How to Read CSV Files as Strings in Pandas
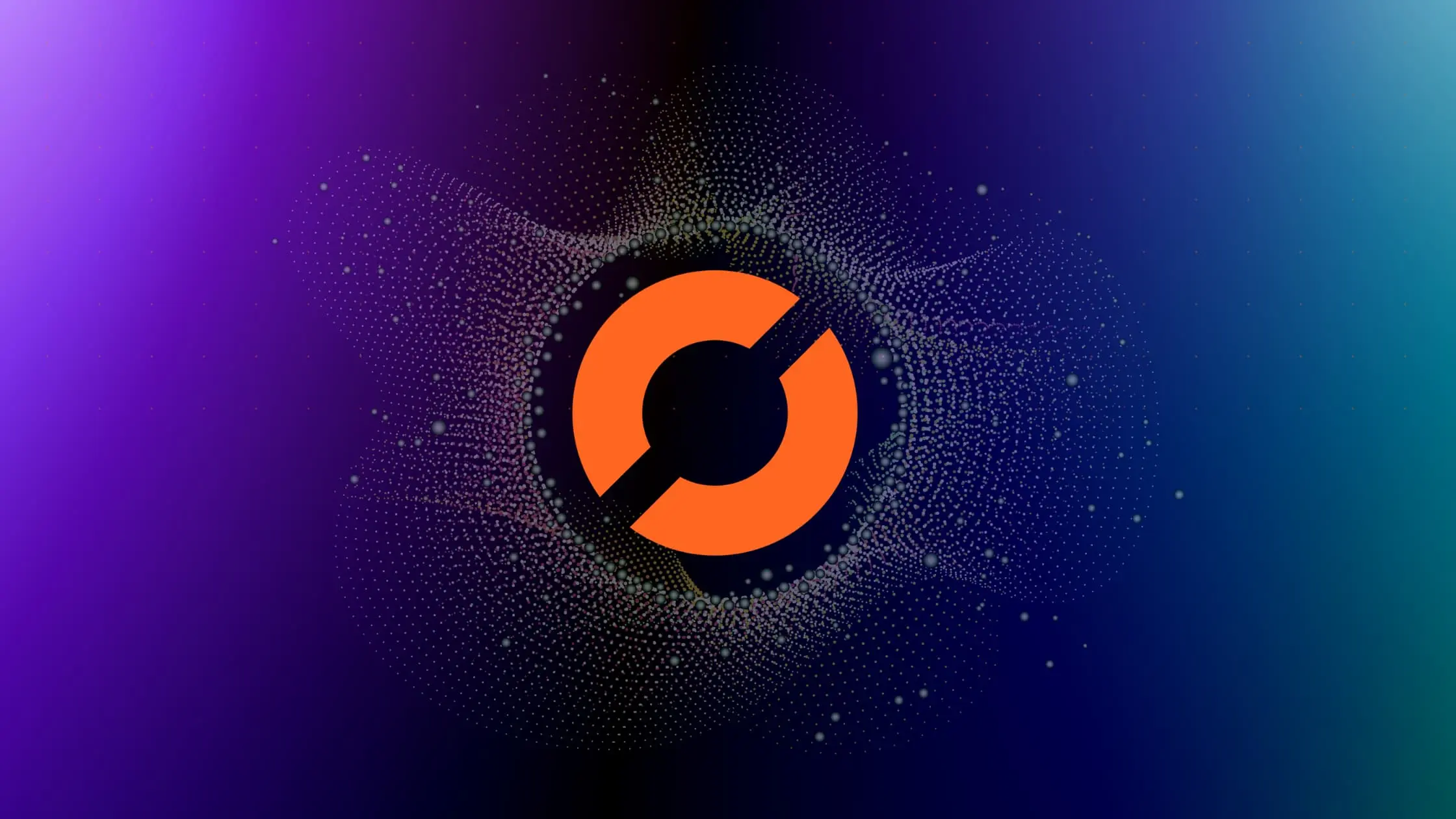
As a data scientist or software engineer, you will often work with CSV files to analyze and manipulate data. The Pandas library offers an easy-to-use solution for reading and manipulating CSV files in Python. However, by default, Pandas will infer the data type of each column in the CSV file, which can sometimes lead to unexpected behavior. In this article, we will explore how to read CSV files as string type in Pandas.
What is Pandas?
Pandas is an open-source data analysis and manipulation library for the Python programming language. It provides data structures and functions necessary for working with structured data seamlessly. Pandas is built on top of NumPy, another popular Python library for scientific computing, and provides additional functionality for data manipulation.
The Problem with Reading CSV Files in Pandas
When you read a CSV file in Pandas, the library infers the data type of each column based on the data in the file. This can be useful in many cases, as it saves you the trouble of specifying the data types manually. However, there are situations where you may want to read the data as string type, regardless of its actual data type in the file.
For example, consider a CSV file with a column called “Phone Number”. If the phone numbers in the file are formatted as strings, you may want to read them as strings in Pandas, even though they could be read as integers or floats. If you don’t read the data as strings, you may lose leading zeros or encounter other unexpected behavior.
Reading CSV Files as String Type
To read a CSV file as string type in Pandas, you can use the dtype
parameter of the read_csv()
function. The dtype
parameter allows you to specify the data type of each column in the CSV file.
Here’s an example of how you can read a CSV file as string type:
Name,Phone Number,Age
John Doe,123-456-7890,25
Jane Smith,987-654-3210,30
Bob Johnson,555-123-4567,22
Alice Brown,333-888-9999,28
import pandas as pd
# Specify the data type for all columns as string using the dtype parameter
df = pd.read_csv("data.csv", dtype=str)
# Display the DataFrame
print(df)
Output:
Name Phone Number Age
0 John Doe 123-456-7890 25
1 Jane Smith 987-654-3210 30
2 Bob Johnson 555-123-4567 22
3 Alice Brown 333-888-9999 28
In this example, we use the read_csv()
function to read a CSV file called “data.csv”. We also specify the dtype
parameter as str
, which tells Pandas to read all the columns as strings.
Now, Pandas will read all the columns in the CSV file as strings, even if they could be read as other data types. This ensures that you get the data in the format you want, without any unexpected behavior.
Specifying Data Types for Specific Columns
In some cases, you may want to read only specific columns as string type, while letting Pandas infer the data type of other columns. To do this, you can specify the data type for each column in a dictionary, and pass it to the dtype
parameter of the read_csv()
function.
Here’s an example of how you can specify the data type for specific columns:
import pandas as pd
# Specify the data type for each column
dtype = {"Name": str, "Phone Number": str, "Age": str}
# Read the CSV file with specified data types
df = pd.read_csv("data.csv", dtype=dtype)
# Display the DataFrame
print(df)
Output:
Name Phone Number Age
0 John Doe 123-456-7890 25
1 Jane Smith 987-654-3210 30
2 Bob Johnson 555-123-4567 22
3 Alice Brown 333-888-9999 28
In this example, we use a dictionary to specify the data type for two columns: “Phone Number” and “Age”. We specify “Phone Number” as str
, and “Age” as int
. Pandas will infer the data type for all other columns.
Conclusion
Reading CSV files as string type in Pandas is important in certain situations where you want to ensure that the data is read in the format you want, without any unexpected behavior. By using the dtype
parameter of the read_csv()
function, you can easily read CSV files as string type, either for all columns or for specific ones. This ensures that you get the data you need to perform your analysis or manipulation tasks accurately.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.