How to Properly Reverse a Pandas DataFrame
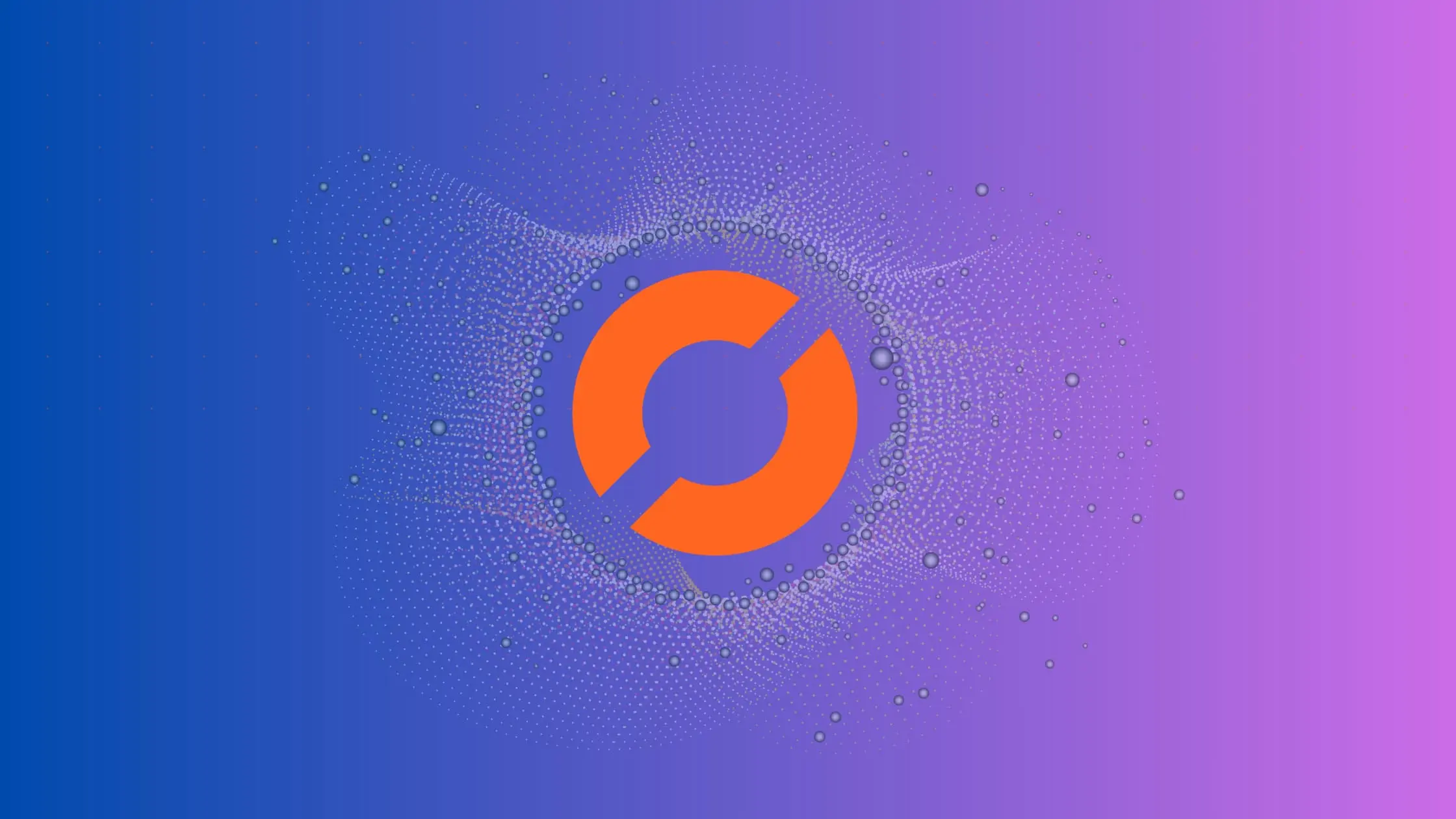
As a data scientist or software engineer, you may find yourself working with large datasets in Pandas, and at times, you may need to manipulate the data in various ways. One such operation is reversing a Pandas DataFrame, which may seem like a simple task, but it can be tricky if not done properly. In this article, we will explore the right way to reverse a Pandas DataFrame.
What is a Pandas DataFrame?
Pandas is a powerful data manipulation library in Python that provides high-performance, easy-to-use data structures, and data analysis tools. The primary data structure in Pandas is the DataFrame, which is a two-dimensional table-like structure that stores data in rows and columns. The rows represent the observations or samples, while the columns represent the variables or features of the data.
Why Reverse a Pandas DataFrame?
There are several reasons why you may need to reverse a Pandas DataFrame, including:
- Reversing the order of rows or columns to match a specific order or sequence.
- Reversing the order of data to visualize trends or patterns.
- Reversing the order of data to perform time-series analysis.
How to Reverse a Pandas DataFrame
The most common way to reverse a Pandas DataFrame is by using the iloc
method, which is a powerful indexing operator that allows you to select rows and columns based on their integer positions. The iloc
method allows you to slice, select, and modify data in a Pandas DataFrame by using integer positions instead of labels.
Reverse Rows in a Pandas DataFrame
To reverse the order of rows in a Pandas DataFrame, you can use the iloc
method with a negative step value. The negative step value tells the iloc
method to start from the last row and move backward by one row at a time.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# reverse the order of rows
df_reverse_rows = df.iloc[::-1]
print(df_reverse_rows)
Output:
A B C
2 3 6 9
1 2 5 8
0 1 4 7
As you can see, the iloc[::-1]
method reversed the order of rows in the Pandas DataFrame.
Reverse Columns in a Pandas DataFrame
To reverse the order of columns in a Pandas DataFrame, you can use the iloc
method with a negative step value on the columns axis. The negative step value tells the iloc
method to start from the last column and move backward by one column at a time.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# reverse the order of columns
df_reverse_cols = df.iloc[:, ::-1]
print(df_reverse_cols)
Output:
C B A
0 7 4 1
1 8 5 2
2 9 6 3
As you can see, the iloc[:, ::-1]
method reversed the order of columns in the Pandas DataFrame.
Reverse Rows and Columns in a Pandas DataFrame
To reverse the order of both rows and columns in a Pandas DataFrame, you can combine the iloc
method with a negative step value on both the rows and columns axis.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# reverse the order of rows and columns
df_reverse_both = df.iloc[::-1, ::-1]
print(df_reverse_both)
Output:
C B A
2 9 6 3
1 8 5 2
0 7 4 1
As you can see, the iloc[::-1, ::-1]
method reversed the order of both rows and columns in the Pandas DataFrame.
Conclusion
In this article, we have explored the right way to reverse a Pandas DataFrame. We have seen that the iloc
method is a powerful indexing operator that allows you to select rows and columns based on their integer positions. By using a negative step value with the iloc
method, you can reverse the order of rows and columns in a Pandas DataFrame. Properly reversing a Pandas DataFrame is essential when manipulating data for visualization, analysis, or machine learning tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.