How to Remove Decimal Points in Pandas A Guide for Data Scientists
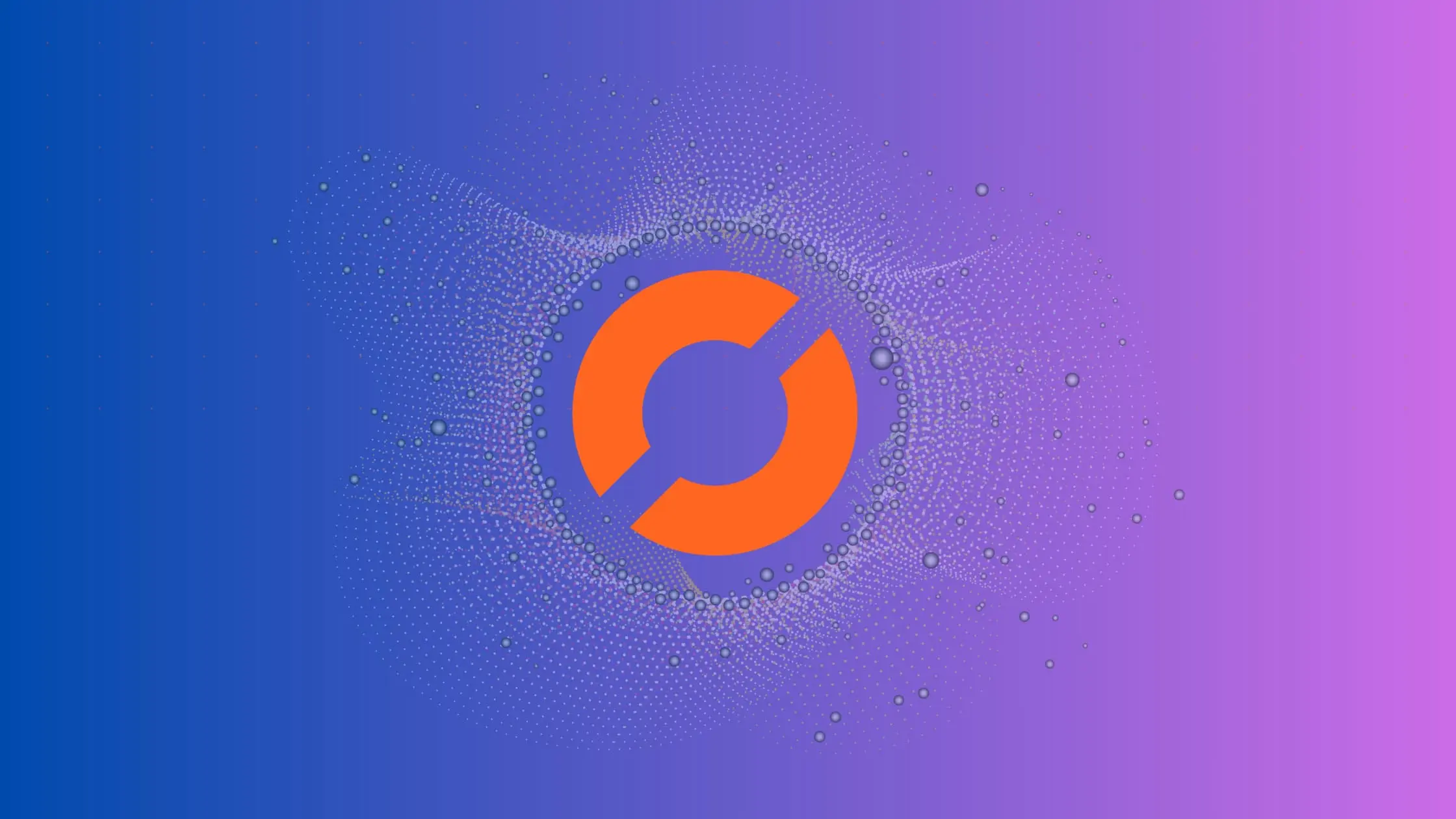
As a data scientist, you know that working with large datasets can be a complex and challenging task. One common issue that often arises is dealing with decimal points in your data. While decimal points can be useful in some situations, they can also be problematic when you need to perform calculations or when you want to display your data in a more readable format.
