How to Merge Multiple Sheets from Multiple Excel Workbooks into a Single Pandas Dataframe
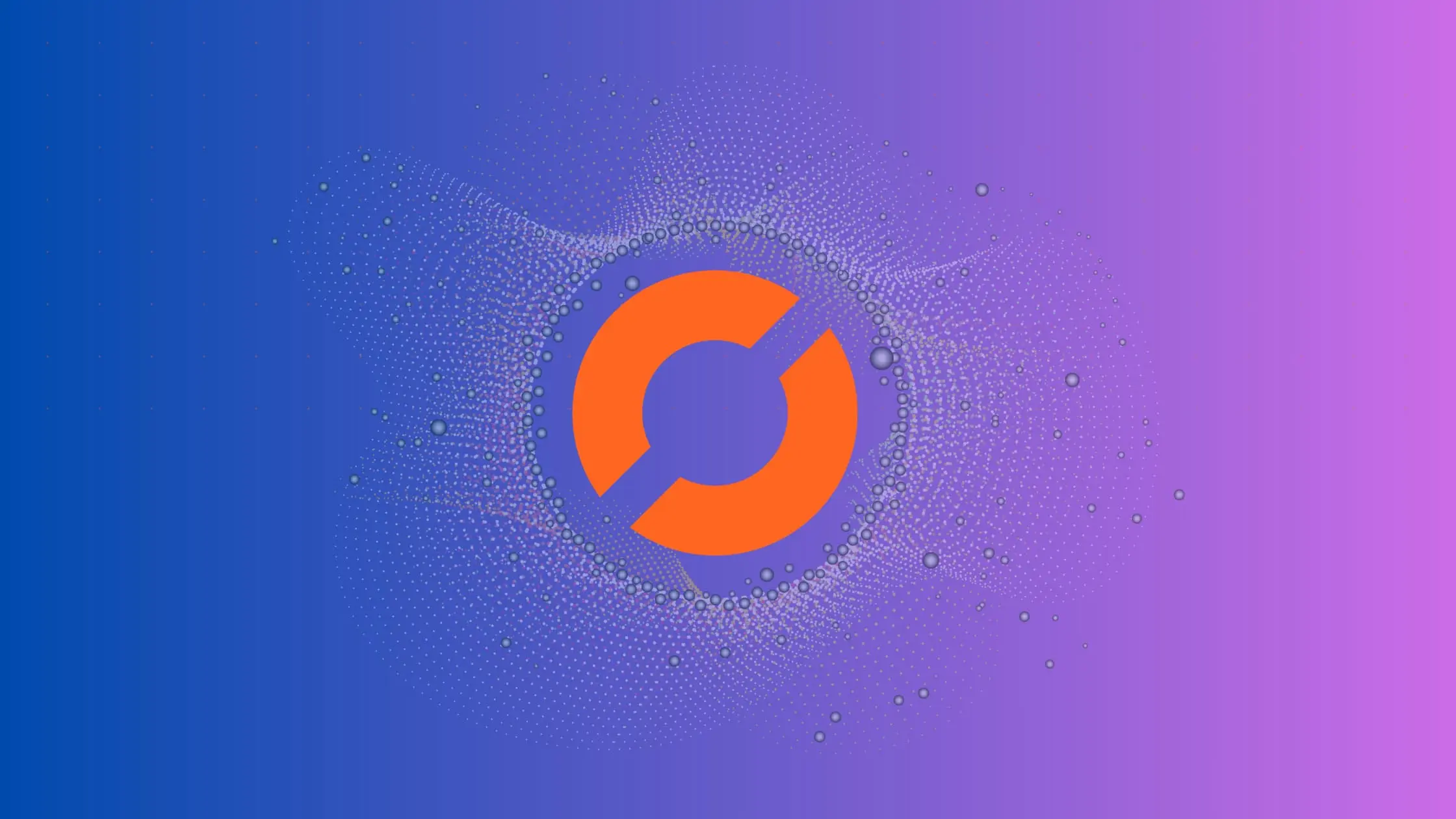
As a data scientist or software engineer, you may often find yourself working with data spread across different Excel workbooks and sheets. Manually combining these sheets can be a tedious and time-consuming task, especially when dealing with large amounts of data. Luckily, with the help of Python and Pandas, merging multiple sheets from multiple Excel workbooks into a single Pandas dataframe can be done quickly and easily.
In this article, we’ll go over the steps to merge multiple sheets from multiple Excel workbooks into a single Pandas dataframe. We’ll use Python and the Pandas library to achieve this. Let’s get started!
