How to Remove Rows from Pandas Data Frame that Contains any String in a Particular Column
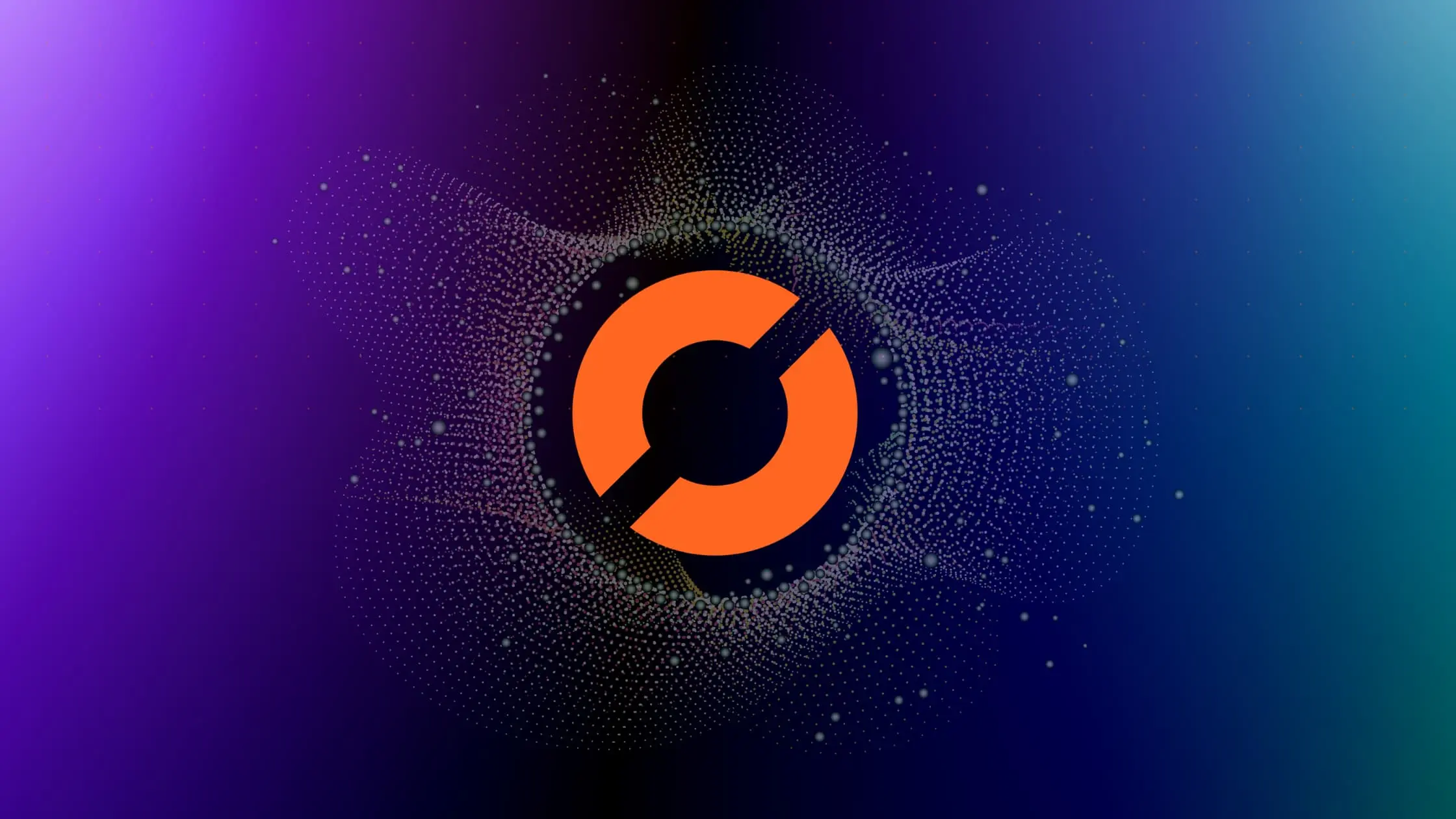
As a data scientist or a software engineer, dealing with data is a crucial part of our work. Pandas is one of the most popular Python libraries for working with data frames. It provides various functions and methods to manipulate data frames, including removing rows that contain a particular string in a specific column.
