How to Replace Multiple Values in One Column using Pandas
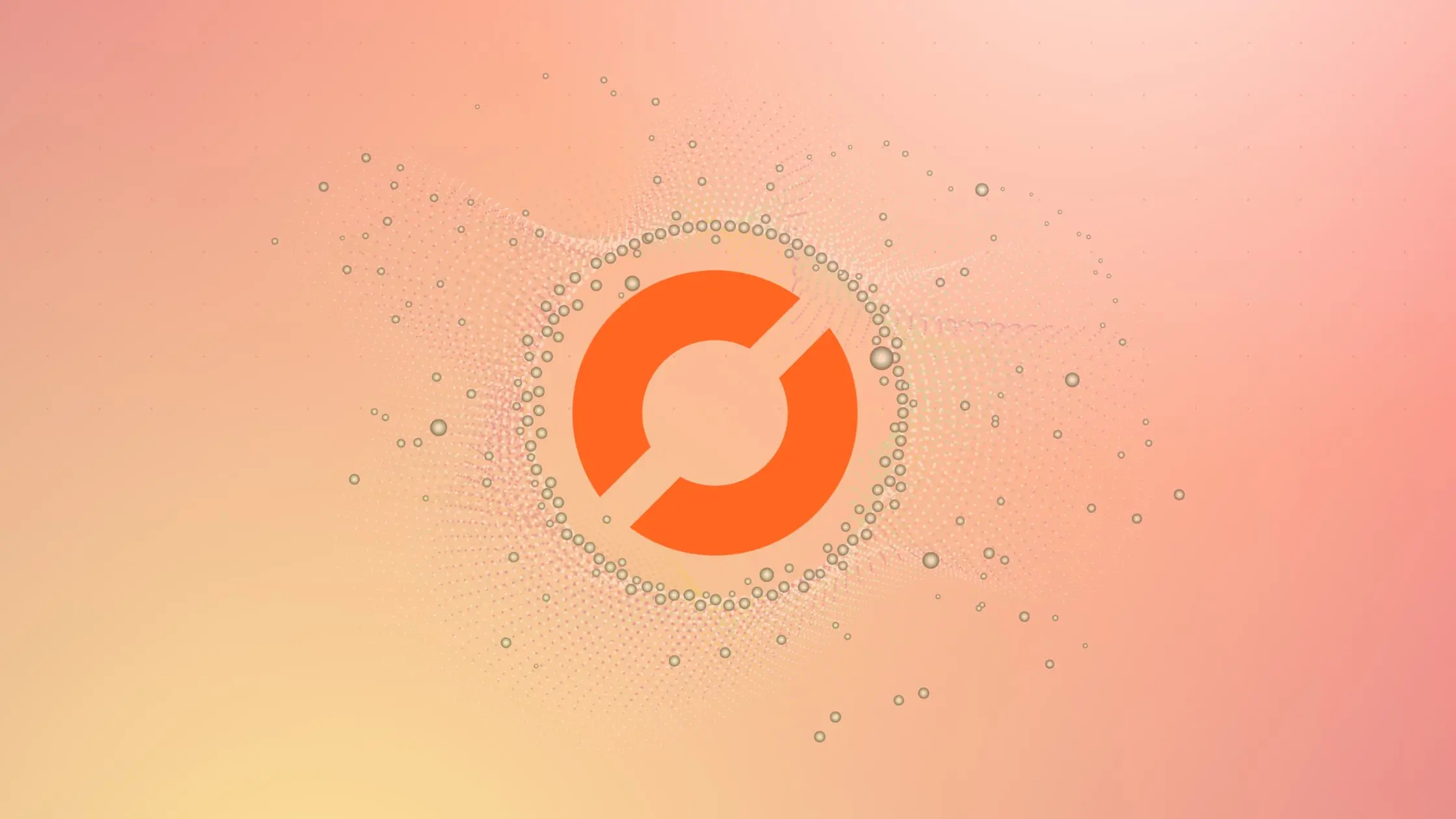
Table of Contents
- What is Pandas?
