How to lowercase a pandas dataframe string column if it has missing values
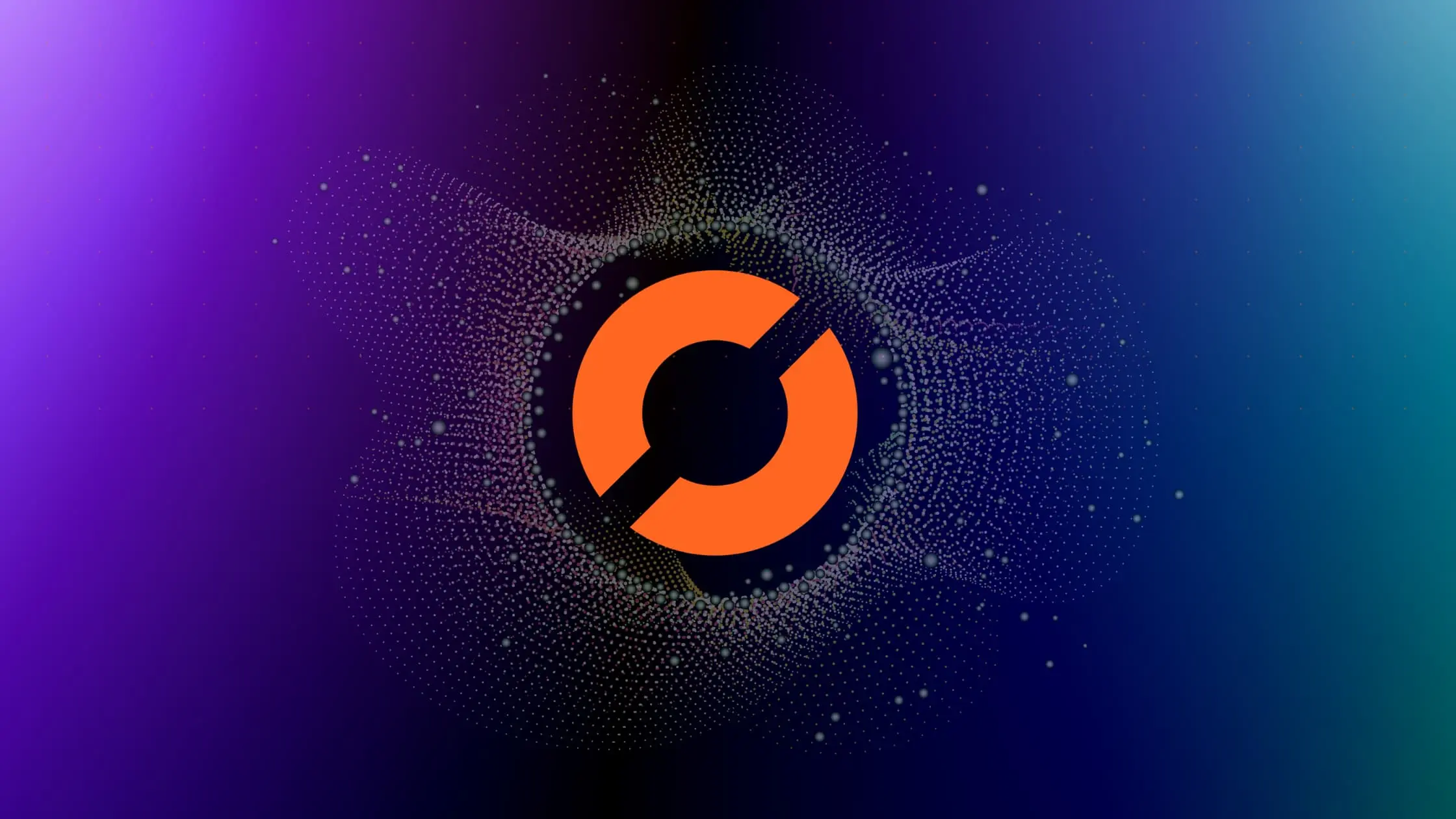
As a data scientist, one of the most common tasks you’ll encounter is data cleaning and preparation. This often involves dealing with missing values, which can be a challenge when you’re trying to lowercase a string column in a pandas dataframe. In this article, we’ll explore how to lowercase a pandas dataframe string column even if it has missing values.
