How to Remove Header Index in Pandas DataFrame
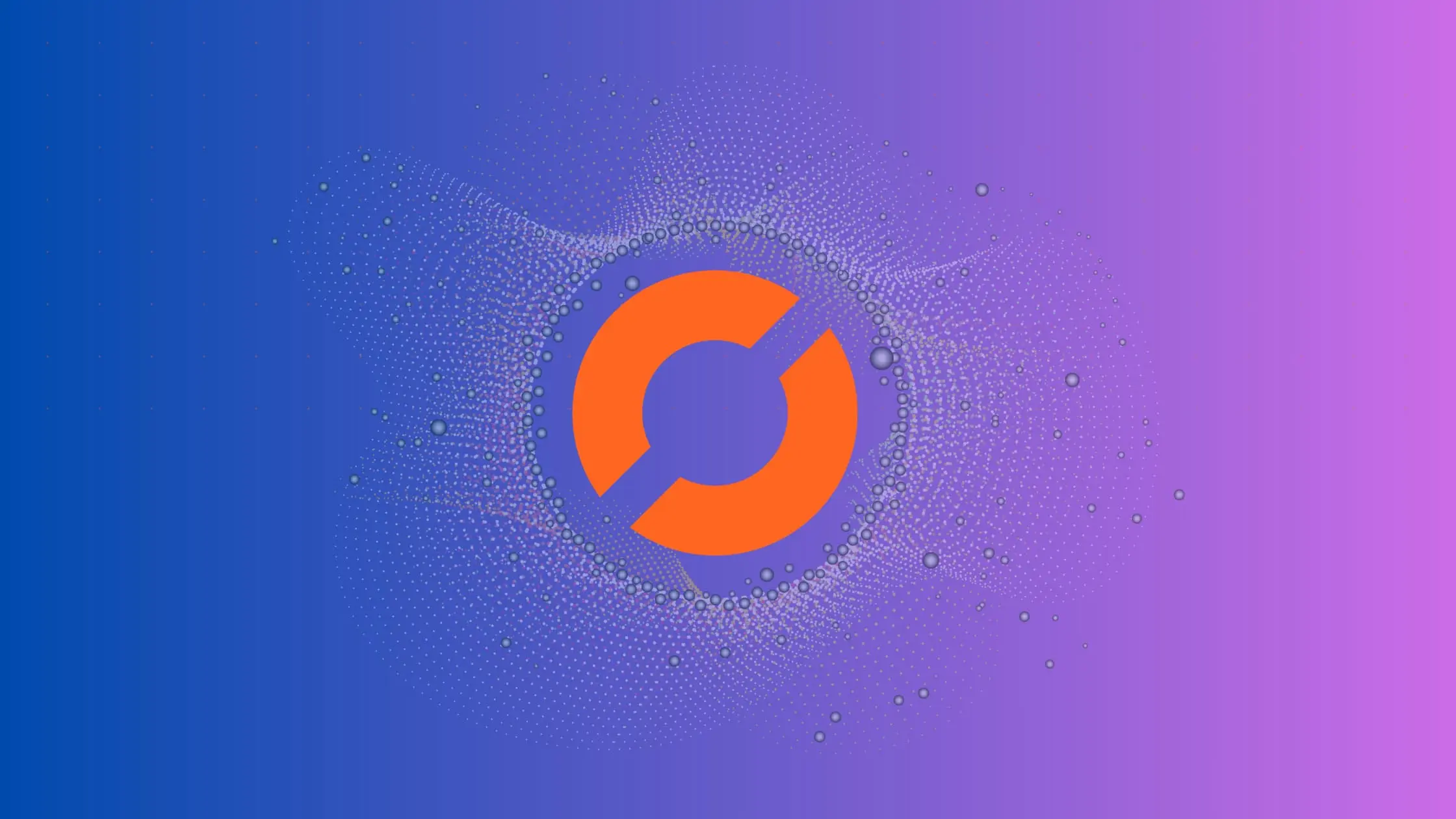
If you’re working with data in Python, chances are you’re using the Pandas library to manipulate and analyze your data. One common issue that data scientists and software engineers may encounter is how to remove the header index in a Pandas DataFrame.
