How to Parse Dates in Different Columns with Pandas' read_csv()
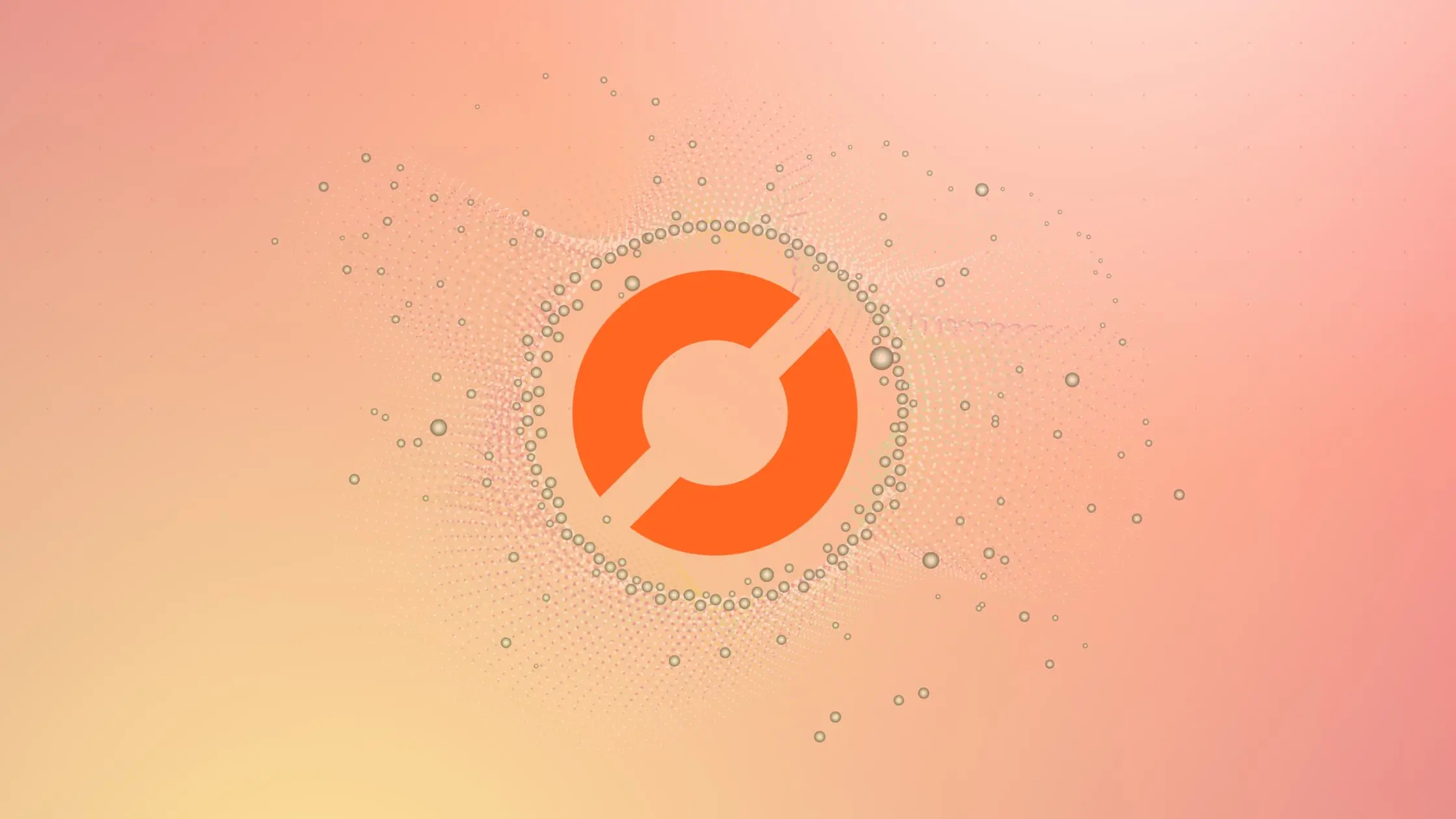
As a data scientist or software engineer, working with large datasets is a common task. One of the most popular Python libraries for handling data is Pandas. Pandas provides numerous functionalities for reading, manipulating, and storing data in various formats. In this article, we’ll explore how to parse dates in different columns with Pandas' read_csv()
function.
Background
Pandas' read_csv()
function is a versatile tool for reading data from CSV files. It can handle various types of data, including text, numbers, dates, and times. One of the most significant advantages of read_csv()
is its ability to parse dates automatically. However, by default, it tries to parse dates in a single column, which may not always be the case.
Consider the following CSV file:
date,sales
2021-01-01,100
2021-01-02,200
2021-01-03,300
If we use read_csv()
to read this file, it will correctly parse the date column as a datetime object. However, what if our data is structured differently, like this:
year,month,day,sales
2021,01,01,100
2021,01,02,200
2021,01,03,300
In this case, the date is split into three columns: year, month, and day. Parsing dates in this format requires some additional configuration.
Solution
To parse dates in different columns with read_csv()
, we need to use the parse_dates
parameter. By default, parse_dates
is set to False
, which means that read_csv()
will not attempt to parse any dates. If we set parse_dates
to True
, it will try to parse dates in all columns.
However, since we want to parse dates in specific columns, we need to provide a list of column names or column indices to parse_dates
. For example, to parse dates in the “year”, “month”, and “day” columns, we can use the following code:
import pandas as pd
df = pd.read_csv("data.csv", parse_dates={"date": ["year", "month", "day"]})
Here, we’re passing a dictionary to the parse_dates
parameter. The keys of the dictionary are the names of the columns that we want to parse as dates, and the values are lists of column names or indices that correspond to the parts of the date. In this case, we’re telling Pandas to parse the “year”, “month”, and “day” columns as a single date column called “date”.
We can also use column indices instead of column names:
df = pd.read_csv("data.csv", parse_dates={0: [1, 2, 3]})
In this example, we’re telling Pandas to parse the first column (index 0) as a date column, using the second, third, and fourth columns (indices 1, 2, and 3) as the date parts.
Conclusion
In conclusion, Pandas' read_csv()
function is a powerful tool for reading and parsing data from CSV files. When working with date data that is split into multiple columns, we can use the parse_dates
parameter to parse dates correctly. By providing a dictionary or a list of dictionaries to parse_dates
, we can specify which columns to parse and how to parse them.
I hope you found this article useful. Happy coding!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.