How to Pause a For Loop and Wait for User Input in Matplotlib
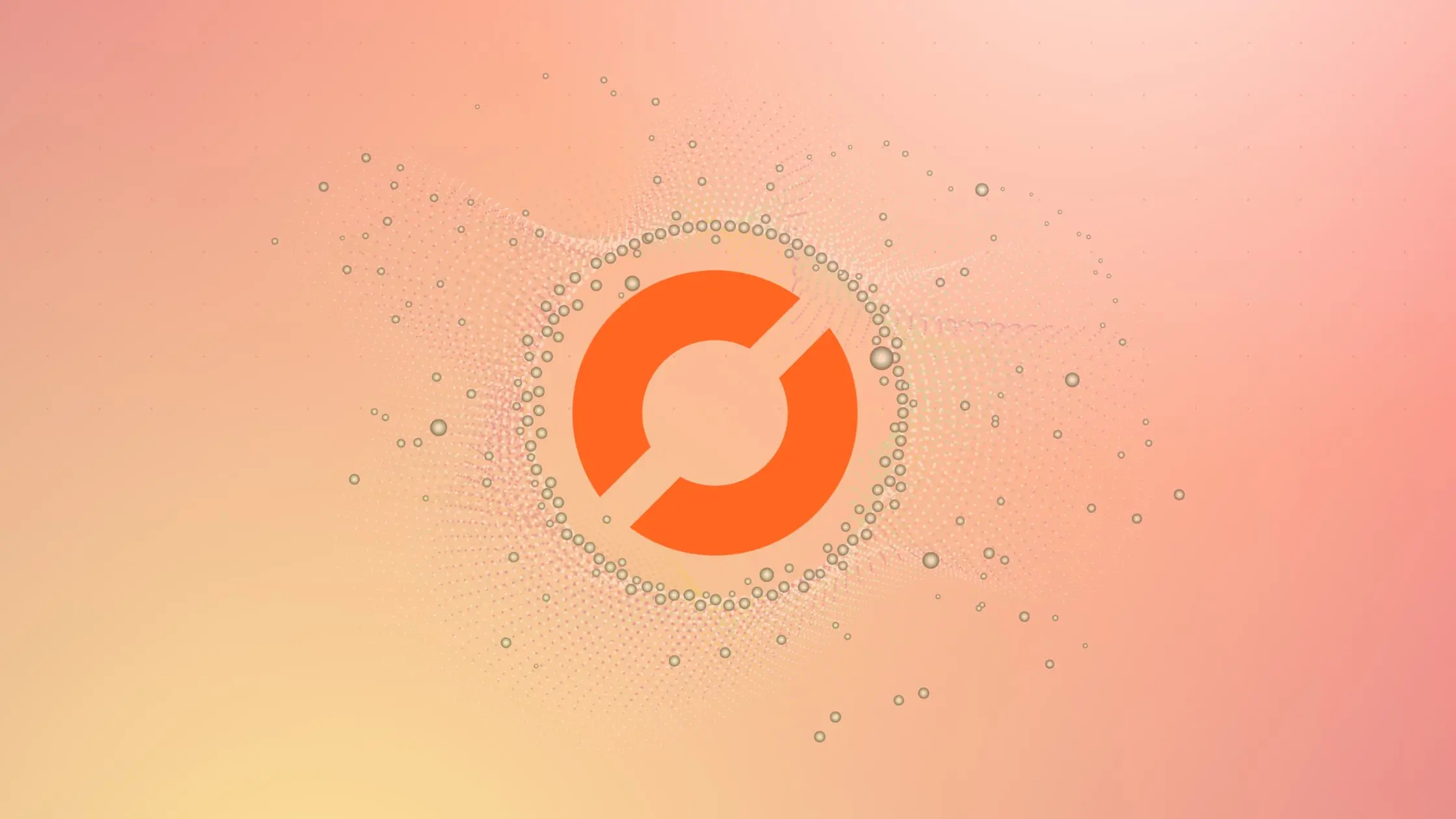
In the world of data science, visualizing data is a crucial step. Matplotlib, a popular Python library, is often used for this purpose. However, there might be instances where you want to pause a for loop and wait for user input while using Matplotlib. This blog post will guide you through the process, step by step.
Table of Contents
- Introduction
- Prerequisites
- Pausing a For Loop
- Integrating User Input with Matplotlib
- Using plt.pause() Function
- Common Errors and How to Handle Them
- Conclusion
Introduction
Matplotlib is a powerful tool for creating static, animated, and interactive visualizations in Python. It’s a versatile library that allows you to generate plots, histograms, power spectra, bar charts, error charts, scatterplots, and more with just a few lines of code.
In some cases, you may want to pause a for loop in your code to wait for user input. This can be useful when you want to create interactive plots or when you need user feedback before proceeding with the rest of your code. This post will show you how to do just that.
Prerequisites
Before we begin, make sure you have the following installed:
- Python 3.6 or later
- Matplotlib
- IPython
You can install these packages using pip:
pip install python matplotlib ipython
Pausing a For Loop
Let’s start by discussing how to pause a for loop. In Python, the input()
function can be used to pause your program and wait for user input. Here’s a simple example:
for i in range(10):
print(i)
input("Press Enter to continue...")
In this code, the for loop will print a number, then pause and wait for the user to press Enter before continuing. Output:
0
Press Enter to continue...
1
Press Enter to continue...
2
Press Enter to continue...
3
Press Enter to continue...
4
Press Enter to continue...
5
Press Enter to continue...
6
Press Enter to continue...
7
Press Enter to continue...
8
Press Enter to continue...
9
Press Enter to continue...
Integrating User Input with Matplotlib
Now, let’s integrate this concept with Matplotlib. Suppose we have a list of x and y coordinates, and we want to plot each point one by one, waiting for user input before plotting the next point.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.ion() # Turn on interactive mode
for i in range(len(x)):
plt.scatter(x[i], y[i])
plt.draw()
input("Press Enter to plot the next point...")
In this code, plt.ion()
turns on the interactive mode in Matplotlib. This allows us to update the plot in real-time. The plt.draw()
function is used to update the plot with the new point.
Using plt.pause() Function
The plt.pause() function is a Matplotlib feature that introduces a delay in the execution, providing a visual representation of the plot to the user. This method is suitable for real-time plotting scenarios.
import matplotlib.pyplot as plt
import numpy as np
data = np.random.rand(10)
for value in data:
plt.plot(value)
plt.pause(0.5)
Common Errors and How to Handle Them
Error 1: Plot Not Updating
Solution: Check the Matplotlib backend and ensure that the plt.pause() function is appropriately placed within the loop.
Error 2: Unresponsive User Input
Solution: Verify that the input() function is correctly implemented and that there are no blocking issues in the loop.
Conclusion
In this post, we’ve learned how to pause a for loop and wait for user input in Matplotlib. This can be a powerful tool for creating interactive visualizations and can help you better understand your data.
Remember, the key to mastering any programming concept is practice. So, try integrating these techniques into your own projects and see what you can create!
References
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.