In this tutorial, we’ll walk through the steps to remove the header index in a Pandas DataFrame, and explain why you might want to do this.
Table of Contents
What is a Header Index in Pandas?
In a Pandas DataFrame, the header index is the row of column labels at the top of the DataFrame. By default, Pandas assigns an index to this row, which is numbered starting from 0.
Here’s an example of a DataFrame with a header index:
import pandas as pd
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'salary': [50000, 60000, 70000]
})
print(df)
Output:
name age salary
0 Alice 25 50000
1 Bob 30 60000
2 Charlie 35 70000
In this example, the header index is the row that starts with “name”, “age”, and “salary”. The numbers 0, 1, and 2 indicate the index of each row in the DataFrame.
Why Remove the Header Index?
There are a few reasons why you might want to remove the header index in a Pandas DataFrame:
To make the DataFrame easier to read and manipulate: When you’re working with a large DataFrame, the header index can take up valuable screen space and make it harder to read the data. Removing the header index can make the DataFrame more compact and easier to work with.
To export the DataFrame to a file: When you’re exporting a Pandas DataFrame to a file, you may not want to include the header index in the file. This can make the file more difficult to work with in other programs or tools.
To merge or concatenate DataFrames: When you’re merging or concatenating DataFrames, having a header index can cause issues with duplicate columns or unexpected behavior. Removing the header index can help avoid these issues.
Now that we’ve covered why you might want to remove the header index, let’s move on to how to do it.
How to Remove the Header Index in Pandas
Removing the header index in Pandas is actually quite simple. There are a few different ways to do it, depending on your specific use case.
Method 1: Use the header Parameter when Reading in the Data
If you’re reading in data from a file, you can use the header
parameter in the read_csv()
function to specify that the first row of the file should not be treated as the header index.
Here’s an example:
import pandas as pd
df = pd.read_csv('data.csv', header=None)
print(df)
Output:
0 1 2
0 Alice 25 50000
1 Bob 30 60000
2 Charlie 35 70000
In this example, we’re reading in data from a CSV file called “data.csv”. The header=None
parameter tells Pandas that there is no header index in the file, so it should use the first row of data as the column labels instead.
Method 2: Use the columns Attribute to Remove the Header
If you already have a DataFrame and you want to remove its header (i.e., the column names), you can achieve this by setting the columns attribute of the DataFrame to None. This will remove the column names and leave you with a DataFrame without headers.
Here’s an example:
import pandas as pd
# Create a DataFrame with a header
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'salary': [50000, 60000, 70000]
})
# Remove the header by setting the columns to None
df.columns = [None] * len(df.columns)
print(df)
Output:
None None None
0 Alice 25 50000
1 Bob 30 60000
2 Charlie 35 70000
In this example, the df.columns = [None] * len(df.columns)
line replaces the column names with None
, effectively removing the headers. The DataFrame will then display without column names.
Remember, this operation doesn’t delete any data rows; it only affects the display of the column names.
Method 3: Use the rename() Method to Rename the Columns
If you want to keep the first row of the DataFrame as the column labels, but you don’t want them to be treated as an index, you can use the rename()
method to rename the columns.
Here’s an example:
import pandas as pd
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'salary': [50000, 60000, 70000]
})
df = df.rename(columns=df.iloc[0]).drop(df.index[0])
print(df)
Output:
Alice 25 50000
1 Bob 30 60000
2 Charlie 35 70000
In this example, we’re using the rename()
method to rename the columns of the DataFrame to match the values in the first row of the DataFrame (df.iloc[0]
). We’re then using the drop()
method to remove the first row of the DataFrame, which was previously the header index.
Conclusion
Removing the header index in a Pandas DataFrame is a simple but useful technique for data scientists and software engineers. By removing the header index, you can make your DataFrame easier to read and manipulate, avoid issues with merging or concatenating DataFrames, and export your data to files more easily.
In this tutorial, we covered three different methods for removing the header index in Pandas: using the header
parameter when reading in data, using the drop()
method to delete the first row of the DataFrame, and using the rename()
method to rename the columns.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.