How to Perform a Union of Two Pandas DataFrames
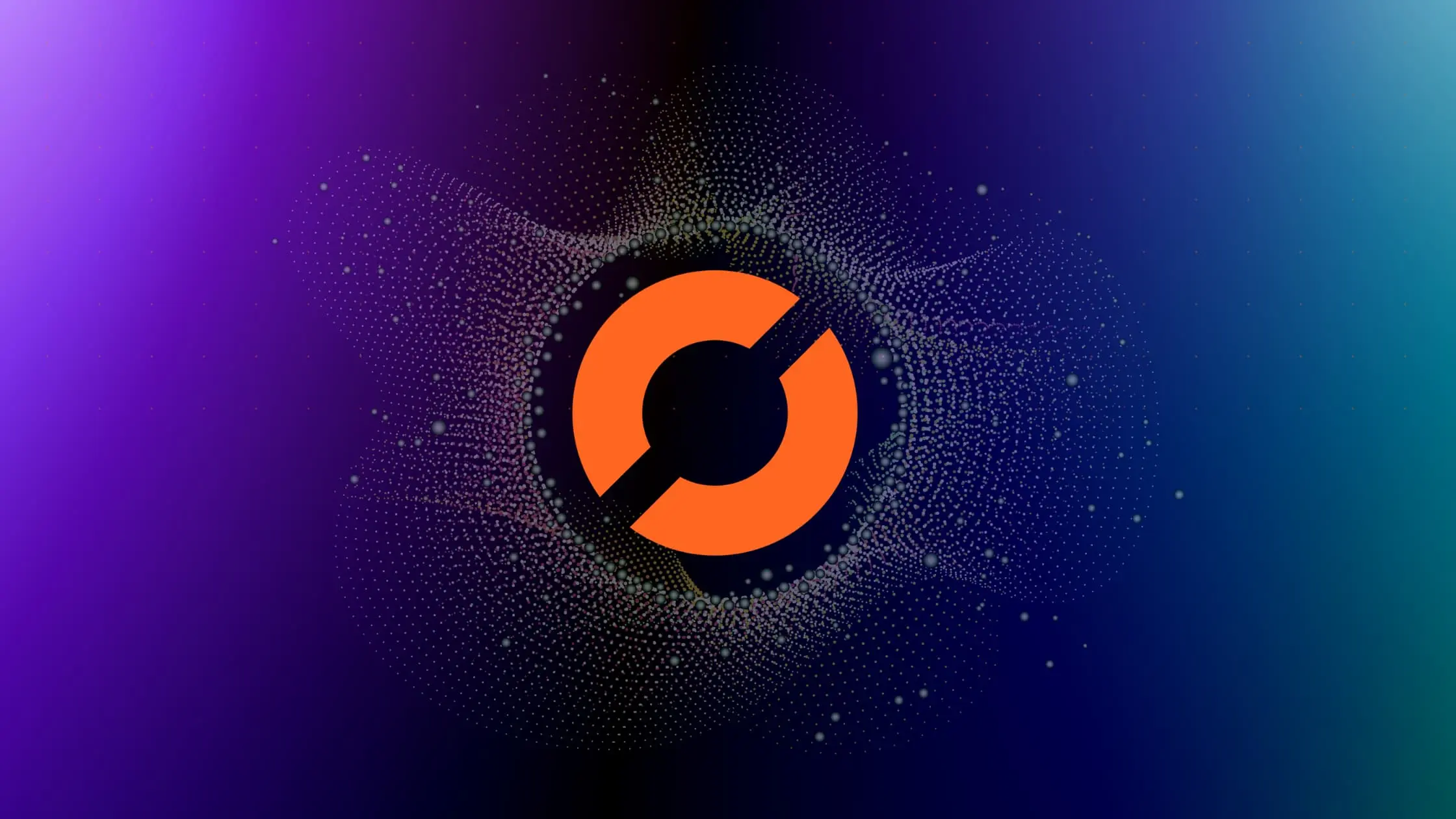
Pandas is a popular Python library for data manipulation and analysis. One of the most common tasks in data science is combining or merging data from multiple sources. In this article, we’ll explore how to perform a union of two pandas DataFrames.
Table of Contents
- What is a Union of DataFrames?
