How to Replace Column Values in a Pandas DataFrame
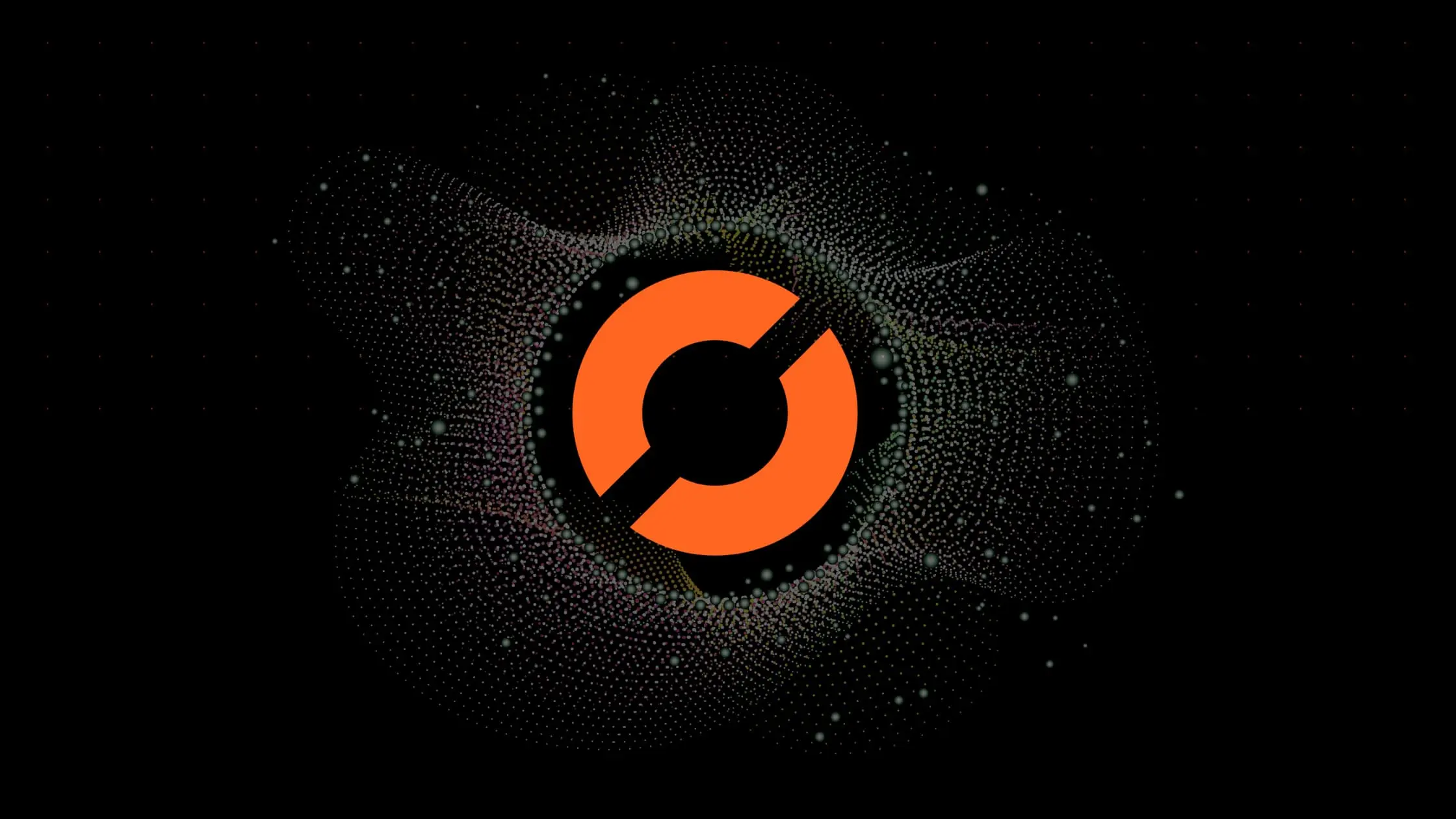
How to Replace Column Values in a Pandas DataFrame
As a data scientist or software engineer, you may often find yourself working with large datasets that require cleaning and transformation. One common task is replacing column values in a Pandas DataFrame. In this article, we will explore different methods for replacing column values in a Pandas DataFrame, and discuss the advantages and disadvantages of each approach.
