How to Merge Pandas DataFrames with Different Column Names and Avoid Duplicates
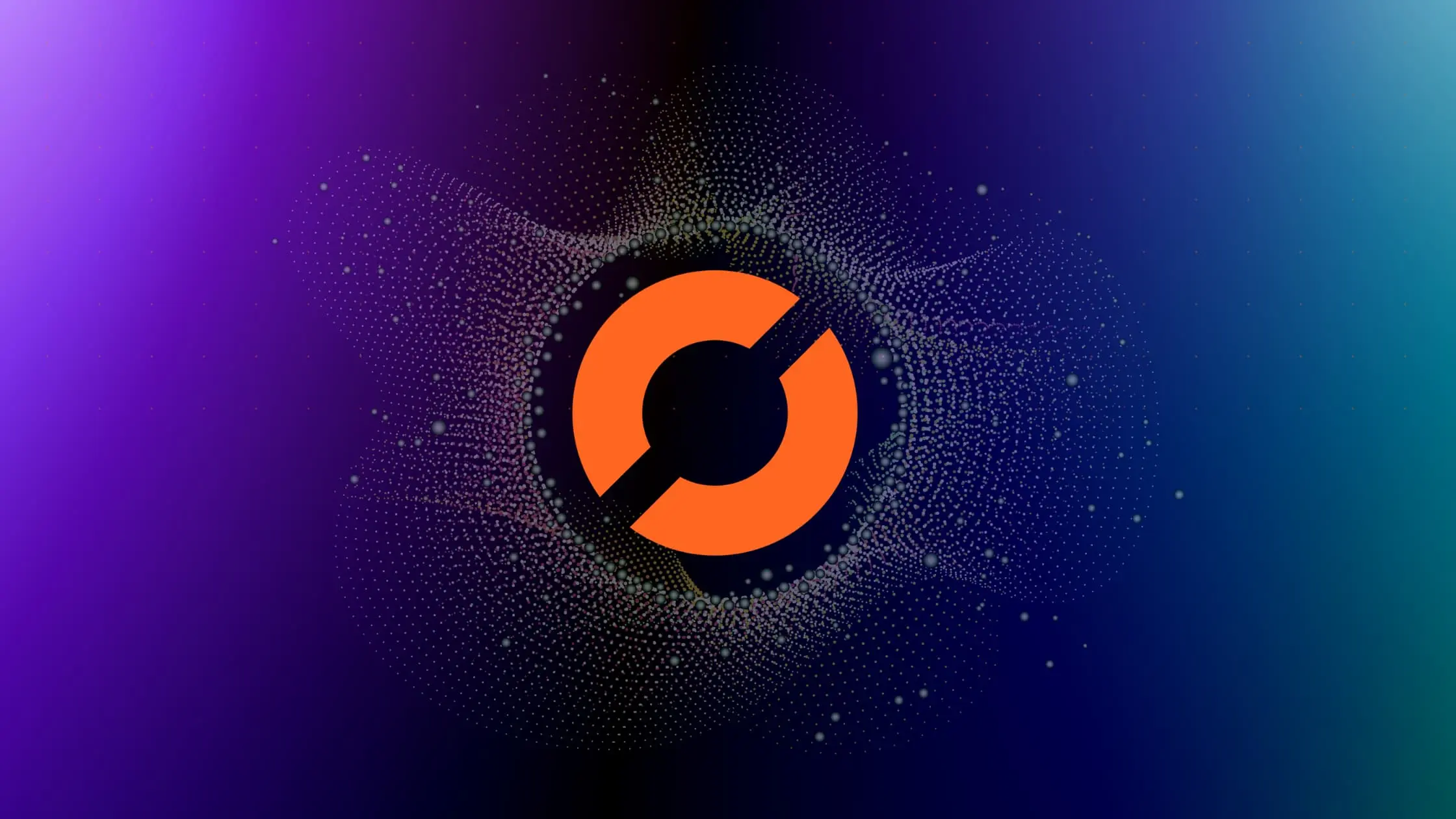
As a data scientist or software engineer, one of the most common tasks you’ll encounter is merging data from multiple sources. Pandas is a powerful tool for data manipulation and analysis, but merging DataFrames can be tricky, especially when the columns have different names. In this article, we will explore how to merge Pandas DataFrames with different column names and avoid duplicates.
Table of Contents
- What is Pandas Merge?
- Merging DataFrames with Different Column Names
- Avoiding Duplicates in Merged DataFrames
- Common Errors and Solutions
- Conclusion
What is Pandas Merge?
Pandas merge is a function that combines two or more DataFrames into a single DataFrame. The merge function compares the values in the specified columns of the two DataFrames and returns a new DataFrame with the matched values. The resulting DataFrame has all the columns from both DataFrames.
Merging DataFrames with Different Column Names
When merging DataFrames, it’s essential to ensure that the columns used for merging have the same name in both DataFrames. However, in some cases, the columns may have different names. In such cases, we can use the left_on
and right_on
parameters to specify the columns' names in each DataFrame.
Suppose we have two DataFrames, df1
and df2
, with columns id
and name
in df1
and columns customer_id
and amount
in df2
.
import pandas as pd
# Sample DataFrames
data1 = {'id': [1, 2, 3], 'name': ['Alice', 'Bob', 'Charlie']}
data2 = {'customer_id': [2, 3, 4], 'amount': [50, 30, 20]}
df1 = pd.DataFrame(data1)
df2 = pd.DataFrame(data2)
To merge these DataFrames, we can use the following code:
merged_df = pd.merge(df1, df2, left_on='id', right_on='customer_id', how='inner')
print(merged_df)
Output:
id name customer_id amount
0 2 Bob 2 50
1 3 Charlie 3 30
In the above code, we use the left_on
parameter to specify the column name in df1
, and the right_on
parameter to specify the column name in df2
. The resulting merged_df
DataFrame will have columns id
, name
, customer_id
, and amount
.
Avoiding Duplicates in Merged DataFrames
One common issue when merging DataFrames is the presence of duplicate values. Duplicate values can skew the results and lead to inaccurate analysis. To avoid duplicates, we can use the drop_duplicates
function to drop any duplicate rows in the merged DataFrame.
merged_df.drop_duplicates(subset=['id'], keep='first', inplace=True)
In the above code, we use the subset
parameter to specify the column(s) to consider when dropping duplicates. In this case, we use the id
column. The keep
parameter specifies which duplicate(s) to keep. In this case, we keep the first occurrence of each duplicate value. The inplace
parameter specifies whether to modify the original DataFrame or return a new DataFrame.
Common Errors and Solutions
Error: Column name not found.
Solution: Double-check column names and ensure they exist in both DataFrames.
Error: Unexpected duplicate columns.
Solution: Use the suffixes parameter to append custom suffixes and disambiguate the columns.
Conclusion
Merging Pandas DataFrames with different column names is a common task in data analysis and manipulation. In this article, we have explored how to merge DataFrames with different column names and avoid duplicates. By using the left_on
and right_on
parameters, we can merge DataFrames with different column names. Additionally, by using the drop_duplicates
function, we can avoid duplicates in the merged DataFrame.
Pandas is a powerful tool for data manipulation and analysis, and mastering its merge function is essential for any data scientist or software engineer. With the tips and techniques outlined in this article, you should be able to merge DataFrames with different column names and avoid duplicates with ease.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.