How to Pass Variables and Data from PHP to JavaScript
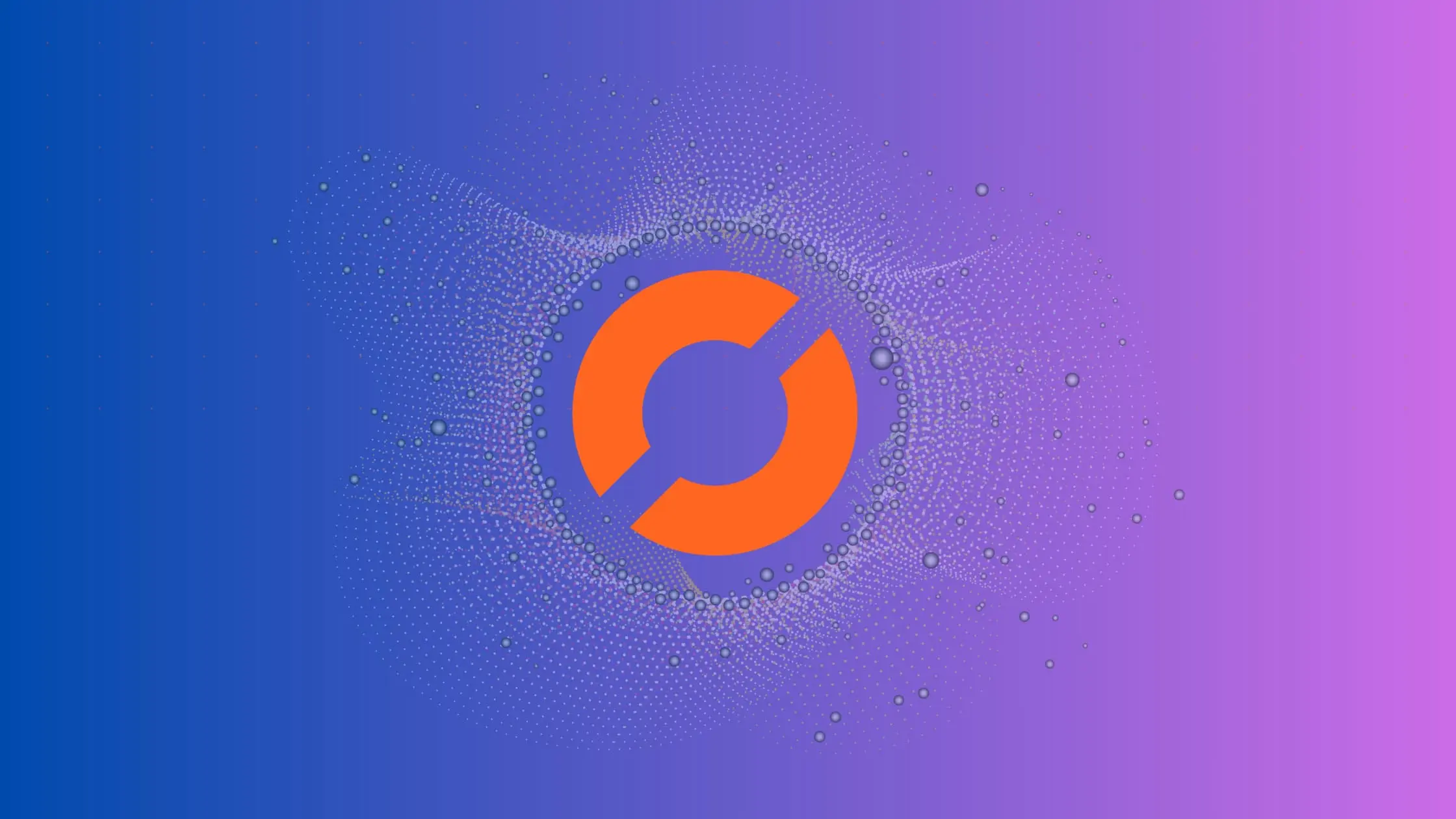
How to Pass Variables and Data from PHP to JavaScript
As a software engineer, you may find yourself working on a project that requires you to pass data from PHP to JavaScript. This task may seem daunting, but it’s actually quite simple once you understand the basics. In this post, we will cover the different methods you can use to pass variables and data from PHP to JavaScript.
Method 1: Using Inline JavaScript
The simplest way to pass data from PHP to JavaScript is by using inline JavaScript. In this method, you can echo out the data you want to pass from PHP using the echo
statement, and then assign it to a JavaScript variable.