What is a Pandas DataFrame?
Pandas is a powerful data manipulation library for Python. A Pandas DataFrame is a two-dimensional table-like data structure that contains rows and columns. It is similar to a spreadsheet or a SQL table, but with more functionality. DataFrames are widely used in data science and machine learning, and they provide a convenient way to manipulate and analyze data.
Why replace column values in a Pandas DataFrame?
Data cleaning is an essential step in the data analysis process. In many cases, datasets contain missing or incorrect values that can affect the accuracy of your analysis. Replacing column values is one way to clean your data and ensure that it is accurate and reliable. For example, you may want to replace missing values with a default value, or replace incorrect values with the correct ones.
How to replace column values in a Pandas DataFrame
There are several ways to replace column values in a Pandas DataFrame. The method you choose depends on the specific task you are trying to accomplish, and the structure of your data. Here are some of the most common methods:
Method 1: Using the .replace() method
The .replace() method is a simple way to replace column values in a Pandas DataFrame. This method takes two arguments: the value you want to replace, and the new value you want to replace it with. Here is an example:
import pandas as pd
# create a DataFrame
df = pd.DataFrame({'A': ['foo', 'bar', 'baz'], 'B': [1, 2, 3]})
# replace 'foo' with 'qux'
df['A'].replace('foo', 'qux', inplace=True)
# print the DataFrame
print(df)
Output:
A B
0 qux 1
1 bar 2
2 baz 3
In this example, we created a DataFrame with two columns (‘A’ and ‘B’). We then used the .replace() method to replace the value ‘foo’ in column ‘A’ with the value ‘qux’. The inplace=True parameter tells Pandas to modify the DataFrame in place, rather than creating a new copy.
Method 2: Using Boolean indexing
Boolean indexing is another way to replace column values in a Pandas DataFrame. This method involves creating a Boolean mask that indicates which values to replace, and then using this mask to replace the values. Here is an example:
import pandas as pd
# create a DataFrame
df = pd.DataFrame({'A': ['foo', 'bar', 'baz'], 'B': [1, 2, 3]})
# create a Boolean mask
mask = df['A'] == 'foo'
# replace values based on the mask
df.loc[mask, 'A'] = 'qux'
# print the DataFrame
print(df)
Output:
A B
0 qux 1
1 bar 2
2 baz 3
In this example, we created a Boolean mask that is True for all rows where column ‘A’ equals ‘foo’. We then used this mask to replace the corresponding values in column ‘A’ with ‘qux’.
Method 3: Using the .map() method
The .map() method is a powerful way to replace column values in a Pandas DataFrame. This method takes a dictionary as an argument, where the keys represent the values to be replaced, and the values represent the new values. Here is an example:
import pandas as pd
# create a DataFrame
df = pd.DataFrame({'A': ['foo', 'bar', 'baz'], 'B': [1, 2, 3]})
# create a dictionary of replacements
replacements = {'foo': 'qux', 'baz': 'quux'}
# replace values using the .map() method
df['A'] = df['A'].map(replacements).fillna(df['A'])
# print the DataFrame
print(df)
Output:
A B
0 qux 1
1 bar 2
2 quux 3
In this example, we created a dictionary of replacements, where the keys are the values to be replaced, and the values are the new values. We then used the .map() method to apply the replacements to column ‘A’. The .fillna() method is used to fill in any missing values with the original values.
Conclusion
Replacing column values in a Pandas DataFrame is an important task in data cleaning and transformation. There are several methods for replacing column values, each with its own advantages and disadvantages. In this article, we explored three common methods: using the .replace() method, using Boolean indexing, and using the .map() method. By understanding these methods, you can choose the best approach for your specific task and data structure. Happy data cleaning!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.