In this article, we will explore how to remove rows from a pandas data frame that contain any string in a particular column. We will start by understanding the problem statement and then move on to the solution.
Problem Statement
Suppose we have a pandas data frame with multiple columns, and we want to remove rows that contain any string in a particular column. For instance, consider the following data frame:
Name | Age | Gender | |
---|---|---|---|
0 | John Doe | 25 | Male |
1 | Jane Doe | 30 | Female |
2 | Mark Johnson | 40 | Male |
3 | Sarah Smith | 20 | Female |
4 | Adam Smith | 35 | Male |
Suppose we want to remove all the rows that contain the string “Smith” in the “Name” column. In that case, we need to remove rows 3 and 4 from the data frame.
Solution 1: Using str.contains()
To remove rows from a pandas data frame that contains any string in a particular column, we can use the str.contains()
method. This method returns a Boolean series that indicates whether each string contains a specified substring or not.
We can use this Boolean series to filter the rows that contain the specified substring and then remove those rows from the original data frame. Here’s how we can do it:
import pandas as pd
# create a sample data frame
df = pd.DataFrame({'Name': ['John Doe', 'Jane Doe', 'Mark Johnson', 'Sarah Smith', 'Adam Smith'],
'Age': [25, 30, 40, 20, 35],
'Gender': ['Male', 'Female', 'Male', 'Female', 'Male']})
# display the original data frame
print("Original Data Frame:")
print(df)
# filter the rows that contain the substring
substring = 'Smith'
filter = df['Name'].str.contains(substring)
filtered_df = df[~filter]
# display the filtered data frame
print(f"\nData Frame after removing rows that contain '{substring}' in 'Name' column:")
print(filtered_df)
Output:
Original Data Frame:
Name Age Gender
0 John Doe 25 Male
1 Jane Doe 30 Female
2 Mark Johnson 40 Male
3 Sarah Smith 20 Female
4 Adam Smith 35 Male
Data Frame after removing rows that contain 'Smith' in 'Name' column:
Name Age Gender
0 John Doe 25 Male
1 Jane Doe 30 Female
2 Mark Johnson 40 Male
In the above code, we first create a sample data frame with three columns: “Name”, “Age”, and “Gender”. Then, we display the original data frame using the print()
function.
Next, we define the substring that we want to remove from the “Name” column. Here, we have used the string “Smith”. We then use the str.contains()
method to filter the rows that contain the substring in the “Name” column. The ~
operator is used to reverse the Boolean series so that we get the rows that do not contain the specified substring.
Solution 2: Using query
method
Another approach to remove rows is by using the query
method of Pandas. This method allows us to filter data frames using a query expression.
# define the substring to be removed
substring = 'Smith'
# use the query method to filter the rows
filtered_df = df.query(f'not Name.str.contains("{substring}")', engine='python')
# display the filtered data frame
print(f"\nData Frame after removing rows that contain '{substring}' in 'Name' column:")
print(filtered_df)
Output:
Original Data Frame:
Name Age Gender
0 John Doe 25 Male
1 Jane Doe 30 Female
2 Mark Johnson 40 Male
3 Sarah Smith 20 Female
4 Adam Smith 35 Male
Data Frame after removing rows that contain 'Smith' in 'Name' column:
Name Age Gender
0 John Doe 25 Male
1 Jane Doe 30 Female
2 Mark Johnson 40 Male
In the above code, we first create a sample data frame with three columns: “Name”, “Age”, and “Gender”. Then, we display the original data frame using the print() function.
Finally, we create a new data frame containing the filtered rows and display it using the print()
function.
Conclusion
In this article, we learned how to remove rows from a pandas DataFrame that contain a specific string in a particular column. We used the str.contains()
method and the query
method to filter the rows containing the specified substring, and then we removed those rows from the original DataFrame.
This is a simple yet powerful technique that can be employed to filter DataFrames based on specific criteria. It can be especially useful when dealing with large datasets where manual filtering can be time-consuming and error-prone.
As a data scientist or software engineer, it is essential to have a good understanding of pandas and its various functions and methods. By mastering these tools, we can become more efficient in our work and deliver better results to our clients and stakeholders.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.