How to Remove Index Column in Pandas When Reading a CSV
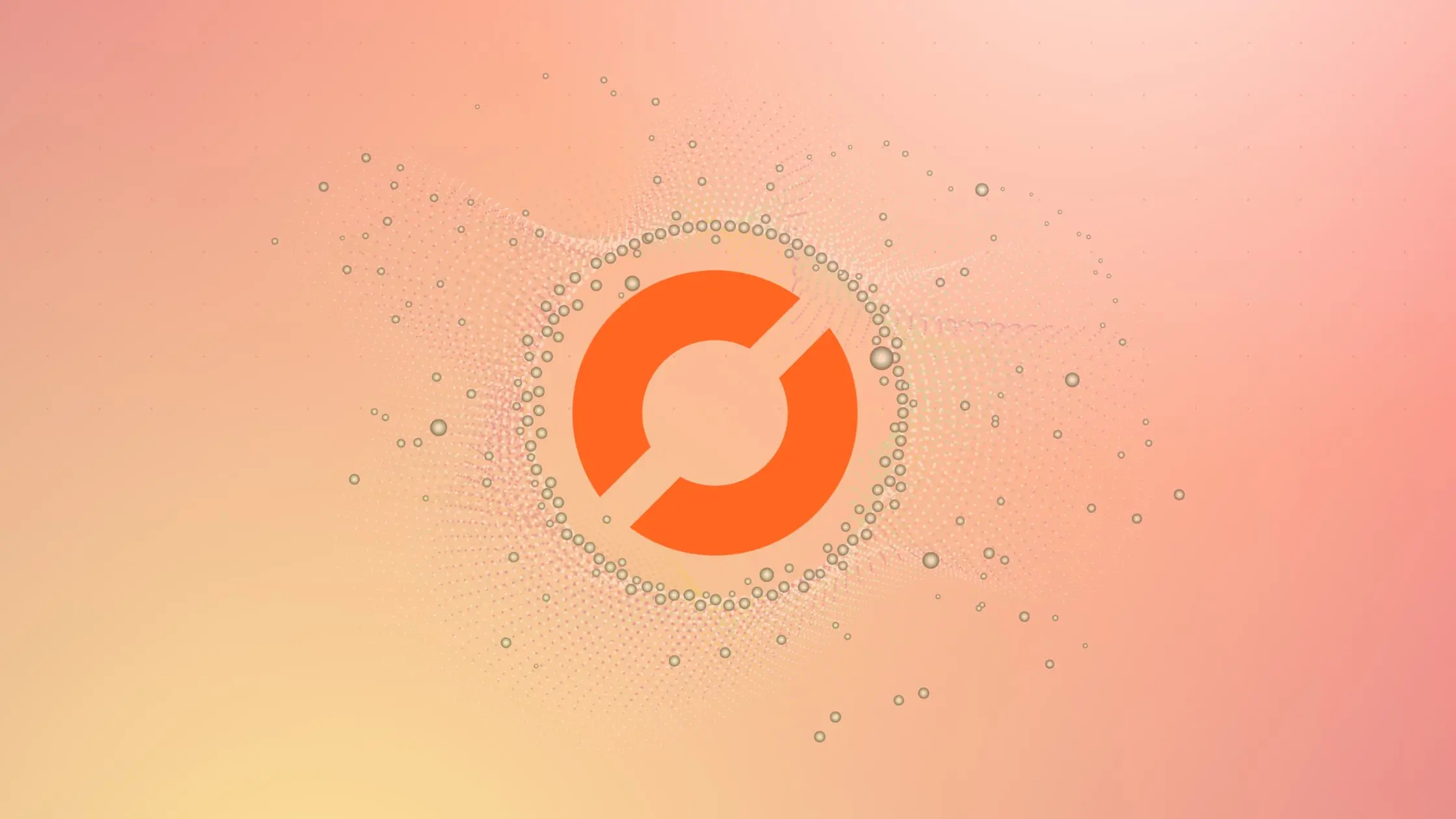
As a data scientist or software engineer, you might have come across a situation where you need to read a CSV file into a Pandas DataFrame but the index column is being included as an extra column. This can be an issue if you want to use the index column as the actual index for the DataFrame. In this blog post, we will discuss how to remove the index column in Pandas when reading a CSV file.
Table of Contents
Problem
By default, when you read a CSV file into a Pandas DataFrame using the read_csv()
function, Pandas assigns an index to each row. This index is displayed as an extra column in the DataFrame. This can be problematic if you want to use the index column as the actual index for the DataFrame.
For example, let’s say you have a CSV file named data.csv
with the following data:
id,name,age
1,John,25
2,Jane,30
3,Bob,40
If you read this CSV file into a Pandas DataFrame using the read_csv()
function, the resulting DataFrame will look like this:
Unnamed: 0 id name age
0 0 1 John 25
1 1 2 Jane 30
2 2 3 Bob 40
As you can see, there is an extra column named Unnamed: 0
, which represents the index column. This can be problematic if you want to use the id
column as the actual index for the DataFrame.
Solution
To remove the index column when reading a CSV file into a Pandas DataFrame, you can use the index_col
parameter of the read_csv()
function. This parameter specifies which column to use as the index for the DataFrame. If you set this parameter to the column index (starting from 0) of the column you want to use as the index, Pandas will not assign an extra index column to the DataFrame.
For example, to use the id
column as the index for the DataFrame, you can set the index_col
parameter to 0
(since id
is the first column in the CSV file):
import pandas as pd
df = pd.read_csv('data.csv', index_col=0)
print(df)
This will result in the following DataFrame:
name age
id
1 John 25
2 Jane 30
3 Bob 40
As you can see, the id
column is now the actual index for the DataFrame, and there is no extra index column.
Other Alternatives
import pandas as pd
# Read the CSV file without setting the index_col parameter
df = pd.read_csv('data.csv')
# Set the desired column as the index after reading the CSV file
df.set_index('id', inplace=True)
print(df)
This will result in the following DataFrame:
name age
id
1 John 25
2 Jane 30
3 Bob 40
This method provides flexibility in cases where the index column is not the first column or when dealing with multiple columns that need to be part of the index. It allows you to read the CSV file as-is and then customize the index based on your specific requirements.
Best Practices
To avoid any redundant index column in the future when you load a csv file, here are some suggestions:
import pandas as pd
# Assuming you have a DataFrame named df
csv_filename = 'data.csv'
df.to_csv(csv_filename, index=False)
print(f"DataFrame has been successfully saved to {csv_filename} without the index column.")
This version of the code adds an explicit comment indicating the best practice being used. Additionally, it stores the CSV file name in a variable (csv_filename), which can make the code more readable and flexible if you need to reuse the file name. Finally, it prints a message indicating that the DataFrame has been successfully saved without the index column, providing useful feedback in your application.
Conclusion
In this blog post, we discussed how to remove the index column in Pandas when reading a CSV file. By setting the index_col
parameter of the read_csv()
function to the column index of the column you want to use as the index, you can avoid having an extra index column in the resulting DataFrame. This can be useful when working with large datasets where optimizing memory usage is important.
Remember that this solution only works when the CSV file has a single index column. If your CSV file has multiple columns that you want to use as the index, you will need to use a different approach, such as setting the index after reading the CSV file into a DataFrame.
I hope you found this blog post helpful.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.