How to Remove Characters from a Pandas Column A Data Scientists Guide
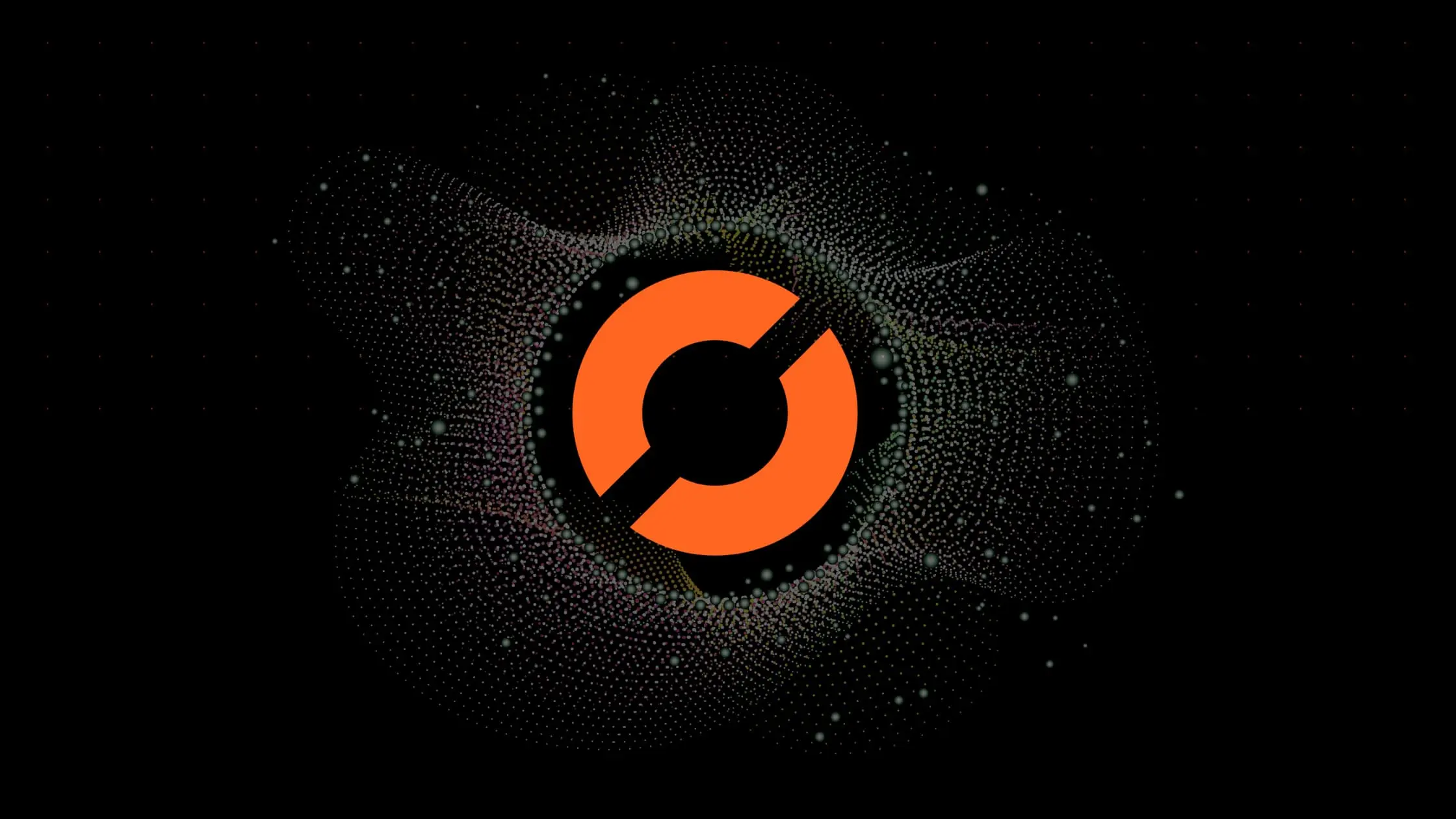
As a data scientist, one of the most common tasks you’ll encounter is cleaning and preprocessing data. In particular, you may need to remove certain characters from a pandas column to extract relevant information or convert the data into a more usable format. In this article, we’ll cover the different methods for removing characters from a pandas column and provide examples to help you get started.
Table of Contents
What is Pandas?
Pandas is a popular open-source data analysis and manipulation library for Python. It provides a flexible and efficient way to work with structured data, such as tabular data, time series, and matrices. Pandas is built on top of NumPy, another popular library for scientific computing in Python, and provides a higher-level interface for data manipulation and analysis.
