How to Rename Column and Index with Pandas
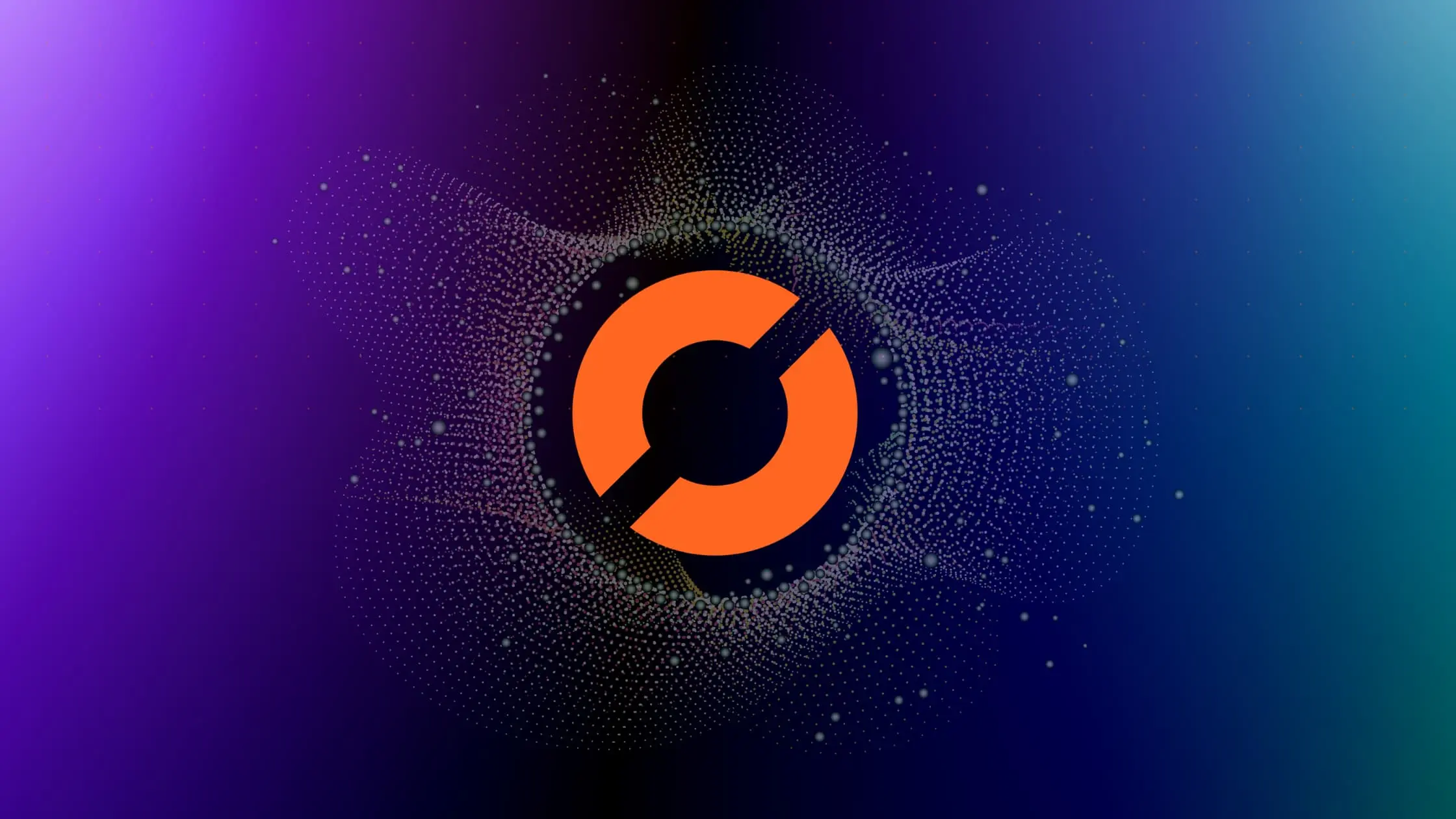
How to Rename Column and Index with Pandas
Pandas is a popular data analysis library in Python. It provides powerful tools for data manipulation and analysis. One of the essential features of Pandas is the ability to rename columns and indexes of a DataFrame. In this tutorial, we will learn how to rename columns and indexes with Pandas.
Renaming Columns
Renaming columns in Pandas is a straightforward process. We can use the rename()
method to rename one or more columns of a DataFrame. The rename()
method takes a dictionary as an argument, where the keys are the old column names, and the values are the new column names.
Let’s take an example DataFrame:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
})
print(df)
Output:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
Suppose we want to rename column A
to X
and column B
to Y
. We can do it as follows:
df = df.rename(columns={'A': 'X', 'B': 'Y'})
print(df)
Output:
X Y C
0 1 4 7
1 2 5 8
2 3 6 9
As we can see, the columns A
and B
have been renamed to X
and Y
, respectively.
Renaming Indexes
Renaming indexes in Pandas is similar to renaming columns. We can use the rename()
method to rename the index of a DataFrame. The rename()
method takes a dictionary as an argument, where the key is the old index name, and the value is the new index name.
Let’s take an example DataFrame:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}, index=['X', 'Y', 'Z'])
print(df)
Output:
A B C
X 1 4 7
Y 2 5 8
Z 3 6 9
Suppose we want to rename the index X
to W
. We can do it as follows:
df = df.rename(index={'X': 'W'})
print(df)
Output:
A B C
W 1 4 7
Y 2 5 8
Z 3 6 9
As we can see, the index X
has been renamed to W
.
Renaming Columns and Indexes in Place
By default, the rename()
method returns a new DataFrame with the renamed columns and indexes. However, we can also rename columns and indexes in place by setting the inplace
parameter to True
.
Let’s take an example DataFrame:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}, index=['X', 'Y', 'Z'])
print(df)
Output:
A B C
X 1 4 7
Y 2 5 8
Z 3 6 9
Suppose we want to rename column A
to X
and index X
to W
in place. We can do it as follows:
df.rename(columns={'A': 'X'}, index={'X': 'W'}, inplace=True)
print(df)
Output:
X B C
W 1 4 7
Y 2 5 8
Z 3 6 9
As we can see, the column A
has been renamed to X
, and the index X
has been renamed to W
in place.
Conclusion
Renaming columns and indexes is a common operation in data analysis. Pandas provides a simple and powerful way to rename columns and indexes with the rename()
method. We can rename one or more columns and indexes by passing a dictionary as an argument, where the keys are the old names, and the values are the new names. We can also rename columns and indexes in place by setting the inplace
parameter to True
.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.