How to Merge Two Data Frames on Multiple Columns using Pandas
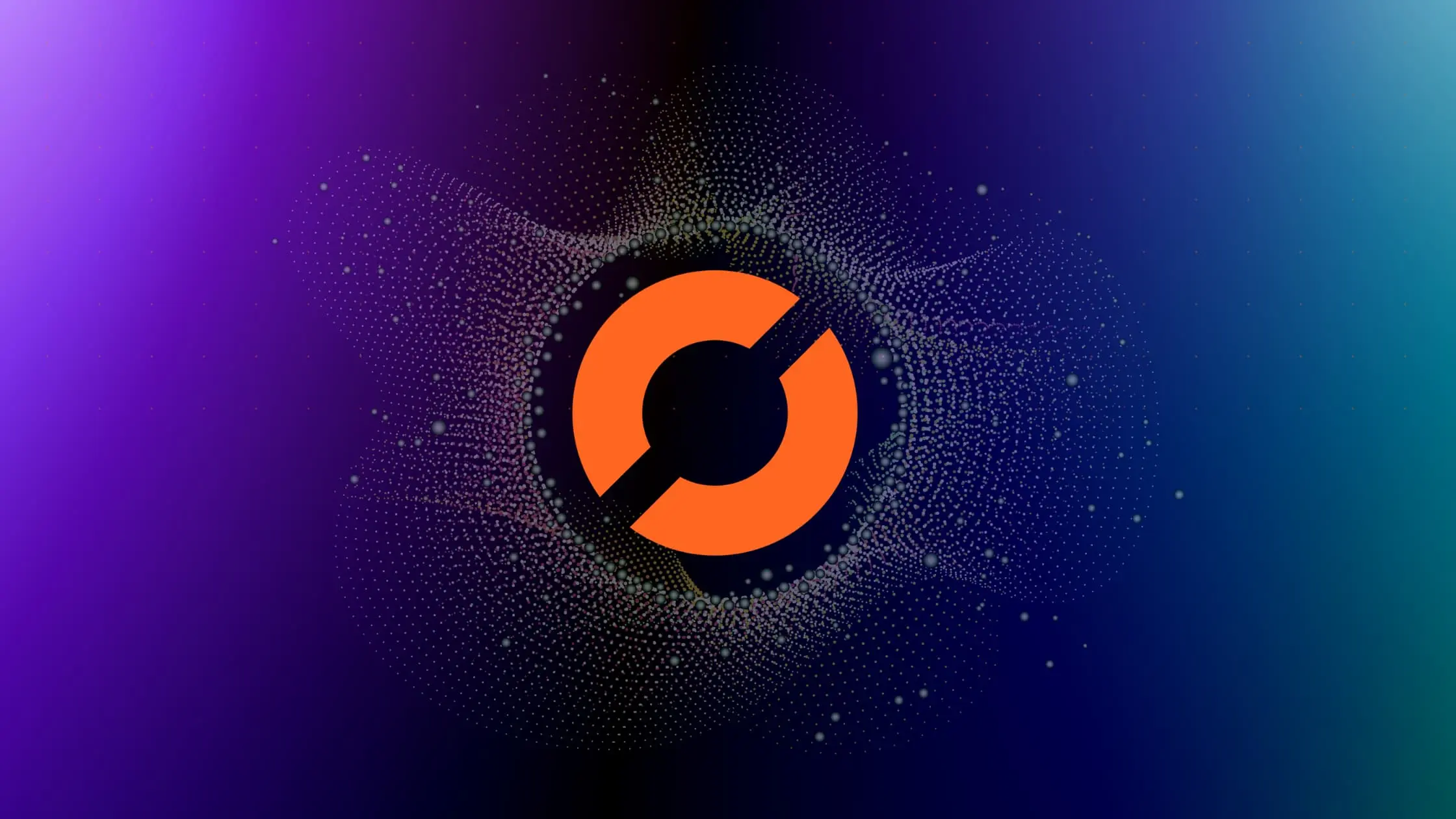
In this article, we will explore how to merge two data frames on multiple columns using Pandas.
Table of Contents
- Introduction to Pandas
- Why Merge Data Frames?
- How to Merge Data Frames on Multiple Columns?
