How to Remove Rows with Specific Values in Pandas DataFrame
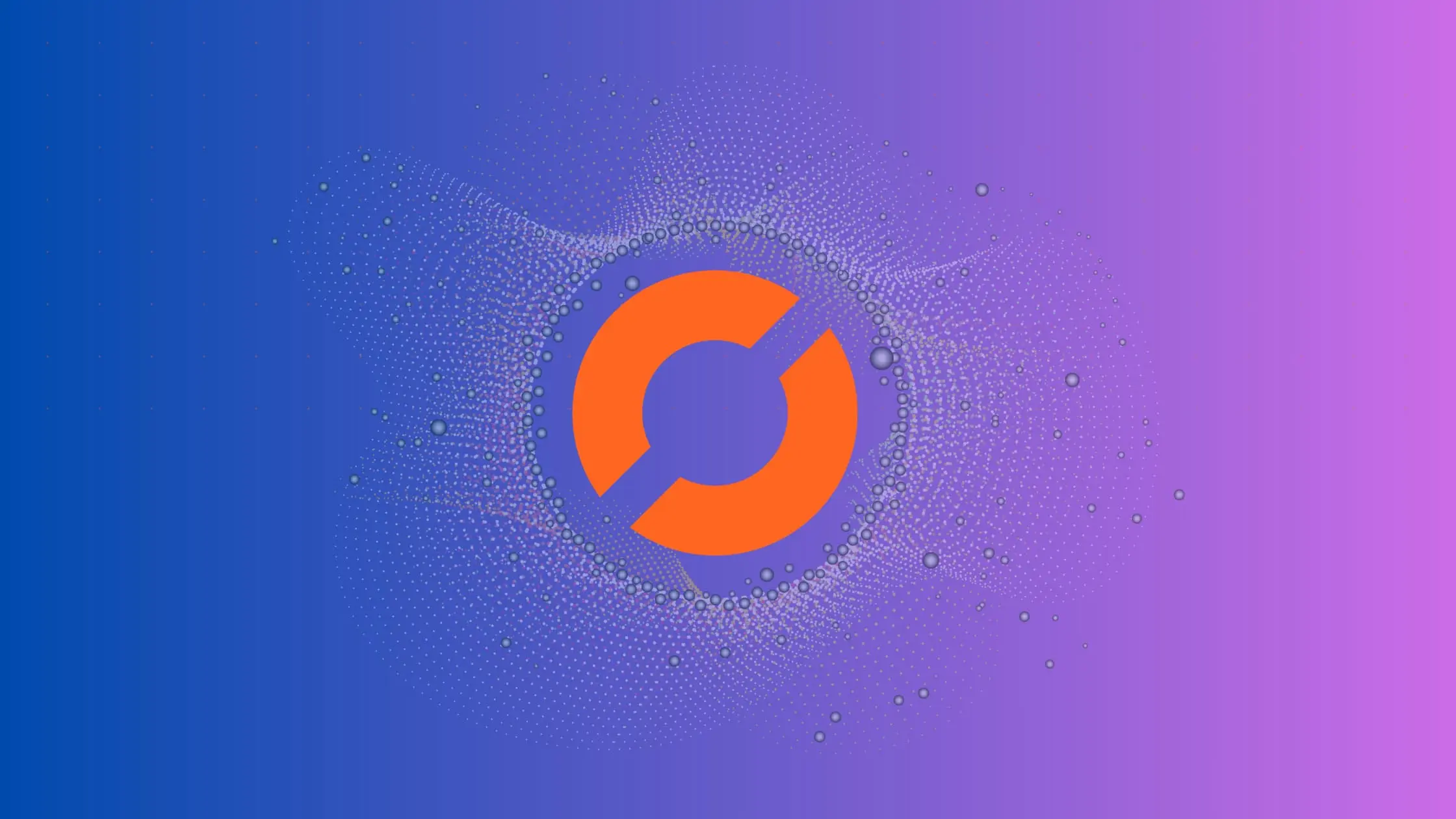
How to Remove Rows with Specific Values in Pandas DataFrame
As a data scientist or software engineer, working with datasets is a common task. Often, we need to clean and preprocess our data before we can start with the actual analysis. One common task that we might need to do is to remove rows with specific values in a Pandas DataFrame. In this article, we will explore how to achieve this task in a simple and efficient way.
