How to Predict Using a PyTorch Model
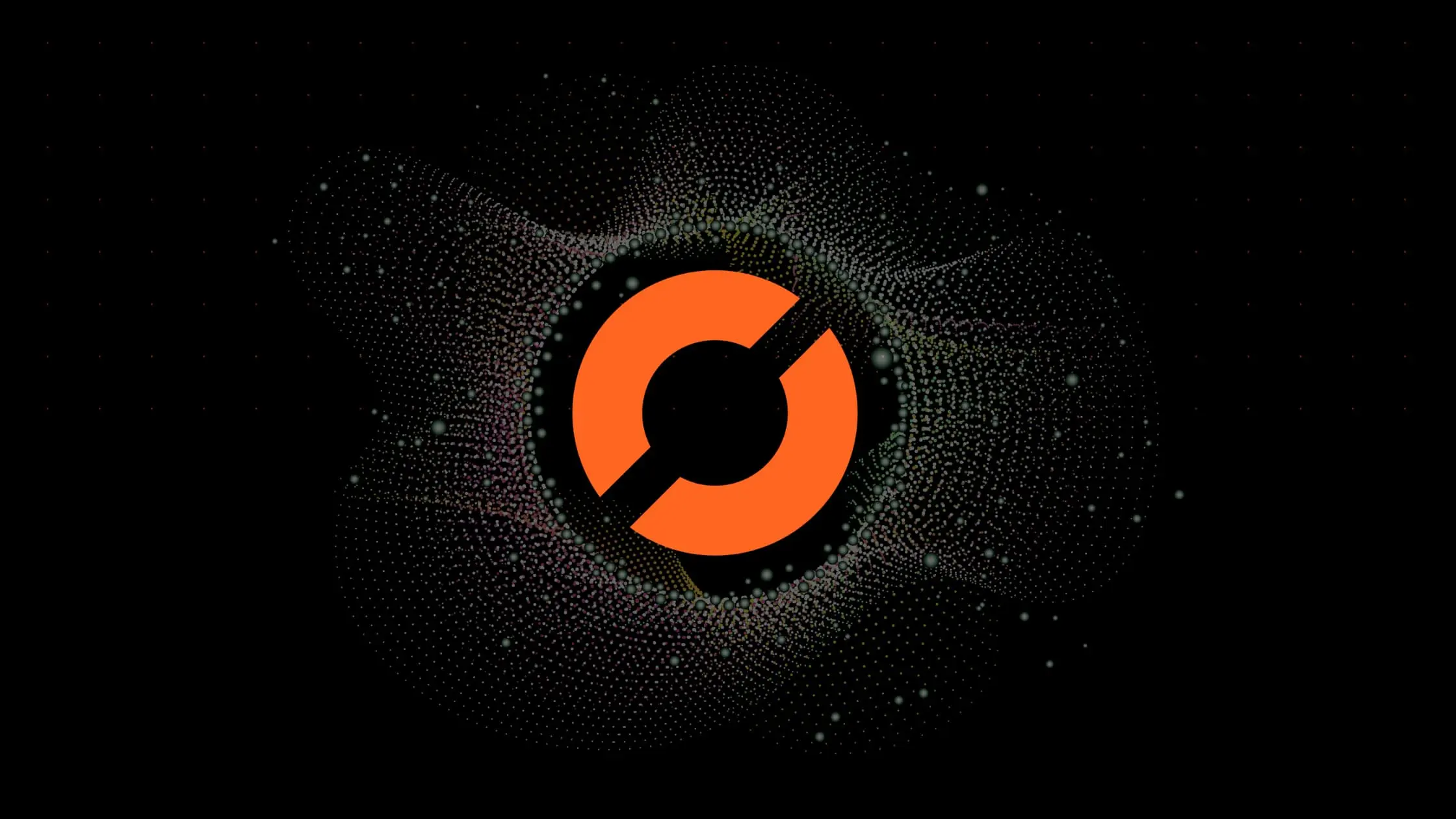
As a data scientist or software engineer, you may have come across the need to predict outcomes using a PyTorch model. PyTorch is a popular open-source machine learning library that is widely used in research and production environments. In this article, we will explore the steps involved in predicting outcomes using a PyTorch model.
Table of Contents
- What is PyTorch?
- Preparing the Data
- Building the Model
- Predicting Using the Model
- Common Errors and How to Handle Them
- Using ResNet to Predict a Dog
- Conclusion
What is PyTorch?
PyTorch is a widely used open-source machine learning library developed by Facebook’s AI Research team. It is based on Torch, which is a scientific computing framework with wide support for machine learning algorithms. PyTorch is designed to be flexible and efficient, making it ideal for research and production environments.