- Common Errors
- Conclusion
Introduction to Pandas
Pandas is a widely used open-source data manipulation library for Python. It provides a fast and flexible way to work with structured data, including reading and writing data from various sources, cleaning, filtering, grouping, and transforming data, and merging or joining multiple data frames. Pandas is built on top of NumPy and provides easy-to-use data structures such as Series and DataFrame, which are optimized for data analysis.
Why Merge Data Frames?
Merging or joining data frames is a common task in data analysis and data science. It involves combining data from two or more data frames based on one or more common columns. This process allows you to combine data from different sources, compare and analyze data from multiple perspectives, and extract meaningful insights. For example, you may want to merge customer data with sales data to analyze customer behavior and preferences, or merge weather data with crop yield data to analyze the impact of weather on crop production.
How to Merge Data Frames on Multiple Columns?
Merging data frames in Pandas is a straightforward process. It involves specifying the common columns that you want to merge on and the type of merge operation that you want to perform. In this section, we will explore how to merge two data frames on multiple columns using Pandas step by step.
Step 1: Create Two Data Frames
Before we can merge two data frames, we need to create them. Let’s create two data frames that we will use for this example.
import pandas as pd
# Create first data frame
df1 = pd.DataFrame({
'key1': ['A', 'B', 'C', 'D'],
'key2': ['W', 'X', 'Y', 'Z'],
'value1': [1, 2, 3, 4],
'value2': [5, 6, 7, 8]
})
# Create second data frame
df2 = pd.DataFrame({
'key1': ['A', 'B', 'C', 'E'],
'key2': ['W', 'X', 'Z', 'Y'],
'value3': [9, 10, 11, 12],
'value4': [13, 14, 15, 16]
})
In this example, we created two data frames, df1
and df2
, with four columns each. The key1
and key2
columns are the common columns that we will use for merging the data frames.
Step 2: Merge Data Frames
Once we have created the data frames, we can merge them using the merge()
function in Pandas. The merge()
function takes two data frames as input and returns a new data frame that contains the merged data.
To merge the data frames on multiple columns, we need to specify the names of the common columns as a list in the on
parameter of the merge()
function. We can also specify the type of merge operation that we want to perform using the how
parameter. In this example, we will perform an inner join, which means that only the rows that have matching values in both data frames will be included in the merged data frame.
# Merge data frames on multiple columns
merged_df = pd.merge(df1, df2, on=['key1', 'key2'], how='inner')
After executing this code, we will have a new data frame called merged_df
that contains the merged data from df1
and df2
.
You can change the how
parameter to left
, right
, etc. and experiment different types of merges based on your dataframes.
Step 3: Explore the Merged Data Frame
Now that we have merged the data frames, we can explore the merged data frame to extract meaningful insights. We can use Pandas functions such as head()
and describe()
to view the first few rows and the summary statistics of the merged data frame.
# View first few rows of the merged data frame
print(merged_df.head())
# View summary statistics of the merged data frame
print(merged_df.describe())
This will output the first few rows and summary statistics of the merged data frame.
key1 key2 value1 value2 value3 value4
0 A W 1 5 9 13
1 B X 2 6 10 14
value1 value2 value3 value4
count 2.000000 2.000000 2.000000 2.000000
mean 1.500000 5.500000 9.500000 13.500000
std 0.707107 0.707107 0.707107 0.707107
min 1.000000 5.000000 9.000000 13.000000
25% 1.250000 5.250000 9.250000 13.250000
50% 1.500000 5.500000 9.500000 13.500000
75% 1.750000 5.750000 9.750000 13.750000
max 2.000000 6.000000 10.000000 14.000000
Common Errors
Mismatched column names: Ensure both dataframes have the exact same spelling and casing for the columns used in the on parameter. A single typo can lead to an empty or unexpected result.
Missing common columns: Attempting to merge on columns not present in both dataframes will lead to an error.
Data type inconsistencies: Merging on columns with incompatible data types (e.g., string vs. integer) can cause unexpected results or errors.
Conclusion
Merging or joining data frames is a common task in data analysis and data science. Pandas provides a powerful set of tools for merging data frames on multiple columns. In this article, we explored how to merge two data frames on multiple columns using Pandas step by step. We created two data frames, merged them on the common columns, and explored the merged data frame to extract meaningful insights. By mastering the merging capabilities of Pandas, you can unlock the full potential of your data and gain valuable insights that can help you make informed decisions.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.