- How to Replace Multiple Values in One Column using Pandas
- Handling Common Errors
- Conclusion
As a data scientist or software engineer, you may often come across a situation where you need to replace multiple values in a single column of a Pandas DataFrame. This can be a tedious and time-consuming task if done manually. Fortunately, Pandas provides a simple and efficient way to replace multiple values in one column using the replace()
method.
In this article, we will explore how to use the replace()
method to replace multiple values in one column of a Pandas DataFrame.
What is Pandas?
Pandas is a popular Python library used for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, as well as a wide range of tools for data cleaning, transformation, and analysis.
How to Replace Multiple Values in One Column using Pandas
To replace multiple values in one column of a Pandas DataFrame, we can use the replace()
method. The replace()
method takes two arguments:
to_replace
: the value(s) to be replacedvalue
: the value(s) to replaceto_replace
with
Here is an example of how to use the replace()
method to replace multiple values in a single column:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'col1': ['apple', 'banana', 'orange', 'pear', 'apple', 'banana', 'orange', 'pear'],
'col2': [1, 2, 3, 4, 5, 6, 7, 8]})
# Replace multiple values in col1
df['col1'].replace(['apple', 'banana'], 'fruit', inplace=True)
print(df)
Output:
col1 col2
0 fruit 1
1 fruit 2
2 orange 3
3 pear 4
4 fruit 5
5 fruit 6
6 orange 7
7 pear 8
In this example, we created a sample DataFrame with two columns (col1
and col2
). We then used the replace()
method to replace the values apple
and banana
in col1
with the value fruit
.
Note that we used the inplace=True
argument to modify the DataFrame in place. If you omit this argument, Pandas will return a new DataFrame with the replaced values.
Replacing Multiple Values with Different Values
In some cases, we may want to replace multiple values in one column with different values. We can still use the replace()
method for this task by passing a dictionary as the value
argument.
Here is an example of how to use the replace()
method to replace multiple values in a single column with different values:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'col1': ['apple', 'banana', 'orange', 'pear', 'apple', 'banana', 'orange', 'pear'],
'col2': [1, 2, 3, 4, 5, 6, 7, 8]})
# Replace multiple values in col1 with different values
df['col1'].replace({'apple': 'fruit', 'banana': 'fruit', 'orange': 'citrus'}, inplace=True)
print(df)
Output:
col1 col2
0 fruit 1
1 fruit 2
2 citrus 3
3 pear 4
4 fruit 5
5 fruit 6
6 citrus 7
7 pear 8
In this example, we used a dictionary as the value
argument to replace the values apple
and banana
with the value fruit
, and the value orange
with the value citrus
.
Handling Common Errors
Missing Values
Handling missing values before replacement is crucial to avoid unexpected behavior. Depending on the use case, you can either fill or drop missing values.
import pandas as pd
# Create a sample DataFrame
data = {'Column1': [1, 2, 3, 4, 5, 6],
'Column2': ['A', 'B', 'C', 'D', 'A', 'C']}
df = pd.DataFrame(data)
# Introduce missing values
df.loc[2, 'Column2'] = None
# Attempt to replace values with missing values present
replacement_dict = {'A': 'X', 'B': 'Y', 'C': 'Z'}
df['Column3'] = df['Column2'].replace(replacement_dict)
# Display the DataFrame to identify the issue
print(df)
Output:
Column1 Column2 Column3
0 1 A X
1 2 B Y
2 3 None None
3 4 D D
4 5 A X
5 6 C Z
Handling:
Use dropna()
to remove rows with missing values if they are not relevant to your analysis.
# Drop rows with missing values before replacement
df = df.dropna(subset=['Column2']).replace(replacement_dict)
Output:
Column1 Column2 Column3
0 1 X X
1 2 Y Y
3 4 D D
4 5 X X
5 6 Z Z
Conclusion
In this article, we explored how to replace multiple values in one column of a Pandas DataFrame using the replace()
method. We also learned how to replace multiple values with different values using a dictionary.
The replace()
method is a powerful tool for data manipulation and can save a lot of time and effort when dealing with large datasets. By using this method, you can easily replace multiple values in one column of a Pandas DataFrame with just a few lines of code.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.