How to Pass Variables to spark.sql Query in PySpark: A Guide
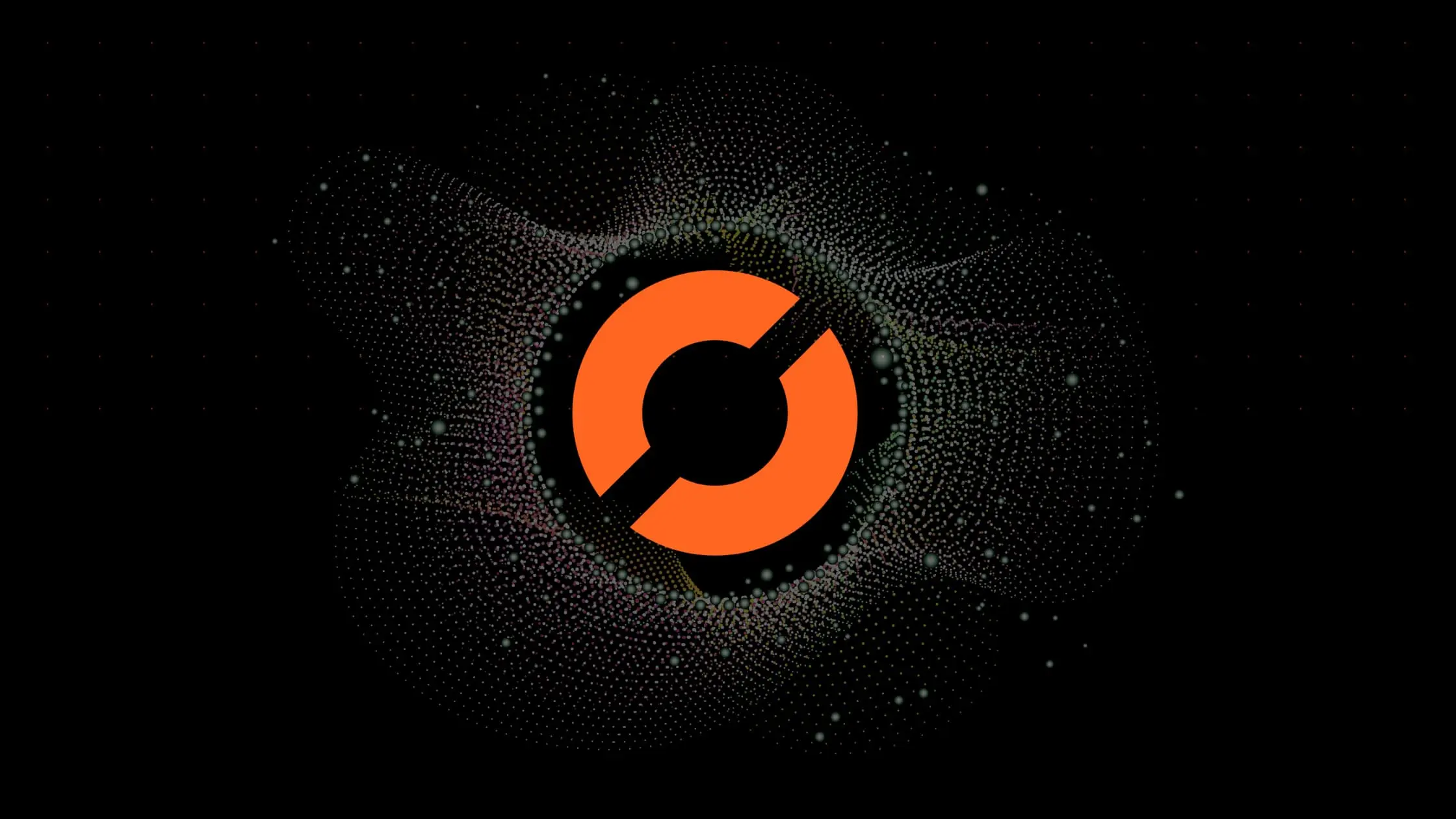
How to Pass Variables to spark.sql Query in PySpark: A Guide
In the world of big data, Apache Spark has emerged as a powerful computational engine that allows data scientists to process and analyze large datasets. PySpark, the Python library for Spark, is often used due to its simplicity and the wide range of Python libraries available. One common task when working with PySpark is passing variables to a spark.sql
query. This blog post will guide you through the process, step by step.
Why Pass Variables to spark.sql Query?
Before we delve into the how, let’s discuss the why. Passing variables to a spark.sql
query allows you to create dynamic queries. This is particularly useful when you need to filter data based on user input or a specific condition. By passing variables, you can write more flexible and reusable code.
Step 1: Initialize SparkSession
First, we need to initialize a SparkSession
. This is the entry point to any Spark functionality. If you’re working with Spark 2.0 and above, you only need a SparkSession
. Here’s how to initialize it:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("Pass Variables to spark.sql Query").getOrCreate()
Step 2: Create a DataFrame
Next, let’s create a DataFrame to work with. For this example, we’ll create a simple DataFrame with two columns: id
and value
.
data = [("1", "foo"), ("2", "bar"), ("3", "baz")]
df = spark.createDataFrame(data, ["id", "value"])
df.show()
Output:
+---+-----+
| id|value|
+---+-----+
| 1| foo|
| 2| bar|
| 3| baz|
+---+-----+
Step 3: Register the DataFrame as a TempTable
To use Spark SQL’s query capabilities, we need to register our DataFrame as a temporary table.
df.createOrReplaceTempView("tempTable")
Step 4: Pass Variables to spark.sql Query
Now, we’re ready to pass variables to our spark.sql
query. There are two common ways to do this: string formatting and concatenation.
Method 1: String Formatting
Python’s string formatting is a powerful tool that can be used to insert variables into your query. Here’s an example:
id_var = "1"
query = f"SELECT * FROM tempTable WHERE id = '{id_var}'"
result = spark.sql(query)
result.show()
Output:
+---+-----+
| id|value|
+---+-----+
| 1| foo|
+---+-----+
Method 2: String Concatenation
Alternatively, you can use string concatenation to build your query:
id_var = "1"
query = "SELECT * FROM tempTable WHERE id = '" + id_var + "'"
result = spark.sql(query)
result.show()
Output:
+---+-----+
| id|value|
+---+-----+
| 1| foo|
+---+-----+
Both methods will give you the same result. However, string formatting is generally preferred due to its readability and flexibility.
Important Note: Preventing SQL Injection
When passing variables to a spark.sql
query, it’s crucial to be aware of SQL injection, a common web hacking technique. Never pass user input directly into a query without sanitizing it first. Always validate and sanitize user input to prevent malicious SQL code from being executed.
Conclusion
Passing variables to a spark.sql
query in PySpark is a simple yet powerful technique that allows you to create dynamic queries. By following the steps outlined in this guide, you can write more flexible and reusable code.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.