How to Replace All Values in a Pandas DataFrame Column Based on a Condition
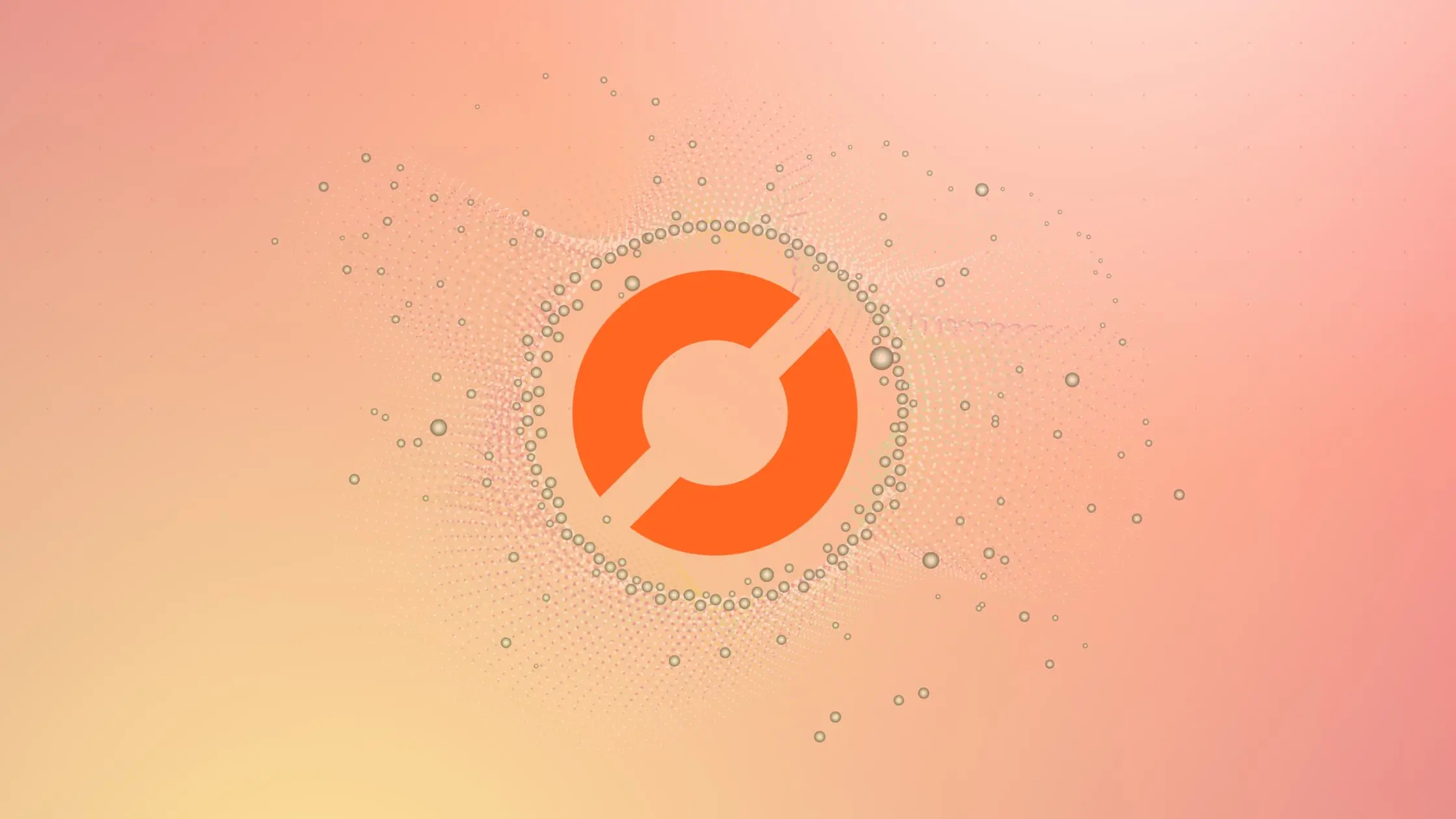
As a data scientist or software engineer, you may come across a situation where you need to replace all values in a Pandas DataFrame column based on a certain condition. This can be easily achieved using the powerful DataFrame capabilities of Pandas library in Python.