Fortunately, there are several ways to remove decimal points in pandas, a popular data manipulation library in Python. In this article, we’ll explore some of the most effective methods for removing decimal points in pandas and provide examples of how to implement them in your code.
Table of Contents
Method 1: Using the round() Function
The simplest way to remove decimal points in pandas is by using the round()
function. This function rounds a given number to a specified number of decimal places. To remove all decimal points, you can set the number of decimal places to 0. Here’s an example:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'col1': [1.234, 2.345, 3.456]})
# round all values to 0 decimal places
df['col1'] = df['col1'].round(0)
print(df)
Output:
col1
0 1.0
1 2.0
2 3.0
As you can see, the `round()`` function has removed all decimal points from the values in the ‘col1’ column of the dataframe.
Pros
- Simple and straightforward.
- Allows specifying the number of decimal places to round to.
Cons
- May not be suitable for scenarios where rounding is not the desired operation.
Method 2: Using the astype() Function
Another method for removing decimal points in pandas is by using the astype()
function. This function changes the data type of a pandas series or dataframe. To remove decimal points, you can convert the data type of the series or dataframe to an integer. Here’s an example:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'col1': [1.234, 2.345, 3.456]})
# convert the values in 'col1' to integers
df['col1'] = df['col1'].astype(int)
print(df)
Output:
col1
0 1
1 2
2 3
In this example, the astype()
function has converted the values in the ‘col1’ column of the dataframe to integers, effectively removing all decimal points.
Pros
- Changes the data type to integer, directly removing decimal points.
- Can be useful when you specifically want integers.
Cons
- Rounds towards zero, which may not be suitable for rounding down in all cases.
Method 3: Using the apply() Function with a Lambda Function
The apply()
function in pandas applies a given function to each element of a pandas series or dataframe. This function can be a built-in function, a user-defined function, or a lambda function. To remove decimal points using the apply()
function, you can create a lambda function that rounds each value to 0 decimal places. Here’s an example:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'col1': [1.234, 2.345, 3.456]})
# apply a lambda function to each value in 'col1'
df['col1'] = df['col1'].apply(lambda x: round(x, 0))
print(df)
Output:
col1
0 1.0
1 2.0
2 3.0
In this example, the apply()
function has applied the lambda function to each value in the ‘col1’ column of the dataframe, rounding each value to 0 decimal places.
Pros
- Offers flexibility by allowing the use of custom rounding functions.
- Useful for more complex rounding scenarios.
Cons
- Can be less concise than other methods for simple rounding operations.
Method 4: Using the floor() Function
The floor()
function in pandas rounds a given number down to the nearest integer. This function can be useful when you want to remove decimal points and round down at the same time. Here’s an example:
import pandas as pd
import numpy as np
# create a sample dataframe
df = pd.DataFrame({'col1': [1.234, 2.345, 3.456]})
# round down each value in 'col1'
df['col1'] = np.floor(df['col1'])
print(df)
Output:
col1
0 1.0
1 2.0
2 3.0
In this example, the floor()
function has rounded each value in the ‘col1’ column of the dataframe down to the nearest integer, effectively removing all decimal points.
Pros
- Rounds down to the nearest integer, which can be useful in certain situations.
Cons:
- Limited to rounding down; not suitable for scenarios where rounding up or to the nearest integer is needed.
General Considerations:
Performance:
- The performance of these methods may vary depending on the size of the dataset.
- The astype() method is likely to be faster since it directly changes the data type.
Rounding Behavior:
- Consider the rounding behavior required for your specific use case (rounding up, down, or to the nearest integer).
Flexibility:
- If you need more flexibility in rounding behavior, the apply() method with a lambda function is a good choice.
Conclusion
In this article, we’ve explored several methods for removing decimal points in pandas, a popular data manipulation library in Python. These methods include using the round() function, the astype()
function, the apply()
function with a lambda function, and the floor()
function. By implementing these methods in your code, you can effectively remove decimal points from your data and make it more readable and easier to work with.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.