Table of Contents
- Introduction
- Step 1: Import Required Libraries
- Step 2: Get the List of Excel Files
- Step 3: Load the Data into Pandas Dataframes
- Step 4: Merge the Dataframes
- Step 5: Export the Merged Dataframe
- Pros and Cons of Using Python and Pandas for Merging Excel Sheets
- Error Handling in Merging Excel Sheets
- Conclusion
Step 1: Import Required Libraries
Before we begin, we need to import the required libraries. We’ll be using the Pandas and os libraries for this task. Here’s the code to import these libraries:
import pandas as pd
import os
Step 2: Get the List of Excel Files
Once we have imported the required libraries, the next step is to get the list of Excel files that we want to merge. We can do this using the os library. We’ll define a variable folder_path
that contains the path to the folder where our Excel files are located. We’ll then use the os.listdir()
method to get a list of all the files in that folder. We’ll then filter the list to only include Excel files using a list comprehension.
folder_path = '/path/to/folder'
files = [f for f in os.listdir(folder_path) if f.endswith('.xlsx')]
Excel sample data:
Step 3: Load the Data into Pandas Dataframes
Now that we have the list of Excel files, we can load the data from each file into a separate Pandas dataframe. We’ll use a for loop to iterate over the list of files and load the data from each file into a separate dataframe using the pd.read_excel()
method.
dataframes = []
for file in files:
df = pd.read_excel(os.path.join(folder_path, file))
dataframes.append(df)
Step 4: Merge the Dataframes
Now that we have loaded the data from each Excel file into a separate Pandas dataframe, we need to merge these dataframes into a single dataframe. We can do this using the pd.concat()
method. We’ll pass the list of dataframes to this method to merge them into a single dataframe.
merged_df = pd.concat(dataframes)
Step 5: Export the Merged Dataframe
Finally, we need to export the merged dataframe to a new Excel file. We can do this using the pd.to_excel()
method. We’ll define a variable output_path
that contains the path to the folder where we want to save the merged Excel file. We’ll then use the pd.to_excel()
method to export the merged dataframe to a new Excel file.
output_path = '/path/to/output/folder'
merged_df.to_excel(os.path.join(output_path, 'merged_data.xlsx'), index=False)
And that’s it! We have successfully merged multiple sheets from multiple Excel workbooks into a single Pandas dataframe using Python and Pandas.
Pros and Cons of Using Python and Pandas for Merging Excel Sheets
Pros
Automation and Efficiency: Automating the process with Python and Pandas saves significant time, especially when dealing with large datasets.
Scalability: This method can handle large amounts of data and multiple files with ease, which is not as practical with manual methods.
Flexibility: Python and Pandas offer the flexibility to handle various data types and structures within Excel files.
Data Integrity: Automated merging reduces the risk of human error, ensuring better data integrity.
Easy Integration: The merged data in Pandas can be easily used for further data processing, analysis, or visualization within the Python ecosystem.
Cons
Learning Curve: For those unfamiliar with Python or Pandas, there’s a learning curve involved.
Setup Requirements: This method requires the setup of a Python environment and installation of Pandas, which may not be straightforward for all users.
File Compatibility: Python and Pandas might face difficulties with very complex Excel files, particularly those containing macros or advanced formatting.
Resource Intensive: Large data processing might be resource-intensive, requiring a good hardware setup for smooth execution.
Error Handling in Merging Excel Sheets
When merging Excel sheets using Python and Pandas, you might encounter several errors. Here are common issues and how to handle them:
File Not Found Error: Ensure the file paths are correct. Use try-except blocks to catch and report incorrect file paths.
Corrupted or Unreadable Excel Files: Validate the integrity of Excel files before processing. Catch exceptions that indicate file corruption.
Mismatched Data Formats: Ensure all data frames have compatible formats before merging. Handle exceptions related to data type mismatches.
Memory Errors with Large Data: Implement chunk-based processing for large files to avoid overwhelming system memory.
Missing Data: Handle missing data in Excel files appropriately, either by filling in default values or by using Pandas functionality to handle NaN values.
Conclusion
In this article, we learned how to merge multiple sheets from multiple Excel workbooks into a single Pandas dataframe using Python and Pandas. We used the os library to get a list of Excel files, loaded the data from each file into a separate Pandas dataframe, merged the dataframes into a single dataframe using the pd.concat()
method, and exported the merged dataframe to a new Excel file using the pd.to_excel()
method.
By using Python and Pandas to merge multiple sheets from multiple Excel workbooks, we save time and reduce errors that can occur when manually combining data. We hope this article has been helpful in demonstrating how to merge multiple sheets from multiple Excel workbooks into a single Pandas dataframe.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.