Pandas provides two main data structures: Series and DataFrame. A Series is a one-dimensional labeled array that can hold any data type, while a DataFrame is a two-dimensional labeled data structure that can hold multiple Series. You can think of a DataFrame as a spreadsheet or a SQL table, where each column represents a variable and each row represents a record.
How to Remove Characters from a Pandas Column
There are several methods for removing characters from a pandas column, depending on the specific requirements of your data. Here, we’ll cover some of the most common methods.
Method 1: Using the str.replace()
Method
The str.replace()
method is a convenient way to replace a specific substring or character in a pandas column. This method works by searching for a specified string or character in each element of the column and replacing it with a new string or character.
Here’s an example of how to use the str.replace()
method to remove a specific character, such as a comma, from a pandas column:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'name': ['Alice, Bob', 'Charlie, David', 'Eve, Frank']})
# Remove the comma from the name column
df['name'] = df['name'].str.replace(',', '')
print(df)
Output:
name
0 Alice Bob
1 Charlie David
2 Eve Frank
In this example, we created a sample DataFrame with a column named ‘name’ that contains names separated by commas. We then used the str.replace()
method to remove the commas from the name column and replaced them with an empty string.
Method 2: Using Regular Expressions
Regular expressions, or regex, are a powerful tool for pattern matching and text manipulation. They allow you to match and extract specific patterns of characters from a string, such as digits, letters, or symbols. Pandas provides a convenient way to apply regex to a pandas column using the str.extract() method.
Here’s an example of how to use regex to extract a specific pattern of characters from a pandas column:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'phone': ['(123) 456-7890', '(456) 789-0123', '(789) 012-3456']})
# Extract the digits from the phone column
df['phone'] = df['phone'].str.extract(r'(\d{3})\D*(\d{3})\D*(\d{4})')
print(df)
Output:
phone
0 1234567890
1 4567890123
2 7890123456
In this example, we created a sample DataFrame with a column named ‘phone’ that contains phone numbers in a specific format. We then used regex to extract the digits from the phone column and concatenate them into a single string.
Method 3: Using a Custom Function
If the above methods do not meet your requirements, you can create a custom function to remove characters from a pandas column. This method provides more flexibility and allows you to customize the cleaning process based on your specific needs.
Here’s an example of how to create a custom function to remove a specific character, such as a hyphen, from a pandas column:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'date': ['2022-01-01', '2022-02-01', '2022-03-01']})
# Define a custom function to remove the hyphen from the date column
def remove_hyphen(column):
return column.str.replace('-', '')
# Apply the custom function to the date column
df['date'] = remove_hyphen(df['date'])
print(df)
Output:
date
0 20220101
1 20220201
2 20220301
In this example, we created a sample DataFrame with a column named 'date'
that contains dates in a specific format. We then defined a custom function named remove_hyphen
that uses the str.replace()
method to remove the hyphen from the date column. Finally, we applied the custom function to the date column using the apply()
method.
Common Pitfalls
Type Mismatch
An often encountered stumbling block involves attempting string operations on columns that aren’t of type str
. It’s imperative to verify that the column is appropriately cast to a string type before initiating any string manipulation.
Handling NaN Values
Exercise caution when dealing with NaN (Not a Number) values. Directly applying string operations to columns with missing values can lead to unforeseen complications. Prioritize thorough handling of NaN values using methods such as fillna()
before embarking on string manipulations.
Case Sensitivity Quandary
Default case sensitivity in regular expressions can be a source of errors. It’s essential to be vigilant regarding case differences when employing regular expressions in character removal operations.
Best Practices
Graceful Handling of Missing Values
Adopt practices that gracefully manage missing values in your dataset. Techniques like utilizing the fillna()
method can effectively address missing values before executing string operations.
Performance Considerations
When dealing with extensive datasets, carefully assess the performance implications of various methods. Opt for vectorized operations whenever feasible, as they generally offer superior efficiency compared to iterative approaches.
Leverage Vectorized Operations
Harness the power of Pandas' vectorized operations to enhance performance. Minimize the use of row-wise iteration, as it can be less efficient compared to vectorized alternatives.
Conclusion
In this article, we covered the different methods for removing characters from a pandas column, including using the str.replace()
method, regular expressions, and custom functions. We also provided examples to help you understand how to implement these methods in your own data cleaning and preprocessing tasks.
Cleaning and preprocessing data is an important part of the data analysis process, and pandas provides a powerful set of tools for these tasks. By mastering the different methods for removing characters from a pandas column, you’ll be better equipped to handle a wide range of data cleaning challenges and extract insights from your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.