Here’s an example:
<?php
$name = "John";
echo "<script>var name = '$name';</script>";
?>
In this example, we’re assigning the value of the PHP variable $name
to a JavaScript variable named name
. We’re doing this by echoing out a string of JavaScript code that sets the value of name
to the value of $name
.
This method is straightforward and easy to implement, but it has some downsides. For one, it can make your code harder to read and maintain, especially if you have a lot of inline JavaScript. It can also be a security risk if you’re not careful, as it can allow for cross-site scripting (XSS) attacks if you’re not sanitizing your input.
Method 2: Using JSON
Another way to pass data from PHP to JavaScript is by using JSON (JavaScript Object Notation). JSON is a lightweight data interchange format that is easy to read and write.
To use JSON, you first need to encode the data you want to pass from PHP as a JSON string using the json_encode
function. Once you have the JSON string, you can then pass it to JavaScript using the json_decode
function.
Here’s an example:
<?php
$data = array(
'name' => 'John',
'age' => 30,
'city' => 'New York'
);
$json = json_encode($data);
echo "<script>var data = $json;</script>";
?>
In this example, we’re creating an array called $data
that contains some data we want to pass to JavaScript. We’re then using the json_encode
function to encode the $data
array as a JSON string, which we’re assigning to the $json
variable. Finally, we’re echoing out a string of JavaScript code that sets the value of a JavaScript variable named data
to the decoded JSON string.
This method is more secure than using inline JavaScript, as it doesn’t allow for XSS attacks. It’s also more maintainable, as you can easily add or remove data from the $data
array without having to modify the JavaScript code.
Method 3: Using AJAX
If you’re working on a web application that requires dynamic updates without refreshing the page, you can use AJAX (Asynchronous JavaScript and XML) to pass data from PHP to JavaScript.
To use AJAX, you first need to create a PHP script that will return the data you want to pass to JavaScript. You can then use JavaScript to make an AJAX call to the PHP script and retrieve the data.
Here’s an example:
<?php
$data = array(
'name' => 'John',
'age' => 30,
'city' => 'New York'
);
echo json_encode($data);
?>
In this example, we’re creating the same $data
array as before, but instead of echoing out a JavaScript variable, we’re using the json_encode
function to encode the $data
array as a JSON string and directly outputting it.
To retrieve this data in JavaScript, you can use the XMLHttpRequest
object or a JavaScript library like jQuery to make an AJAX call to the PHP script and retrieve the data.
Here’s an example using jQuery:
$.ajax({
url: 'data.php',
success: function(data) {
var name = data.name;
var age = data.age;
var city = data.city;
console.log(name, age, city);
}
});
In this example, we’re using the $.ajax
function to make an AJAX call to the data.php
script. When the call is successful, the success
function is executed, which retrieves the data using the data
parameter and assigns it to JavaScript variables.
This method is the most powerful and flexible option, as it allows for dynamic updates and can retrieve data from external sources. However, it requires more setup and knowledge of AJAX and JavaScript.
PHP to JavaScript Data Scenarios
Dynamic Web Pages: When you want to create dynamic web pages where data from PHP needs to be displayed or manipulated in real-time on the client side using JavaScript. This can include scenarios like showing live updates, charts, or user-specific data.
Form Processing: When you have a form on a webpage, and after the user submits the form, you want to use JavaScript to display a success message or handle errors without reloading the entire page. This requires transferring data from PHP (usually after form submission) to JavaScript to update the user interface.
AJAX-Driven Applications: If you’re building a web application that relies heavily on AJAX to fetch or update data without reloading the entire page, you need to pass data between PHP and JavaScript. AJAX is commonly used in scenarios like auto-suggest search, infinite scrolling, or chat applications.
Security and Data Validation: When you want to pass data to JavaScript securely, you should use techniques like JSON encoding. This is particularly important in scenarios where you need to display user-specific data or content fetched from a database without exposing it to potential security vulnerabilities like Cross-Site Scripting (XSS) attacks.
Complex Web Applications: In more complex web applications, especially those built with JavaScript frameworks like React or Angular, you may need to pass data between PHP and JavaScript for various purposes, such as pre-rendering initial page content, handling application state, or updating the user interface based on user interactions.
Conclusion
Passing variables and data from PHP to JavaScript may seem like a daunting task, but it’s actually quite simple once you understand the basics. In this post, we covered three methods you can use to pass data from PHP to JavaScript: using inline JavaScript, using JSON, and using AJAX.
Each method has its own benefits and drawbacks, so you should choose the one that best fits your project’s needs. Whether you’re working on a simple web page or a complex web application, understanding how to pass data between PHP and JavaScript is an essential skill for any software engineer.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.