How to Remove Header Row from Pandas Dataframe
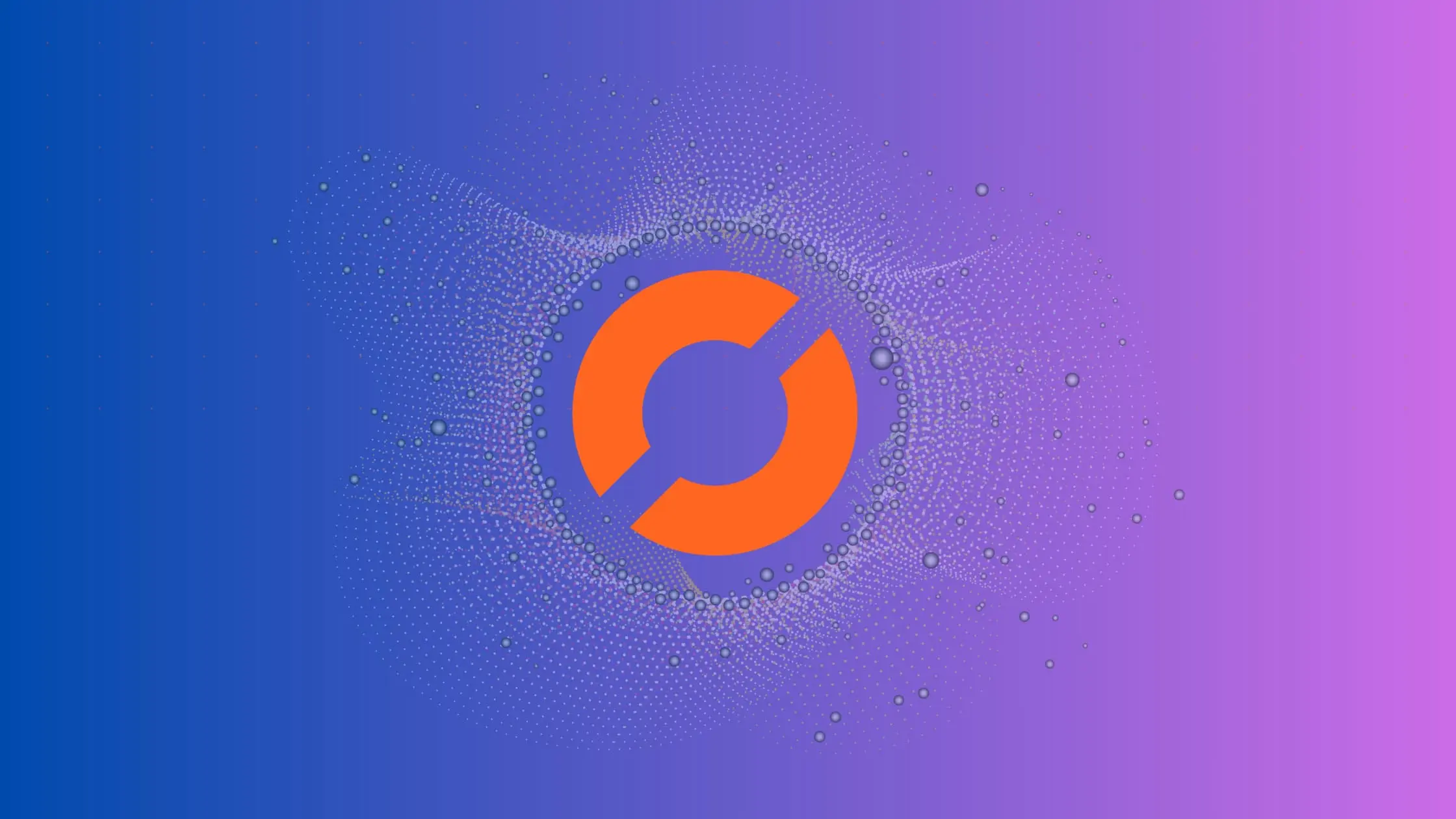
As a data scientist or software engineer, you may have come across a scenario where you need to remove the header row from a pandas dataframe. This can be useful when you are trying to manipulate or analyze data and the header row is not needed. In this article, we will explore how to remove the header row from a pandas dataframe.
Table of Contents
- Introduction
- What is Pandas?
- Why Remove Header Column from Pandas Dataframe?
- How to Remove Header Column from Pandas Dataframe
- Use skiprows Parameter while Loading the Data
- Conclusion
What is Pandas?
Pandas is a widely used open-source data manipulation and analysis library for Python. It provides data structures and functions for working with structured data such as tables, time series, and matrices. Pandas is built on top of the NumPy library and provides easy-to-use interfaces for data manipulation, cleaning, and analysis.
Why Remove Header Column from Pandas Dataframe?
There are several reasons why you may want to remove the header row from a pandas dataframe. Some common reasons include:
- The header row is not needed for analysis or manipulation
- The header row is causing issues with indexing or data manipulation
- The header row is causing issues with data visualization or plotting
How to Remove Header Column from Pandas Dataframe
Removing the header row from a pandas dataframe is a simple process. Here are the steps:
- Load the data into a pandas dataframe
- Check the existing header row using the
.columns
attribute - Remove the header row using the
.iloc
attribute - Reset the index of the dataframe using the
.reset_index()
function
Here is an example code snippet that demonstrates how to remove the header row from a pandas dataframe:
CSV example:
Column1,Column2,Column3
1,Alice,25
2,Bob,30
3,Charlie,22
4,David,35
5,Eve,28
import pandas as pd
# Load the data into a pandas dataframe
df = pd.read_csv('data.csv', header=None)
# Check the existing header row
print(df.columns)
# Remove the header row
df = df.iloc[1:]
# Reset the index of the dataframe
df = df.reset_index(drop=True)
# Check the updated dataframe
print(df.head())
Output:
0 1 2
0 1 Alice 25
1 2 Bob 30
2 3 Charlie 22
3 4 David 35
4 5 Eve 28
In this example, we first load the data into a pandas dataframe using the pd.read_csv()
function. By setting header=None
during the reading process, you inform pandas that there is no header in the data, and it will use default numeric column names. We then check the existing header row using the .columns
attribute. Next, we remove the header row using the .iloc
attribute, which selects all rows except the first row (which contains the header row). Finally, we reset the index of the dataframe using the .reset_index()
function.
Use skiprows parameter while loading the data
Another effective method to remove the header row from a pandas dataframe is by utilizing the skiprows
parameter while loading the data with the pd.read_csv()
function. This parameter allows you to specify the number of rows to skip at the beginning of the file.
Here’s an example code snippet demonstrating how to employ the skiprows
parameter:
# Another method: Skip the header row while loading the data
df_another_method = pd.read_csv('data.csv', skiprows=1, header=None)
Output:
0 1 2
0 1 Alice 25
1 2 Bob 30
2 3 Charlie 22
3 4 David 35
4 5 Eve 28
In this example, setting `skiprows=1`` instructs pandas to skip the first row during the reading process, effectively excluding the header row from the dataframe. This method can be particularly handy if you prefer to handle the removal of the header row directly during the data loading step, streamlining your workflow.
Conclusion
Removing the header row from a pandas dataframe is a simple process that can be useful in many scenarios. By following the steps outlined in this article, you can easily remove the header row and continue with your data manipulation and analysis tasks. Remember to always check your data after removing the header row to ensure that your analysis or visualization is not affected.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.