In this article, we will explore the step-by-step process of replacing column values in a Pandas DataFrame based on a condition. We will also provide some examples to help you understand the process better.
Prerequisites
- Basic knowledge of Python programming language
- Familiarity with Pandas library and its DataFrame object
Step 1: Import Required Libraries
Before we start working with Pandas DataFrame, we need to import the required libraries. In this case, we need to import the Pandas library.
import pandas as pd
Step 2: Create a Sample DataFrame
We will create a sample DataFrame to demonstrate how to replace column values based on a condition. The sample DataFrame will have two columns: “name” and “age”.
# create a sample DataFrame
data = {'name': ['Alice', 'Bob', 'Charlie', 'David', 'Eva'],
'age': [25, 35, 45, 55, 65]}
df = pd.DataFrame(data)
Step 3: Replace Column Values Based on Condition
To replace column values based on a condition, we can use the loc
method of Pandas DataFrame. The loc
method allows us to select rows and columns based on labels or boolean arrays.
In this example, we will replace all values in the “age” column that are greater than or equal to 50 with the value 50.
# replace values in the 'age' column based on condition
df.loc[df['age'] >= 50, 'age'] = 50
The above code selects all rows where the “age” column value is greater than or equal to 50 and replaces the value with 50.
Step 4: Verify the Result
To verify that the values have been replaced correctly, we can print the DataFrame using the print()
function.
# print the DataFrame
print(df)
Output:
name age
0 Alice 25
1 Bob 35
2 Charlie 45
3 David 50
4 Eva 50
As we can see from the output, all values in the “age” column that were greater than or equal to 50 have been replaced with the value 50.
Pros and Cons of Condition-Based Value Replacement in Pandas DataFrame:
Pros:
- Simplicity and Readability: The method for replacing column values based on a condition using the Pandas library is concise and easy to understand.
- Flexibility: The loc method provides a flexible way to select specific rows and columns based on conditions, allowing for various replacement scenarios.
- Integration with DataFrame Operations: The approach seamlessly integrates with other DataFrame operations, making it part of a comprehensive data manipulation workflow.
- Applicability: This method is applicable to a wide range of scenarios, providing a general solution for condition-based value replacement in DataFrame columns.
Cons:
- In-Place Modification: The method modifies the DataFrame in place. While this can be efficient, it might not be suitable if you want to keep the original DataFrame unchanged.
- Limited to Label-Based Selection: The loc method primarily relies on label-based selection, which may not cover all scenarios, especially if more complex conditions or index-based selection is needed.
- Potential for Overwriting Data: Care must be taken when specifying conditions to avoid unintentionally replacing values that should be retained.
Common Errors and How to Handle:
- Unintended Overwriting:
- Error: Specifying a broad condition might unintentionally overwrite more values than intended.
- Handling: Carefully craft conditions to ensure that only the desired values are replaced. Test on a subset of data if necessary.
- Mismatched Data Types:
- Error: If the replacement value has a different data type than the original column, it can lead to unexpected results or errors.
- Handling: Ensure that the replacement value has a compatible data type with the column being modified.
- Complex Conditions:
- Error: Trying to implement complex conditions may lead to syntax errors or unexpected behavior.
- Handling: Break down complex conditions into simpler steps or use additional boolean arrays to construct the final condition.
Conclusion
In this article, we have demonstrated how to replace all values in a Pandas DataFrame column based on a condition. We used the loc
method of Pandas DataFrame to select rows and columns based on labels or boolean arrays and replaced the values in the selected rows based on the condition.
You can use this technique to replace column values in any Pandas DataFrame based on a condition that meets your specific requirements.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.