How to Name Only One Column in a Pandas DataFrame
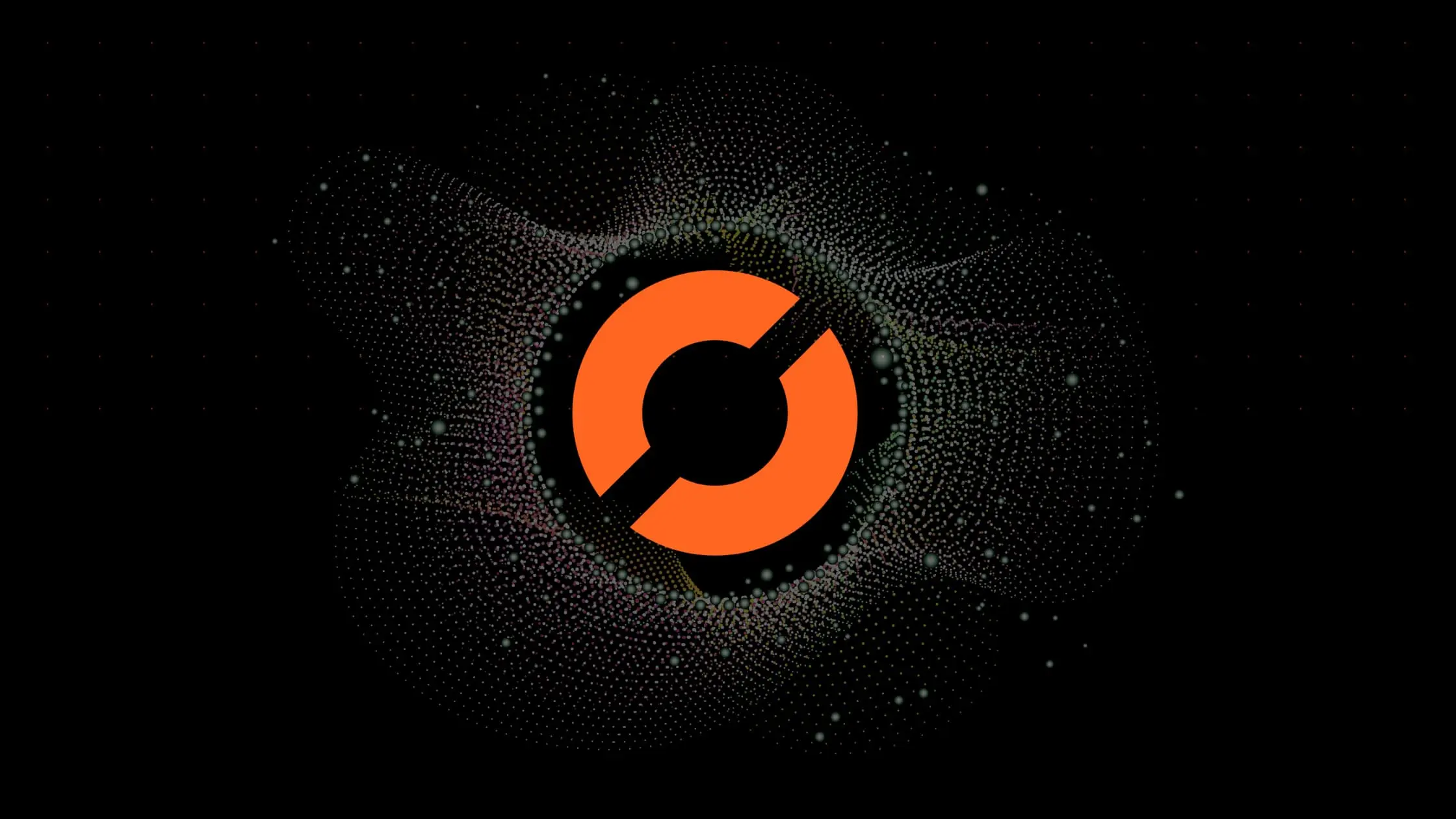
As a data scientist or software engineer working with data, you have likely encountered the need to rename columns in a Pandas DataFrame. Renaming a single column can be useful in situations where you only want to change the name of one variable or if you want to make the column name more descriptive. In this blog post, we will explore how to name only one column in a Pandas DataFrame, providing step-by-step instructions and code examples.
What is a Pandas DataFrame?
Before we dive into renaming columns, let’s first define what a Pandas DataFrame is. A DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table and is a fundamental data structure in the Pandas library. DataFrames are useful for data wrangling and manipulation, and they can handle a variety of data types, including numerical, categorical, and text data.
Renaming a Single Column in a Pandas DataFrame
To rename a single column in a Pandas DataFrame, we can use the rename()
method. This method can be used to rename one or more columns by passing a dictionary of the form {old_name: new_name}
to the columns
parameter. Here’s an example:
import pandas as pd
# create a sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
# rename column 'A' to 'X'
df = df.rename(columns={'A': 'X'})
# view the updated DataFrame
print(df)
Output:
X B
0 1 4
1 2 5
2 3 6
In this example, we first create a sample DataFrame with two columns, ‘A’ and ‘B’. We then use the rename()
method to rename column ‘A’ to ‘X’. Finally, we print the updated DataFrame, which now has columns ‘X’ and ‘B’.
Updating a Single Column Name in Place
In some cases, you may want to update the name of a single column in place, without creating a new DataFrame. To do this, we can simply assign a new name to the column using the df.columns
attribute. Here’s an example:
import pandas as pd
# create a sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
# update column name 'A' to 'X'
df.columns = ['X', 'B']
# view the updated DataFrame
print(df)
Output:
X B
0 1 4
1 2 5
2 3 6
In this example, we first create a sample DataFrame with two columns, ‘A’ and ‘B’. We then update the name of column ‘A’ to ‘X’ using the df.columns
attribute. Finally, we print the updated DataFrame, which now has columns ‘X’ and ‘B’.
Conclusion
In this blog post, we explored how to name only one column in a Pandas DataFrame. We learned that we can use the rename()
method to rename one or more columns by passing a dictionary of the form {old_name: new_name}
to the columns
parameter. We also learned that we can update a single column name in place by assigning a new name to the column using the df.columns
attribute.
Renaming a single column in a Pandas DataFrame can be a useful technique for data wrangling and manipulation, and it is a skill that every data scientist and software engineer should have in their toolkit. With the step-by-step instructions and code examples provided in this blog post, you should now be able to confidently rename a single column in a Pandas DataFrame.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.