How to Replace NaN Values with Mean Values in Pandas for a Given Grouping
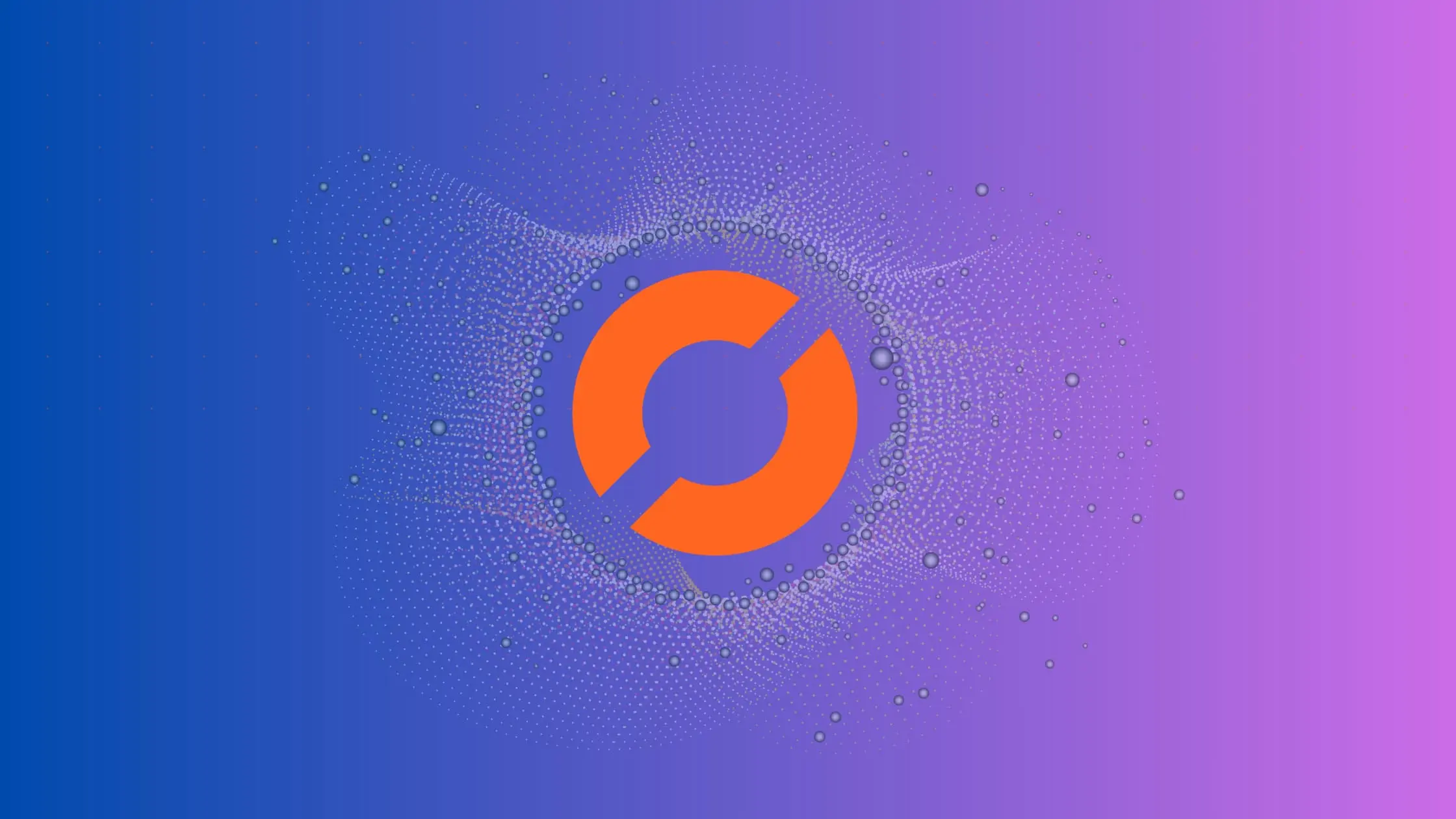
As a data scientist or software engineer, you may encounter datasets with missing values or NaN values. These missing values can negatively affect the accuracy of your analysis or machine learning models. To mitigate this, you can replace the NaN values with mean values, which can give you a better understanding of the data.
In this article, I will show you how to use Pandas, a popular data manipulation library, to replace NaN values with mean values for a given grouping. This technique is particularly useful when dealing with large datasets with missing values.
What is Pandas?
Pandas is an open-source Python library used for data manipulation and analysis. It provides a powerful data structure called a DataFrame, which allows you to store and manipulate tabular data in a flexible and efficient way. Pandas also provides a wide range of functions for data cleaning, preparation, and analysis.
What are NaN Values?
NaN stands for “Not a Number,” and it is a common placeholder used to represent missing or undefined values in a dataset. NaN values can be caused by a variety of factors, such as human error, sensor malfunction, or data corruption. NaN values can also be introduced during data cleaning or preparation.
How to Replace NaN Values with Mean Values in Pandas for a Given Grouping?
To replace NaN values with mean values in Pandas, we can use the fillna()
and groupby()
functions. The fillna()
function is used to replace NaN values with specified values, while the groupby()
function is used to group rows based on a specific column or columns.
Here’s a step-by-step guide on how to replace NaN values with mean values for a given grouping in Pandas:
Step 1: Import the Required Libraries
To use Pandas, we need to import it first. Additionally, we will also import NumPy, another popular Python library used for numerical operations.
import pandas as pd
import numpy as np
Step 2: Load the Dataset
Next, we need to load the dataset into a Pandas DataFrame. For this example, we will use a sample dataset containing information about cars and their fuel consumption. The dataset contains missing values in the “mpg” column, which we will replace with mean values.
df = pd.read_csv('cars.csv')
Step 3: Group the Data by a Specific Column
Now, we will group the data by a specific column. In this example, we will group the data by the “cylinders” column, which contains information about the number of cylinders in each car.
grouped = df.groupby(['cylinders'])
Step 4: Calculate the Mean Value for Each Group
Next, we will calculate the mean value for each group using the mean()
function.
mean_values = grouped.mean()
Step 5: Replace NaN Values with Mean Values
Finally, we will replace the NaN values in the “mpg” column with the mean values of each group using the transform method. This will preserve the original structure of the DataFrame.
df['mpg'] = grouped['mpg'].transform(lambda x: x.fillna(x.mean()))
Step 6: Check the Results
To verify that the NaN values have been replaced with mean values, we can print the first few rows of the updated DataFrame.
print(df.head())
The output should look like this:
cylinders mpg displacement horsepower weight acceleration model_year origin car_name
0 8 18.0 307.0 130.0 3504.0 12.0 70 1 chevrolet chevelle malibu
1 8 15.0 350.0 165.0 3693.0 11.5 70 1 buick skylark 320
2 8 18.0 318.0 150.0 3436.0 11.0 70 1 plymouth satellite
3 8 16.0 304.0 150.0 3433.0 12.0 70 1 amc rebel sst
4 8 14.0 454.0 220.0 4354.0 9.0 70 1 ford torino
As you can see, the NaN values in the “mpg” column have been replaced with mean values for each group.
Conclusion
Replacing NaN values with mean values for a given grouping is a useful technique in data cleaning and preparation. With Pandas, it is easy to group data by a specific column and calculate mean values for each group. By replacing NaN values with mean values, you can improve the accuracy of your analysis or machine learning models.
I hope this article has helped you understand how to replace NaN values with mean values for a given grouping in Pandas. If you have any questions or comments, please feel free to leave them below.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.