- How to Perform a Union of Two Pandas DataFrames
- Pros and Cons of Each Method
- Best Practices for Union Operations
- Common Errors and How to Handle Them
- Conclusion
What is a Union of DataFrames?
A union of two pandas DataFrames is the process of combining the rows from both DataFrames into a single DataFrame. This is similar to the SQL UNION operation, where two SELECT statements are combined to produce a single result set.
In pandas, we can perform a union of DataFrames using the concat
function. This function takes a list of DataFrames as input and concatenates them along a specified axis. By default, concat
concatenates DataFrames along the rows (axis=0), which is what we need for a union.
How to Perform a Union of Two Pandas DataFrames
Let’s start by creating two sample DataFrames that we’ll use for our examples. We’ll create two DataFrames with different columns and some overlapping data.
import pandas as pd
# Create the first DataFrame
df1 = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'city': ['New York', 'Chicago', 'San Francisco']
})
# Create the second DataFrame
df2 = pd.DataFrame({
'name': ['Dave', 'Eve', 'Charlie'],
'age': [40, 45, 35],
'state': ['Texas', 'California', 'California']
})
The first DataFrame df1
contains information about three people, including their name, age, and city. The second DataFrame df2
contains information about three other people, including their name, age, and state.
Using concat
To perform a union of these two DataFrames, we simply need to concatenate them using the concat
function:
# Concatenate the two DataFrames
union_df = pd.concat([df1, df2])
print(union_df)
The output of this code will be a new DataFrame that contains all six rows from both DataFrames:
name age city state
0 Alice 25 New York NaN
1 Bob 30 Chicago NaN
2 Charlie 35 San Francisco NaN
0 Dave 40 NaN Texas
1 Eve 45 NaN California
2 Charlie 35 NaN California
As you can see, the resulting DataFrame contains all columns from both DataFrames, and all rows from both DataFrames have been concatenated. The index values from the original DataFrames have been preserved in the union DataFrame, resulting in duplicate index values.
If you want to reset the index of the union DataFrame, you can use the reset_index
function:
# Reset the index of the union DataFrame
union_df = union_df.reset_index(drop=True)
print(union_df)
The output of this code will be the same DataFrame as before, but with a new index:
name age city state
0 Alice 25 New York NaN
1 Bob 30 Chicago NaN
2 Charlie 35 San Francisco NaN
3 Dave 40 NaN Texas
4 Eve 45 NaN California
5 Charlie 35 NaN California
Using append
:
The append
method simplifies vertical concatenation. Observe its application with real-world data:
# Appending with ignore_index
result_append = df1.append(df2, ignore_index=True)
print(result_append)
Using merge
:
The merge
method, known for precise merging control, is adept at unions. Observe its application with real-world data:
# Merging with outer join
result_merge = pd.merge(df1, df2, how='outer')
print(result_merge)
Output:
name age city state
0 Alice 25 New York NaN
1 Bob 30 Chicago NaN
2 Charlie 35 San Francisco NaN
3 Dave 40 NaN Texas
4 Eve 45 NaN California
5 Charlie 35 NaN California
Pros and Cons of Each Method:
Method | Pros | Cons |
---|---|---|
concat | - Simple syntax - Fast execution | - Potential index handling complexities |
append | - Concise syntax for vertical concatenation | - Limited to appending rows, potential column mismatch challenges |
merge | - Precise control over merging logic | - Complexity, especially for straightforward unions |
Best Practices for Union Operations:
- Ensure consistent column names or use the
ignore_index
parameter. - Validate data types to prevent unexpected mismatches.
- Be mindful of memory usage, especially with extensive datasets.
Common Errors and How to Handle Them:
Mismatched Columns:
Address by ensuring uniform column names or using the ignore_index
parameter.
Duplicate Indices:
Handle by resetting indices using reset_index
.
Missing Data:
Prevent issues by addressing missing data with methods like fillna
or dropna
before union operations.
Conclusion
Performing a union of two pandas DataFrames is a basic operation in data science, and can be accomplished using the concat
function. By concatenating two DataFrames along the rows, we can create a new DataFrame that contains all rows from both DataFrames. The resulting DataFrame will contain duplicate index values, but these can be reset using the reset_index
function.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.