How to Prevent Pandas readcsv from Treating the First Row as Header of Column Names
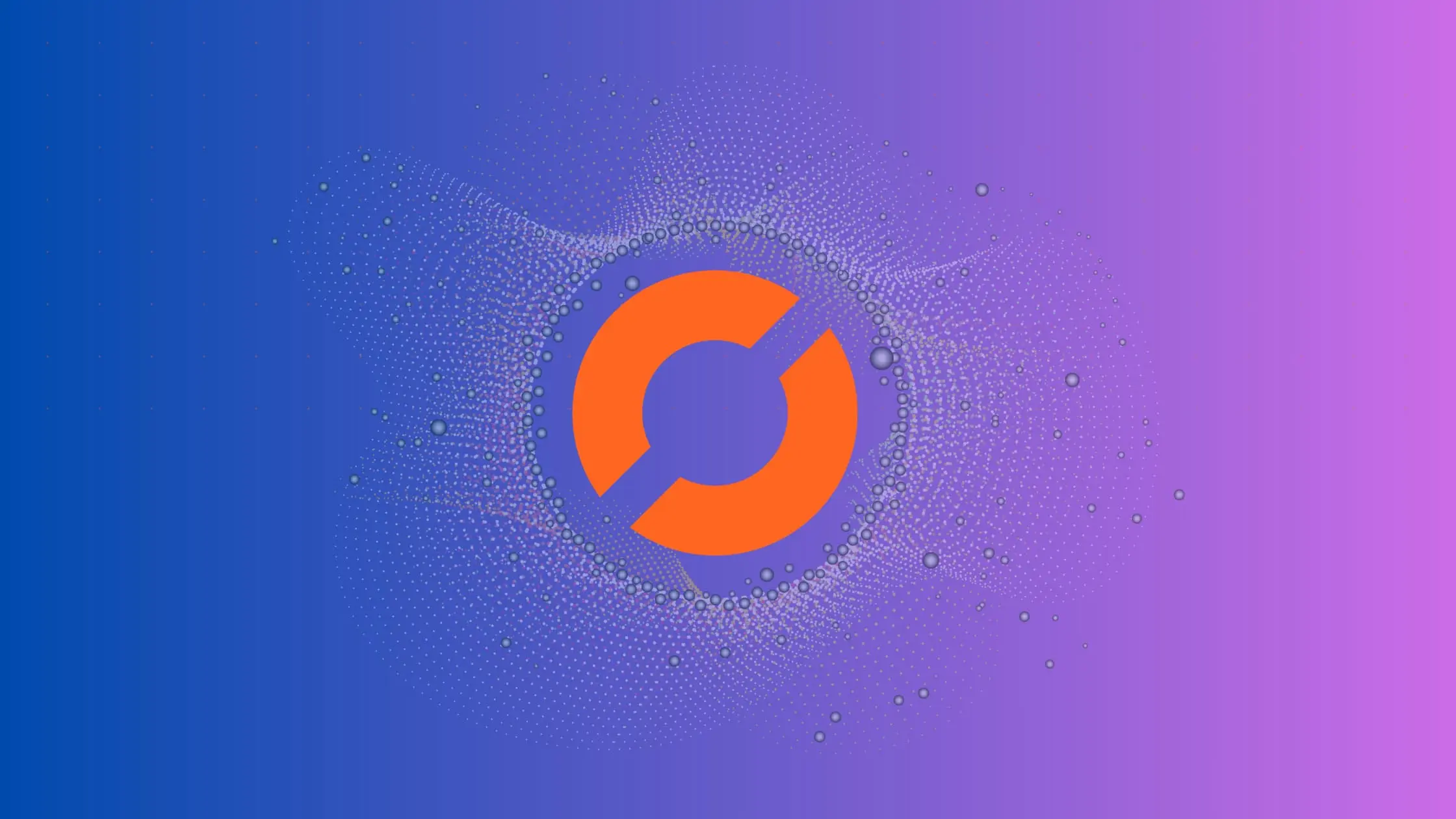
How to Prevent Pandas readcsv from Treating the First Row as Header of Column Names
When working with data in Python, pandas is one of the most popular libraries used for data manipulation and analysis. One of the most common tasks when working with pandas is reading CSV files into a pandas DataFrame using the read_csv
method. By default, read_csv
assumes that the first row of the CSV file contains the header of the DataFrame. However, there may be situations where the first row of the CSV file does not contain the header, and you want to prevent read_csv
from treating it as such. In this article, we will explain how to prevent pandas read_csv
from treating the first row as the header of column names.
Why would you want to prevent pandas read_csv from treating the first row as header?
There are several reasons why you might want to prevent pandas read_csv
from treating the first row as the header of column names. The most common reason is that your CSV file may not contain a header row, or the header row may be incomplete or incorrect. In such cases, pandas read_csv
will try to use the first row as the header, which can lead to errors or incorrect data analysis. Additionally, if your CSV file contains a mix of data types in the first row, it can be difficult for pandas to correctly infer the data types for the columns.
How to Prevent pandas read_csv from Treating the First Row as Header
To prevent pandas read_csv
from treating the first row as the header of column names, you can use the header
parameter and set it to None
. This will tell pandas that there is no header row in the CSV file.
import pandas as pd
df = pd.read_csv('data.csv', header=None)
In the above code, we are reading a CSV file named data.csv
and setting the header
parameter to None
. This will prevent pandas from treating the first row as the header of column names. The resulting DataFrame will have columns named 0
, 1
, 2
, etc., instead of using the values from the first row as column names.
If your CSV file contains a header row but you want to skip it, you can use the skiprows
parameter to skip the first row.
import pandas as pd
df = pd.read_csv('data.csv', skiprows=1)
In the above code, we are reading a CSV file named data.csv
and setting the skiprows
parameter to 1
. This will skip the first row of the CSV file and use the values from the second row as the header of column names.
Conclusion
In this article, we have explained how to prevent pandas read_csv
from treating the first row as the header of column names. By setting the header
parameter to None
, you can tell pandas that there is no header row in the CSV file. If your CSV file contains a header row but you want to skip it, you can use the skiprows
parameter to skip the first row. These simple tips can help you avoid errors and ensure that your data analysis is accurate.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.