NaN values with the mean of each column
df.fillna(mean, inplace=True)
print(df)
Output:
A B C
0 1.0 5.0 9.0
1 2.0 6.6 10.0
2 2.3 7.0 11.0
3 4.0 8.0 10.0
In this example, we created a sample DataFrame with NaN values in column A, B, and C. We then calculated the mean of each column using the mean()
method, which returns a Series with the mean of each column. Finally, we replaced the NaN values in the DataFrame with the means of their respective columns using the fillna()
method and passing the mean Series as the fill value.
Pros and Cons of Filling NaN Values with Column Means:
Pros:
- Simplicity: The method is straightforward and easy to implement, making it accessible for users at various skill levels.
- Preservation of Data Structure: The approach retains the structure of the original DataFrame, preventing the introduction of unnecessary complexity.
- Preservation of Central Tendency: Filling NaN values with the average helps maintain the central tendency of the data, which can be important for certain types of analysis and modeling.
Cons:
- Sensitivity to Outliers: The mean is sensitive to extreme values (outliers), and replacing NaN values with the mean might not be appropriate if your dataset contains outliers. This can potentially skew the data.
- Impact on Variability: Filling NaN with the mean may not accurately represent the variability in the data, especially if the distribution is skewed.
- Potential Information Loss: If the missing values follow a pattern or contain valuable information, replacing them with the mean could lead to the loss of important insights.
Common Errors and How to Handle:
- Ignoring Non-Numeric Columns:
- Error: Attempting to calculate the mean on non-numeric columns will result in an error.
- Handling: Before applying the
mean()
method, ensure that the DataFrame contains only numeric columns or use theselect_dtypes
method to filter numeric columns.
numeric_df = df.select_dtypes(include=np.number)
mean = numeric_df.mean()
- Forgetting to Exclude NaN Values in Mean Calculation:
- Error: Calculating the mean without excluding NaN values can lead to inaccurate results.
- Handling: Use the
skipna
parameter in themean()
method to exclude NaN values.
mean = df.mean(skipna=True)
- Not Checking for All-NaN Columns:
- Error: If an entire column consists of NaN values, replacing them with the mean would not provide any meaningful information.
- Handling: Check for columns where all values are NaN before applying the replacement.
nan_columns = df.columns[df.isna().all()]
df.drop(columns=nan_columns, inplace=True)
- Consideration for Categorical Data:
- Error: Using this method on categorical data can lead to inappropriate results.
- Handling: For categorical data, consider using alternative methods such as filling NaN with the mode or a placeholder value.
# Replace NaN with mode for a specific column 'Category'
df['Category'].fillna(df['Category'].mode()[0], inplace=True)
By addressing these common errors and considering the pros and cons, you can enhance the robustness of your data imputation process when dealing with missing values in a pandas DataFrame.
Conclusion
Replacing NaN values with the average of columns in a pandas DataFrame is a simple yet effective way to handle missing values. In this blog post, we used the fillna()
method to replace NaN values and the mean()
method to calculate the mean of each column. By following these steps, you can ensure that your analysis or machine learning models are based on complete and accurate data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.