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional, size-mutable, tabular data structure with columns of potentially different types. It is similar to a spreadsheet or a SQL table, but with more powerful features and functionality. Pandas is a popular data manipulation library in Python, widely used in data science and machine learning.
The Problem
Suppose we have a dataset with multiple columns, and we want to remove rows that contain a specific value in a particular column. For example, let’s consider a dataset of customer orders with columns order_id
, customer_id
, product_name
, and quantity
. Suppose we want to remove all rows where the product name is ‘Coca Cola’.
We can achieve this task in multiple ways, but some methods are more efficient than others. In this article, we will explore two methods to remove rows with specific values in a Pandas DataFrame.
Method 1: Using Boolean Indexing
Boolean indexing is a powerful technique in Pandas that allows us to filter a DataFrame based on a Boolean condition. We can use this technique to create a Boolean mask that indicates which rows contain the specific value we want to remove. Then, we can use this mask to select all rows except the ones that contain the specific value.
Here’s how we can implement this method:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({
'order_id': [1, 2, 3, 4, 5],
'customer_id': [101, 102, 103, 104, 105],
'product_name': ['Coca Cola', 'Pepsi', 'Fanta', 'Sprite', 'Pepsi'],
'quantity': [2, 1, 3, 2, 2]
})
print(df)
Output:
order_id customer_id product_name quantity
0 1 101 Coca Cola 1
1 2 102 Pepsi 1
2 3 103 Fanta 3
3 4 104 Sprite 2
4 5 105 Pepsi 2
# create a Boolean mask for the rows to remove
mask = df['product_name'] == 'Coca Cola'
# select all rows except the ones that contain 'Coca Cola'
df = df[~mask]
# print the resulting DataFrame
print(df)
Output:
order_id customer_id product_name quantity
1 2 102 Pepsi 1
2 3 103 Fanta 3
3 4 104 Sprite 2
4 5 105 Pepsi 2
In the code above, we first create a Boolean mask that indicates which rows contain the value Coca Cola
in the product_name
column. We use this mask to select all rows except the ones that contain Coca Cola
, using the negation operator ~
. Finally, we assign the result back to the original DataFrame.
This method is simple and efficient, especially for large datasets. However, it requires creating a Boolean mask, which can be memory-intensive for very large datasets.
Method 2: Using the drop Function
Another method to remove rows with specific values in a Pandas DataFrame is to use the drop
function. This function allows us to remove rows or columns based on their labels or positions. We can use this function to remove all rows that contain the specific value we want to remove.
Here’s how we can implement this method:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({
'order_id': [1, 2, 3, 4, 5],
'customer_id': [101, 102, 103, 104, 105],
'product_name': ['Coca Cola', 'Pepsi', 'Fanta', 'Sprite', 'Pepsi'],
'quantity': [2, 1, 3, 2, 2]
})
# drop all rows that contain 'Coca Cola'
df = df.drop(df[df['product_name'] == 'Coca Cola'].index)
# print the resulting DataFrame
print(df)
Output:
order_id customer_id product_name quantity
1 2 102 Pepsi 1
2 3 103 Fanta 3
3 4 104 Sprite 2
4 5 105 Pepsi 2
In the code above, we first select all rows that contain Coca Cola
using the Boolean indexing technique. Then, we use the drop
function to remove all these rows based on their index. Finally, we assign the result back to the original DataFrame.
This method is also simple and efficient, especially for large datasets. However, it requires selecting the rows to remove twice, which can be time-consuming for very large datasets.
Conclusion
In this article, we explored two methods to remove rows with specific values in a Pandas DataFrame. The first method uses Boolean indexing, which is simple and efficient, especially for large datasets. The second method uses the drop
function, which is also simple and efficient, but requires selecting the rows to remove twice.
Both methods are valid and can be used depending on the specific requirements and constraints of the task at hand. As a data scientist or software engineer, it’s important to be familiar with these techniques and to choose the most appropriate one for each situation.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.