PyTorch supports a wide range of machine learning algorithms, including deep learning. It provides a simple and easy-to-use interface for building and training neural networks. PyTorch also supports automatic differentiation, which makes it easy to calculate gradients and perform backpropagation.
Preparing the Data
Before we can predict outcomes using a PyTorch model, we need to prepare the data. This involves loading the data, preprocessing it, and splitting it into training and test sets.
Loading the Data
The first step in preparing the data is to load it into memory. PyTorch provides several data loading utilities that make this process easy. For example, the torchvision
package provides utilities for loading common datasets such as MNIST and CIFAR-10.
If you have your own dataset, you can use torch.utils.data.Dataset
to create a custom dataset. You can then use torch.utils.data.DataLoader
to load the dataset into memory.
Preprocessing the Data
Once the data is loaded, we need to preprocess it. This involves normalizing the data and converting it into a format that can be used by the PyTorch model.
Normalization is an important step in preprocessing the data. It involves scaling the data so that it has a mean of 0 and a standard deviation of 1. This helps to ensure that the model is able to learn from the data more effectively.
Splitting the Data
After preprocessing the data, we need to split it into training and test sets. The training set is used to train the model, while the test set is used to evaluate the performance of the model.
PyTorch provides several utilities for splitting the data. For example, you can use torch.utils.data.random_split
to split the data randomly. Alternatively, you can use sklearn.model_selection.train_test_split
to split the data using a specific ratio.
Building the Model
Once the data is prepared, we can start building the PyTorch model. This involves defining the network architecture and training the model.
Defining the Network Architecture
The first step in building the PyTorch model is to define the network architecture. This involves creating a class that inherits from torch.nn.Module
.
The class should define the layers of the network using the various PyTorch modules such as torch.nn.Linear
and torch.nn.Conv2d
. You can also define custom layers using torch.nn.Module
.
Training the Model
After defining the network architecture, we can start training the model. This involves defining a loss function and an optimizer, and then running the training loop.
The loss function is used to measure the difference between the predicted and actual values. PyTorch provides several loss functions such as torch.nn.MSELoss
and torch.nn.CrossEntropyLoss
.
The optimizer is used to update the weights of the model during training. PyTorch provides several optimizers such as torch.optim.Adam
and torch.optim.SGD
.
The training loop involves iterating over the training data and updating the model weights using the optimizer. After each epoch, the model is evaluated on the test data to measure its performance.
Predicting Using the Model
Once the model is trained, we can use it to predict outcomes. This involves loading the saved model and using it to predict outcomes on new data.
Loading the Model
To load the saved model, we can use the torch.load
function. This function loads the saved model state dictionary into memory.
model = torch.load('model.pt')
Predicting Outcomes
To predict outcomes using the loaded model, we need to pass the input data through the model and get the predicted output.
y_pred = model(x_test)
The x_test
variable should be a PyTorch tensor that contains the input data. The y_pred
variable will contain the predicted output.
Common Errors and How to Handle Them
Data Mismatch
Mismatch between training and prediction data can lead to inaccurate results. Ensure consistency in data formats and preprocess new data similarly to the training data.
Model Version Compatibility
Ensure that the PyTorch version and model architecture are compatible. Model files trained with different versions may not load properly.
Input Shape Mismatch
Check input shapes when making predictions. Mismatch between the expected and provided input shapes can result in errors. Adjust data preprocessing accordingly.
GPU Memory Issues
Large models or datasets may exceed GPU memory. Batch processing or using model optimization techniques like pruning can help mitigate memory issues.
Example: Using ResNet to Predict a Dog
Let’s demonstrate the prediction process using the ResNet model and the following in image. First, load the pre-trained ResNet model, preprocess the input image, and make predictions.
# Example code using PyTorch and torchvision
import torch
from torchvision import models, transforms
from PIL import Image
# Load pre-trained ResNet model
resnet_model = models.resnet50(pretrained=True)
resnet_model.eval()
# Preprocess input image
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]),
])
image_path = 'path_to_dog_image.jpg'
image = Image.open(image_path)
input_tensor = transform(image)
input_batch = input_tensor.unsqueeze(0)
# Make prediction
with torch.no_grad():
output = resnet_model(input_batch)
# Get predicted class
_, predicted_class = torch.max(output, 1)
print(f"Predicted class index: {predicted_class.item()}")
Output:
Predicted class index: 207
By looking at this label map, we can see that the model accurately predicted the image as a golden-retriever
.
Conclusion
Predicting outcomes using a PyTorch model involves several steps such as preparing the data, building the model, and predicting using the model. PyTorch provides several utilities for loading and preprocessing the data, as well as defining and training the model. By following these steps, you can build and use PyTorch models to predict outcomes in various applications.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.