Table of Contents
Background
Pandas is a popular Python library for data manipulation and analysis. It provides easy-to-use data structures and data analysis tools for handling structured data. One of the most useful features of pandas is its ability to handle missing data. In pandas, missing data is represented by NaN (Not a Number) values, which can be easily identified and handled.
When working with string columns in pandas dataframes, it’s often necessary to convert all the values to lowercase. This can be done using the str.lower() method, which converts all the characters in a string to lowercase. However, if the string column contains missing values, you need to handle them properly to avoid errors.
Solution
Using apply()
and lambda
To lowercase a pandas dataframe string column if it has missing values, you can use the apply()
method. The apply()
method applies a function along an axis of the dataframe. In this case, you can apply the str.lower()
method to the string column while handling missing values.
Here’s an example:
import pandas as pd
# create a sample dataframe with a string column containing missing values
data = {'name': ['Alice', 'Bob', 'Charlie', 'David', None],
'age': [25, 32, 18, 47, 22]}
df = pd.DataFrame(data)
# lowercase the string column while handling missing values
df['name'] = df['name'].apply(lambda x: x.lower() if isinstance(x, str) else x)
print(df)
Output:
name age
0 alice 25
1 bob 32
2 charlie 18
3 david 47
4 None 22
In this example, we first create a sample dataframe with a string column containing missing values. We then use the apply()
method to apply a lambda function to the ‘name’ column. The lambda function checks if the value is a string using the isinstance()
method. If it’s a string, it applies the str.lower()
method to lowercase the string. Otherwise, it returns the original value.
This approach handles missing values properly by leaving them as NaN. It also ensures that only string values are lowered, avoiding errors that could occur if you tried to apply the str.lower()
method to a non-string value.
Using str.lower()
Method
The str.lower()
method in pandas is a convenient way to transform the values of a string column to lowercase. It is applied to string-type elements in the column, converting them to lowercase while leaving non-string (e.g., numeric or missing) values unchanged. This method ensures that the string column becomes case-insensitive, facilitating consistent comparisons and analyses.
Let’s illustrate how to use the str.lower()
method with your example code:
import pandas as pd
# Create a sample dataframe with a string column containing missing values
data = {'name': ['Alice', 'Bob', 'Charlie', 'David', None],
'age': [25, 32, 18, 47, 22]}
df = pd.DataFrame(data)
# Use the str.lower() method to lowercase the 'name' column
df['name'] = df['name'].str.lower()
# Display the modified dataframe
print(df)
In this example, the str.lower()
method is applied to the ‘name’ column using the syntax df['name'].str.lower()
. This transforms the string values in the ‘name’ column to lowercase, while leaving the None value (representing missing data) unchanged. The resulting dataframe will have the ‘name’ column with lowercase string values:
name age
0 alice 25
1 bob 32
2 charlie 18
3 david 47
4 None 22
As seen in the output, the name
column now contains lowercase versions of the original string values, demonstrating the effective use of the str.lower()
method in pandas.
Conclusion
In this article, we’ve explored how to lowercase a pandas dataframe string column even if it has missing values. We’ve seen that you can use the apply()
method with a lambda function to handle missing values and apply the str.lower()
method only to string values. This approach ensures that missing values are properly handled and non-string values are not lowered, avoiding errors. You can also apply str.lower()
directly to the desired column, which yeilds the same output.
Data cleaning and preparation can be time-consuming, but with pandas, you have a powerful tool at your disposal. By leveraging its features, you can quickly and easily handle missing data and manipulate your data to suit your needs.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.